Note
Go to the end to download the full example code
Bootstrap and Closest Peak Direction Getters Example#
This example shows how choices in direction-getter impact fiber tracking results by demonstrating the bootstrap direction getter (a type of probabilistic tracking, as described in Berman et al. (2008) [Berman2008] a nd the closest peak direction getter (a type of deterministic tracking). (Amirbekian, PhD thesis, 2016)
This example is an extension of the Introduction to Basic Tracking example. Let’s start by loading the necessary modules for executing this tutorial.
from dipy.core.gradients import gradient_table
from dipy.data import get_fnames, small_sphere
from dipy.direction import BootDirectionGetter, ClosestPeakDirectionGetter
from dipy.io.gradients import read_bvals_bvecs
from dipy.io.image import load_nifti, load_nifti_data
from dipy.io.stateful_tractogram import Space, StatefulTractogram
from dipy.io.streamline import save_trk
from dipy.reconst.csdeconv import (ConstrainedSphericalDeconvModel,
auto_response_ssst)
from dipy.reconst.shm import CsaOdfModel
from dipy.tracking import utils
from dipy.tracking.local_tracking import LocalTracking
from dipy.tracking.streamline import Streamlines
from dipy.tracking.stopping_criterion import ThresholdStoppingCriterion
from dipy.viz import window, actor, colormap, has_fury
# Enables/disables interactive visualization
interactive = False
hardi_fname, hardi_bval_fname, hardi_bvec_fname = get_fnames('stanford_hardi')
label_fname = get_fnames('stanford_labels')
data, affine, hardi_img = load_nifti(hardi_fname, return_img=True)
labels = load_nifti_data(label_fname)
bvals, bvecs = read_bvals_bvecs(hardi_bval_fname, hardi_bvec_fname)
gtab = gradient_table(bvals, bvecs)
seed_mask = (labels == 2)
white_matter = (labels == 1) | (labels == 2)
seeds = utils.seeds_from_mask(seed_mask, affine, density=1)
Next, we fit the CSD model.
response, ratio = auto_response_ssst(gtab, data, roi_radii=10, fa_thr=0.7)
csd_model = ConstrainedSphericalDeconvModel(gtab, response, sh_order=6)
csd_fit = csd_model.fit(data, mask=white_matter)
0%| | 0/58788 [00:00<?, ?it/s]
1%|▋ | 360/58788 [00:00<00:16, 3581.96it/s]
1%|█▌ | 793/58788 [00:00<00:14, 3999.74it/s]
2%|██▍ | 1265/58788 [00:00<00:13, 4309.22it/s]
3%|███▌ | 1856/58788 [00:00<00:11, 4926.23it/s]
4%|████▍ | 2377/58788 [00:00<00:11, 5011.87it/s]
5%|█████▌ | 2948/58788 [00:00<00:10, 5229.11it/s]
6%|██████▌ | 3471/58788 [00:00<00:11, 4896.59it/s]
7%|███████▌ | 3975/58788 [00:00<00:11, 4929.99it/s]
8%|████████▍ | 4471/58788 [00:00<00:11, 4781.72it/s]
8%|█████████▎ | 4952/58788 [00:01<00:11, 4779.60it/s]
9%|██████████▎ | 5476/58788 [00:01<00:10, 4901.94it/s]
10%|███████████▎ | 5968/58788 [00:01<00:10, 4876.66it/s]
11%|████████████▎ | 6539/58788 [00:01<00:10, 5010.60it/s]
12%|█████████████▌ | 7164/58788 [00:01<00:09, 5361.91it/s]
14%|███████████████ | 7996/58788 [00:01<00:08, 6213.45it/s]
15%|████████████████▍ | 8736/58788 [00:01<00:07, 6544.12it/s]
16%|█████████████████▋ | 9393/58788 [00:01<00:07, 6313.69it/s]
17%|██████████████████▊ | 10044/58788 [00:01<00:07, 6353.90it/s]
18%|███████████████████▉ | 10682/58788 [00:01<00:07, 6345.20it/s]
19%|█████████████████████▏ | 11318/58788 [00:02<00:08, 5664.58it/s]
20%|██████████████████████▎ | 11899/58788 [00:02<00:08, 5692.60it/s]
21%|███████████████████████▎ | 12479/58788 [00:02<00:08, 5551.73it/s]
22%|████████████████████████▍ | 13042/58788 [00:02<00:08, 5564.15it/s]
23%|█████████████████████████▍ | 13604/58788 [00:02<00:08, 5262.55it/s]
24%|██████████████████████████▍ | 14137/58788 [00:02<00:08, 5128.06it/s]
25%|███████████████████████████▍ | 14654/58788 [00:02<00:09, 4880.61it/s]
26%|████████████████████████████▍ | 15188/58788 [00:02<00:08, 4993.49it/s]
27%|█████████████████████████████▎ | 15693/58788 [00:02<00:08, 4996.78it/s]
28%|██████████████████████████████▎ | 16196/58788 [00:03<00:08, 4964.09it/s]
29%|███████████████████████████████▍ | 16788/58788 [00:03<00:08, 5225.73it/s]
30%|████████████████████████████████▍ | 17369/58788 [00:03<00:07, 5380.45it/s]
31%|█████████████████████████████████▌ | 17951/58788 [00:03<00:07, 5497.26it/s]
31%|██████████████████████████████████▌ | 18503/58788 [00:03<00:07, 5335.39it/s]
32%|███████████████████████████████████▌ | 19039/58788 [00:03<00:07, 5327.60it/s]
33%|████████████████████████████████████▋ | 19574/58788 [00:03<00:07, 5132.74it/s]
34%|█████████████████████████████████████▊ | 20235/58788 [00:03<00:07, 5461.80it/s]
35%|██████████████████████████████████████▉ | 20790/58788 [00:03<00:06, 5472.15it/s]
36%|███████████████████████████████████████▉ | 21339/58788 [00:04<00:07, 5278.04it/s]
37%|████████████████████████████████████████▉ | 21869/58788 [00:04<00:07, 5127.49it/s]
38%|█████████████████████████████████████████▉ | 22384/58788 [00:04<00:07, 4905.90it/s]
39%|██████████████████████████████████████████▊ | 22902/58788 [00:04<00:07, 4930.92it/s]
40%|███████████████████████████████████████████▊ | 23420/58788 [00:04<00:07, 4990.50it/s]
41%|████████████████████████████████████████████▊ | 23921/58788 [00:04<00:07, 4688.32it/s]
42%|█████████████████████████████████████████████▊ | 24464/58788 [00:04<00:07, 4883.81it/s]
43%|██████████████████████████████████████████████▊ | 24986/58788 [00:04<00:06, 4966.44it/s]
43%|███████████████████████████████████████████████▊ | 25534/58788 [00:04<00:06, 5103.29it/s]
44%|████████████████████████████████████████████████▉ | 26138/58788 [00:04<00:06, 5362.27it/s]
45%|█████████████████████████████████████████████████▉ | 26677/58788 [00:05<00:05, 5357.34it/s]
46%|██████████████████████████████████████████████████▉ | 27215/58788 [00:05<00:06, 5196.56it/s]
47%|███████████████████████████████████████████████████▉ | 27737/58788 [00:05<00:06, 5072.26it/s]
48%|████████████████████████████████████████████████████▊ | 28247/58788 [00:05<00:06, 5069.55it/s]
49%|█████████████████████████████████████████████████████▊ | 28756/58788 [00:05<00:05, 5033.23it/s]
50%|██████████████████████████████████████████████████████▊ | 29299/58788 [00:05<00:05, 5133.67it/s]
51%|███████████████████████████████████████████████████████▊ | 29833/58788 [00:05<00:05, 5184.04it/s]
52%|████████████████████████████████████████████████████████▉ | 30400/58788 [00:05<00:05, 5316.10it/s]
53%|█████████████████████████████████████████████████████████▉ | 30933/58788 [00:05<00:05, 5013.15it/s]
54%|███████████████████████████████████████████████████████████ | 31564/58788 [00:06<00:05, 5371.67it/s]
55%|████████████████████████████████████████████████████████████ | 32123/58788 [00:06<00:04, 5420.65it/s]
56%|█████████████████████████████████████████████████████████████▏ | 32669/58788 [00:06<00:04, 5295.00it/s]
57%|██████████████████████████████████████████████████████████████▎ | 33288/58788 [00:06<00:04, 5540.94it/s]
58%|███████████████████████████████████████████████████████████████▎ | 33845/58788 [00:06<00:04, 5378.07it/s]
58%|████████████████████████████████████████████████████████████████▎ | 34386/58788 [00:06<00:04, 5342.69it/s]
59%|█████████████████████████████████████████████████████████████████▎ | 34922/58788 [00:06<00:04, 5332.26it/s]
60%|██████████████████████████████████████████████████████████████████▍ | 35477/58788 [00:06<00:04, 5382.11it/s]
61%|███████████████████████████████████████████████████████████████████▍ | 36026/58788 [00:06<00:04, 5400.70it/s]
62%|████████████████████████████████████████████████████████████████████▍ | 36567/58788 [00:06<00:04, 4976.06it/s]
63%|█████████████████████████████████████████████████████████████████████▌ | 37198/58788 [00:07<00:04, 5333.77it/s]
64%|██████████████████████████████████████████████████████████████████████▊ | 37835/58788 [00:07<00:03, 5613.33it/s]
65%|███████████████████████████████████████████████████████████████████████▊ | 38403/58788 [00:07<00:03, 5267.56it/s]
66%|████████████████████████████████████████████████████████████████████████▉ | 38960/58788 [00:07<00:03, 5238.11it/s]
67%|█████████████████████████████████████████████████████████████████████████▉ | 39490/58788 [00:07<00:03, 4936.58it/s]
68%|██████████████████████████████████████████████████████████████████████████▊ | 39990/58788 [00:07<00:03, 4941.99it/s]
69%|███████████████████████████████████████████████████████████████████████████▊ | 40489/58788 [00:07<00:03, 4939.58it/s]
70%|████████████████████████████████████████████████████████████████████████████▉ | 41090/58788 [00:07<00:03, 5129.39it/s]
71%|█████████████████████████████████████████████████████████████████████████████▉ | 41635/58788 [00:07<00:03, 5210.49it/s]
72%|██████████████████████████████████████████████████████████████████████████████▉ | 42211/58788 [00:08<00:03, 5356.94it/s]
73%|███████████████████████████████████████████████████████████████████████████████▉ | 42749/58788 [00:08<00:02, 5352.71it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▎ | 43473/58788 [00:08<00:02, 5891.01it/s]
75%|██████████████████████████████████████████████████████████████████████████████████▍ | 44064/58788 [00:08<00:02, 5369.92it/s]
76%|███████████████████████████████████████████████████████████████████████████████████▌ | 44678/58788 [00:08<00:02, 5573.49it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▋ | 45244/58788 [00:08<00:02, 5588.08it/s]
78%|█████████████████████████████████████████████████████████████████████████████████████▋ | 45809/58788 [00:08<00:02, 5288.67it/s]
79%|██████████████████████████████████████████████████████████████████████████████████████▊ | 46387/58788 [00:08<00:02, 5415.08it/s]
80%|███████████████████████████████████████████████████████████████████████████████████████▊ | 46935/58788 [00:08<00:02, 5302.17it/s]
81%|████████████████████████████████████████████████████████████████████████████████████████▊ | 47470/58788 [00:09<00:02, 5272.34it/s]
82%|█████████████████████████████████████████████████████████████████████████████████████████▊ | 48000/58788 [00:09<00:02, 5267.13it/s]
83%|██████████████████████████████████████████████████████████████████████████████████████████▊ | 48529/58788 [00:09<00:01, 5261.69it/s]
84%|███████████████████████████████████████████████████████████████████████████████████████████▉ | 49113/58788 [00:09<00:01, 5408.93it/s]
84%|████████████████████████████████████████████████████████████████████████████████████████████▉ | 49656/58788 [00:09<00:01, 5246.76it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▉ | 50217/58788 [00:09<00:01, 5338.33it/s]
86%|██████████████████████████████████████████████████████████████████████████████████████████████▉ | 50753/58788 [00:09<00:01, 5328.05it/s]
87%|███████████████████████████████████████████████████████████████████████████████████████████████▉ | 51287/58788 [00:09<00:01, 5170.56it/s]
88%|████████████████████████████████████████████████████████████████████████████████████████████████▉ | 51806/58788 [00:09<00:01, 5019.27it/s]
89%|█████████████████████████████████████████████████████████████████████████████████████████████████▉ | 52320/58788 [00:09<00:01, 5042.05it/s]
90%|██████████████████████████████████████████████████████████████████████████████████████████████████▊ | 52826/58788 [00:10<00:01, 4920.83it/s]
91%|███████████████████████████████████████████████████████████████████████████████████████████████████▊ | 53320/58788 [00:10<00:01, 4748.57it/s]
92%|████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 53842/58788 [00:10<00:01, 4872.65it/s]
93%|█████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 54430/58788 [00:10<00:00, 5153.57it/s]
94%|███████████████████████████████████████████████████████████████████████████████████████████████████████ | 55097/58788 [00:10<00:00, 5583.79it/s]
95%|████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 55690/58788 [00:10<00:00, 5674.04it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 56260/58788 [00:10<00:00, 5353.81it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 56813/58788 [00:10<00:00, 5391.10it/s]
98%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 57356/58788 [00:10<00:00, 5092.77it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 57890/58788 [00:11<00:00, 5149.83it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▎| 58428/58788 [00:11<00:00, 5204.55it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████| 58788/58788 [00:11<00:00, 5221.44it/s]
we use the CSA fit to calculate GFA, which will serve as our stopping criterion.
csa_model = CsaOdfModel(gtab, sh_order=6)
gfa = csa_model.fit(data, mask=white_matter).gfa
stopping_criterion = ThresholdStoppingCriterion(gfa, .25)
Next, we need to set up our two direction getters
Example #1: Bootstrap direction getter with CSD Model#
boot_dg_csd = BootDirectionGetter.from_data(data, csd_model, max_angle=30.,
sphere=small_sphere)
boot_streamline_generator = LocalTracking(boot_dg_csd, stopping_criterion,
seeds, affine, step_size=.5)
streamlines = Streamlines(boot_streamline_generator)
sft = StatefulTractogram(streamlines, hardi_img, Space.RASMM)
save_trk(sft, "tractogram_bootstrap_dg.trk")
if has_fury:
scene = window.Scene()
scene.add(actor.line(streamlines, colormap.line_colors(streamlines)))
window.record(scene, out_path='tractogram_bootstrap_dg.png',
size=(800, 800))
if interactive:
window.show(scene)
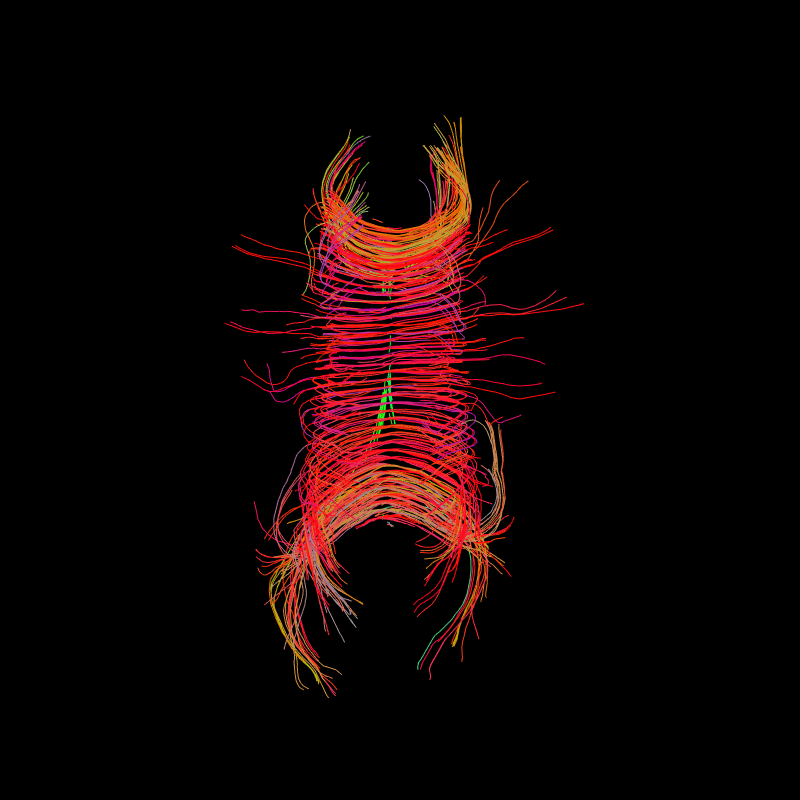
Corpus Callosum Bootstrap Probabilistic Direction Getter
We have created a bootstrapped probabilistic set of streamlines. If you repeat the fiber tracking (keeping all inputs the same) you will NOT get exactly the same set of streamlines.
Example #2: Closest peak direction getter with CSD Model#
pmf = csd_fit.odf(small_sphere).clip(min=0)
peak_dg = ClosestPeakDirectionGetter.from_pmf(pmf, max_angle=30.,
sphere=small_sphere)
peak_streamline_generator = LocalTracking(peak_dg, stopping_criterion, seeds,
affine, step_size=.5)
streamlines = Streamlines(peak_streamline_generator)
sft = StatefulTractogram(streamlines, hardi_img, Space.RASMM)
save_trk(sft, "closest_peak_dg_CSD.trk")
if has_fury:
scene = window.Scene()
scene.add(actor.line(streamlines, colormap.line_colors(streamlines)))
window.record(scene, out_path='tractogram_closest_peak_dg.png',
size=(800, 800))
if interactive:
window.show(scene)
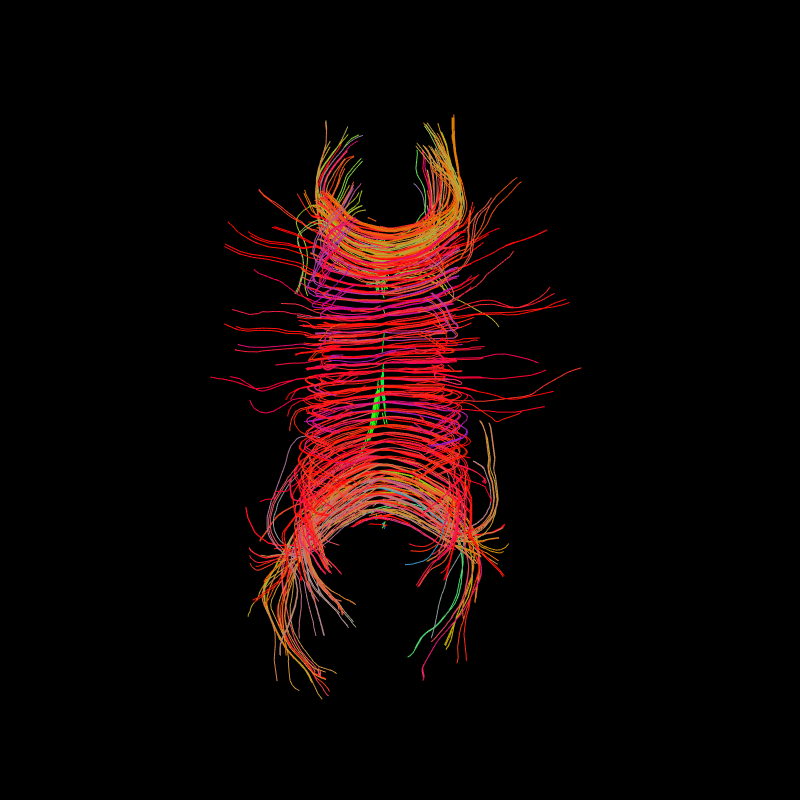
Corpus Callosum Closest Peak Deterministic Direction Getter
We have created a set of streamlines using the closest peak direction getter, which is a type of deterministic tracking. If you repeat the fiber tracking (keeping all inputs the same) you will get exactly the same set of streamlines.
References#
Berman, J. et al., Probabilistic streamline q-ball
tractography using the residual bootstrap, NeuroImage, vol 39, no 1, 2008
Total running time of the script: (0 minutes 43.549 seconds)