Note
Go to the end to download the full example code
Denoise images using the Marcenko-Pastur PCA algorithm#
The PCA-based denoising algorithm exploits the redundancy across the diffusion-weighted images [Manjon2013], [Veraart2016a]. This algorithm has been shown to provide an optimal compromise between noise suppression and loss of anatomical information for different techniques such as DTI [Manjon2013], spherical deconvolution [Veraart2016a] and DKI [Henri2018].
The basic idea behind the PCA-based denoising algorithms is to remove the components of the data that are classified as noise. The Principal Components classification can be performed based on prior noise variance estimates [Manjon2013] (see denoise_localpca) # noqa E501 or automatically based on the Marchenko-Pastur distribution [Veraa2016a]. In addition to noise suppression, the PCA algorithm can be used to get the standard deviation of the noise [Veraa2016b].
In the following example, we show how to denoise diffusion MRI images and estimate the noise standard deviation using the PCA algorithm based on the Marcenko-Pastur distribution [Veraa2016a]
Let’s load the necessary modules
# load general modules
import numpy as np
import matplotlib.pyplot as plt
from time import time
# load main pca function using Marcenko-Pastur distribution
from dipy.denoise.localpca import mppca
# load functions to fetch data for this example
from dipy.data import get_fnames
# load other dipy's functions that will be used for auxiliary analysis
from dipy.core.gradients import gradient_table
from dipy.io.image import load_nifti, save_nifti
from dipy.io.gradients import read_bvals_bvecs
from dipy.segment.mask import median_otsu
import dipy.reconst.dki as dki
For this example, we use fetch to download a multi-shell dataset which was kindly provided by Hansen and Jespersen (more details about the data are provided in their paper [Hansen2016]). The total size of the downloaded data is 192 MBytes, however you only need to fetch it once.
dwi_fname, dwi_bval_fname, dwi_bvec_fname, _ = get_fnames('cfin_multib')
data, affine = load_nifti(dwi_fname)
bvals, bvecs = read_bvals_bvecs(dwi_bval_fname, dwi_bvec_fname)
gtab = gradient_table(bvals, bvecs)
For the sake of simplicity, we only select two non-zero b-values for this example.
bvals = gtab.bvals
bvecs = gtab.bvecs
sel_b = np.logical_or(np.logical_or(bvals == 0, bvals == 1000), bvals == 2000)
data = data[..., sel_b]
gtab = gradient_table(bvals[sel_b], bvecs[sel_b])
print(data.shape)
(96, 96, 19, 67)
As one can see from its shape, the selected data contains a total of 67 volumes of images corresponding to all the diffusion gradient directions of the selected b-values.
The PCA denoising using the Marchenko-Pastur distribution can be performed by
calling the function mppca
:
t = time()
denoised_arr = mppca(data, patch_radius=2)
print("Time taken for local MP-PCA ", -t + time())
Time taken for local MP-PCA 112.24217367172241
Internally, the mppca
algorithm denoises the diffusion-weighted data
using a 3D sliding window which is defined by the variable patch_radius.
In total, this window should comprise a larger number of voxels than the
number of diffusion-weighted volumes. Since our data has a total of 67
volumes, the patch_radius is set to 2 which corresponds to a 5x5x5 sliding
window comprising a total of 125 voxels.
# To assess the performance of the Marchenko-Pastur PCA denoising algorithm,
# an axial slice of the original data, denoised data, and residuals are
# plotted below:
sli = data.shape[2] // 2
gra = data.shape[3] - 1
orig = data[:, :, sli, gra]
den = denoised_arr[:, :, sli, gra]
rms_diff = np.sqrt((orig - den) ** 2)
fig1, ax = plt.subplots(1, 3, figsize=(12, 6),
subplot_kw={'xticks': [], 'yticks': []})
fig1.subplots_adjust(hspace=0.3, wspace=0.05)
ax.flat[0].imshow(orig.T, cmap='gray', interpolation='none',
origin='lower')
ax.flat[0].set_title('Original')
ax.flat[1].imshow(den.T, cmap='gray', interpolation='none',
origin='lower')
ax.flat[1].set_title('Denoised Output')
ax.flat[2].imshow(rms_diff.T, cmap='gray', interpolation='none',
origin='lower')
ax.flat[2].set_title('Residuals')
fig1.savefig('denoised_mppca.png')
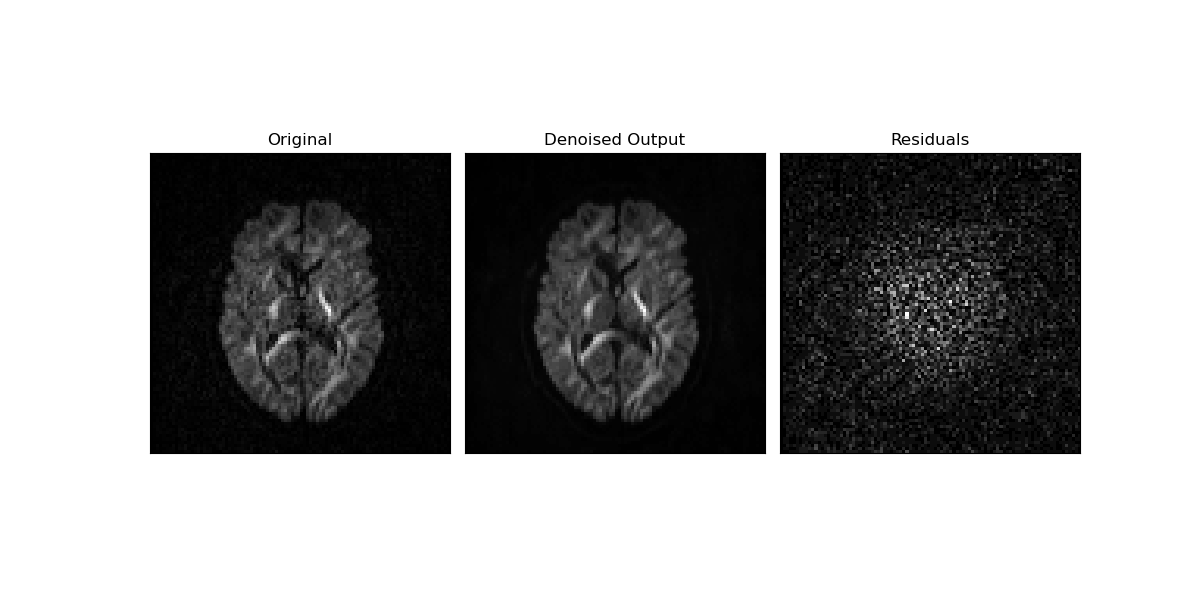
The noise suppression can be visually appreciated by comparing the original data slice (left panel) to its denoised version (middle panel). The difference between original and denoised data showing only random noise indicates that the data’s structural information is preserved by the PCA denoising algorithm (right panel).
Below we show how the denoised data can be saved.
save_nifti('denoised_mppca.nii.gz', denoised_arr, affine)
Additionally, we show how the PCA denoising algorithm affects different diffusion measurements. For this, we run the diffusion kurtosis model below on both original and denoised versions of the data:
dkimodel = dki.DiffusionKurtosisModel(gtab)
maskdata, mask = median_otsu(data, vol_idx=[0, 1], median_radius=4, numpass=2,
autocrop=False, dilate=1)
dki_orig = dkimodel.fit(data, mask=mask)
dki_den = dkimodel.fit(denoised_arr, mask=mask)
0%| | 0/57378 [00:00<?, ?it/s]
1%|▌ | 317/57378 [00:00<00:18, 3142.10it/s]
1%|█▌ | 782/57378 [00:00<00:14, 4007.06it/s]
2%|██▎ | 1183/57378 [00:00<00:14, 3836.40it/s]
3%|███ | 1568/57378 [00:00<00:15, 3550.66it/s]
4%|███▉ | 2057/57378 [00:00<00:13, 3992.26it/s]
4%|████▉ | 2570/57378 [00:00<00:12, 4349.36it/s]
5%|█████▊ | 3010/57378 [00:00<00:12, 4219.07it/s]
6%|██████▋ | 3464/57378 [00:00<00:12, 4309.81it/s]
7%|███████▌ | 3898/57378 [00:00<00:12, 4309.13it/s]
8%|████████▍ | 4331/57378 [00:01<00:12, 4304.81it/s]
8%|█████████▏ | 4763/57378 [00:01<00:12, 4054.20it/s]
9%|██████████ | 5172/57378 [00:01<00:12, 4053.61it/s]
10%|██████████▊ | 5580/57378 [00:01<00:14, 3625.85it/s]
10%|███████████▌ | 5952/57378 [00:01<00:14, 3546.77it/s]
11%|████████████▎ | 6333/57378 [00:01<00:14, 3610.35it/s]
12%|████████████▉ | 6699/57378 [00:01<00:14, 3423.14it/s]
12%|█████████████▋ | 7104/57378 [00:01<00:14, 3586.81it/s]
13%|██████████████▌ | 7497/57378 [00:01<00:13, 3675.24it/s]
14%|███████████████▏ | 7869/57378 [00:02<00:13, 3575.88it/s]
14%|███████████████▉ | 8230/57378 [00:02<00:13, 3576.84it/s]
15%|████████████████▌ | 8590/57378 [00:02<00:14, 3379.01it/s]
16%|█████████████████▎ | 8932/57378 [00:02<00:14, 3291.85it/s]
16%|█████████████████▉ | 9264/57378 [00:02<00:14, 3291.60it/s]
17%|██████████████████▌ | 9595/57378 [00:02<00:14, 3289.90it/s]
17%|███████████████████▎ | 9960/57378 [00:02<00:13, 3387.66it/s]
18%|███████████████████▊ | 10304/57378 [00:02<00:13, 3395.06it/s]
19%|████████████████████▍ | 10675/57378 [00:02<00:13, 3479.07it/s]
19%|█████████████████████▎ | 11088/57378 [00:02<00:12, 3662.91it/s]
20%|█████████████████████▉ | 11455/57378 [00:03<00:13, 3375.63it/s]
21%|██████████████████████▋ | 11828/57378 [00:03<00:13, 3467.83it/s]
21%|███████████████████████▍ | 12197/57378 [00:03<00:12, 3524.63it/s]
22%|████████████████████████ | 12560/57378 [00:03<00:12, 3548.34it/s]
23%|████████████████████████▊ | 12927/57378 [00:03<00:12, 3578.81it/s]
23%|█████████████████████████▍ | 13290/57378 [00:03<00:12, 3584.56it/s]
24%|██████████████████████████▎ | 13699/57378 [00:03<00:11, 3722.21it/s]
25%|██████████████████████████▉ | 14073/57378 [00:03<00:12, 3590.07it/s]
25%|███████████████████████████▋ | 14434/57378 [00:03<00:12, 3485.77it/s]
26%|████████████████████████████▎ | 14792/57378 [00:04<00:12, 3504.29it/s]
27%|█████████████████████████████▏ | 15224/57378 [00:04<00:11, 3733.28it/s]
27%|██████████████████████████████ | 15698/57378 [00:04<00:10, 4026.58it/s]
28%|██████████████████████████████▉ | 16134/57378 [00:04<00:10, 4118.86it/s]
29%|███████████████████████████████▋ | 16548/57378 [00:04<00:10, 3990.04it/s]
30%|████████████████████████████████▋ | 17020/57378 [00:04<00:09, 4194.21it/s]
30%|█████████████████████████████████▍ | 17442/57378 [00:04<00:09, 4193.13it/s]
31%|██████████████████████████████████▏ | 17863/57378 [00:04<00:09, 3956.12it/s]
32%|███████████████████████████████████ | 18262/57378 [00:04<00:10, 3871.71it/s]
33%|███████████████████████████████████▊ | 18652/57378 [00:05<00:10, 3743.24it/s]
33%|████████████████████████████████████▍ | 19029/57378 [00:05<00:10, 3638.12it/s]
34%|█████████████████████████████████████▎ | 19466/57378 [00:05<00:09, 3834.79it/s]
35%|██████████████████████████████████████ | 19852/57378 [00:05<00:09, 3833.47it/s]
35%|██████████████████████████████████████▊ | 20237/57378 [00:05<00:10, 3520.22it/s]
36%|███████████████████████████████████████▍ | 20595/57378 [00:05<00:10, 3451.69it/s]
37%|████████████████████████████████████████▏ | 20944/57378 [00:05<00:10, 3435.59it/s]
37%|████████████████████████████████████████▊ | 21301/57378 [00:05<00:10, 3466.10it/s]
38%|█████████████████████████████████████████▌ | 21650/57378 [00:05<00:10, 3294.84it/s]
38%|██████████████████████████████████████████▏ | 21983/57378 [00:05<00:10, 3278.54it/s]
39%|██████████████████████████████████████████▊ | 22313/57378 [00:06<00:10, 3278.04it/s]
39%|███████████████████████████████████████████▍ | 22642/57378 [00:06<00:10, 3197.81it/s]
40%|████████████████████████████████████████████▏ | 23025/57378 [00:06<00:10, 3370.83it/s]
41%|████████████████████████████████████████████▉ | 23411/57378 [00:06<00:09, 3506.13it/s]
41%|█████████████████████████████████████████████▋ | 23808/57378 [00:06<00:09, 3633.62it/s]
42%|██████████████████████████████████████████████▎ | 24173/57378 [00:06<00:09, 3505.45it/s]
43%|███████████████████████████████████████████████ | 24536/57378 [00:06<00:09, 3535.86it/s]
43%|███████████████████████████████████████████████▊ | 24913/57378 [00:06<00:09, 3596.74it/s]
44%|████████████████████████████████████████████████▌ | 25317/57378 [00:06<00:08, 3719.92it/s]
45%|█████████████████████████████████████████████████▎ | 25690/57378 [00:07<00:08, 3607.40it/s]
45%|█████████████████████████████████████████████████▉ | 26053/57378 [00:07<00:08, 3607.12it/s]
46%|██████████████████████████████████████████████████▋ | 26415/57378 [00:07<00:08, 3601.47it/s]
47%|███████████████████████████████████████████████████▎ | 26776/57378 [00:07<00:08, 3492.24it/s]
47%|████████████████████████████████████████████████████ | 27127/57378 [00:07<00:08, 3389.22it/s]
48%|████████████████████████████████████████████████████▊ | 27573/57378 [00:07<00:08, 3686.61it/s]
49%|█████████████████████████████████████████████████████▌ | 27944/57378 [00:07<00:07, 3685.22it/s]
49%|██████████████████████████████████████████████████████▎ | 28314/57378 [00:07<00:08, 3574.31it/s]
50%|██████████████████████████████████████████████████████▉ | 28673/57378 [00:07<00:08, 3572.40it/s]
51%|███████████████████████████████████████████████████████▉ | 29153/57378 [00:07<00:07, 3921.46it/s]
52%|████████████████████████████████████████████████████████▋ | 29556/57378 [00:08<00:07, 3944.32it/s]
52%|█████████████████████████████████████████████████████████▍ | 29980/57378 [00:08<00:06, 4021.02it/s]
53%|██████████████████████████████████████████████████████████▏ | 30384/57378 [00:08<00:07, 3588.34it/s]
54%|██████████████████████████████████████████████████████████▉ | 30752/57378 [00:08<00:07, 3422.12it/s]
54%|███████████████████████████████████████████████████████████▋ | 31129/57378 [00:08<00:07, 3512.66it/s]
55%|████████████████████████████████████████████████████████████▎ | 31487/57378 [00:08<00:07, 3525.47it/s]
55%|█████████████████████████████████████████████████████████████ | 31844/57378 [00:08<00:07, 3453.87it/s]
56%|█████████████████████████████████████████████████████████████▋ | 32193/57378 [00:08<00:07, 3437.75it/s]
57%|██████████████████████████████████████████████████████████████▍ | 32596/57378 [00:08<00:06, 3599.30it/s]
58%|███████████████████████████████████████████████████████████████▎ | 33035/57378 [00:09<00:06, 3821.89it/s]
58%|████████████████████████████████████████████████████████████████ | 33430/57378 [00:09<00:06, 3850.26it/s]
59%|████████████████████████████████████████████████████████████████▊ | 33817/57378 [00:09<00:06, 3847.22it/s]
60%|█████████████████████████████████████████████████████████████████▌ | 34203/57378 [00:09<00:06, 3627.10it/s]
60%|██████████████████████████████████████████████████████████████████▎ | 34569/57378 [00:09<00:06, 3432.57it/s]
61%|███████████████████████████████████████████████████████████████████ | 34950/57378 [00:09<00:06, 3529.03it/s]
62%|███████████████████████████████████████████████████████████████████▋ | 35307/57378 [00:09<00:06, 3530.41it/s]
62%|████████████████████████████████████████████████████████████████████▎ | 35663/57378 [00:09<00:06, 3249.14it/s]
63%|█████████████████████████████████████████████████████████████████████ | 35994/57378 [00:09<00:06, 3260.94it/s]
63%|█████████████████████████████████████████████████████████████████████▋ | 36324/57378 [00:10<00:06, 3264.53it/s]
64%|██████████████████████████████████████████████████████████████████████▎ | 36660/57378 [00:10<00:06, 3285.55it/s]
64%|██████████████████████████████████████████████████████████████████████▉ | 36993/57378 [00:10<00:06, 3290.90it/s]
65%|███████████████████████████████████████████████████████████████████████▋ | 37367/57378 [00:10<00:05, 3411.11it/s]
66%|████████████████████████████████████████████████████████████████████████▌ | 37832/57378 [00:10<00:05, 3766.44it/s]
67%|█████████████████████████████████████████████████████████████████████████▎ | 38259/57378 [00:10<00:04, 3906.44it/s]
67%|██████████████████████████████████████████████████████████████████████████▏ | 38678/57378 [00:10<00:04, 3982.26it/s]
68%|██████████████████████████████████████████████████████████████████████████▉ | 39078/57378 [00:10<00:04, 3864.54it/s]
69%|███████████████████████████████████████████████████████████████████████████▋ | 39466/57378 [00:10<00:04, 3751.80it/s]
69%|████████████████████████████████████████████████████████████████████████████▍ | 39843/57378 [00:10<00:04, 3563.07it/s]
70%|█████████████████████████████████████████████████████████████████████████████▎ | 40352/57378 [00:11<00:04, 3982.00it/s]
71%|██████████████████████████████████████████████████████████████████████████████▏ | 40755/57378 [00:11<00:04, 3750.18it/s]
72%|██████████████████████████████████████████████████████████████████████████████▊ | 41136/57378 [00:11<00:04, 3760.60it/s]
72%|███████████████████████████████████████████████████████████████████████████████▌ | 41516/57378 [00:11<00:04, 3562.43it/s]
73%|████████████████████████████████████████████████████████████████████████████████▎ | 41877/57378 [00:11<00:04, 3473.82it/s]
74%|████████████████████████████████████████████████████████████████████████████████▉ | 42228/57378 [00:11<00:04, 3478.12it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▋ | 42578/57378 [00:11<00:04, 3292.16it/s]
75%|██████████████████████████████████████████████████████████████████████████████████▎ | 42967/57378 [00:11<00:04, 3451.55it/s]
76%|███████████████████████████████████████████████████████████████████████████████████ | 43324/57378 [00:11<00:04, 3479.34it/s]
76%|███████████████████████████████████████████████████████████████████████████████████▋ | 43675/57378 [00:12<00:04, 3309.00it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▎ | 44009/57378 [00:12<00:04, 3293.18it/s]
77%|█████████████████████████████████████████████████████████████████████████████████████ | 44341/57378 [00:12<00:04, 3128.86it/s]
78%|█████████████████████████████████████████████████████████████████████████████████████▋ | 44669/57378 [00:12<00:04, 3163.53it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▏ | 44988/57378 [00:12<00:03, 3098.12it/s]
79%|██████████████████████████████████████████████████████████████████████████████████████▉ | 45345/57378 [00:12<00:03, 3207.67it/s]
80%|███████████████████████████████████████████████████████████████████████████████████████▊ | 45780/57378 [00:12<00:03, 3527.34it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▍ | 46135/57378 [00:12<00:03, 3407.61it/s]
81%|█████████████████████████████████████████████████████████████████████████████████████████ | 46478/57378 [00:12<00:03, 3407.93it/s]
82%|█████████████████████████████████████████████████████████████████████████████████████████▊ | 46821/57378 [00:13<00:03, 3408.35it/s]
82%|██████████████████████████████████████████████████████████████████████████████████████████▍ | 47163/57378 [00:13<00:02, 3405.15it/s]
83%|███████████████████████████████████████████████████████████████████████████████████████████ | 47505/57378 [00:13<00:02, 3323.22it/s]
83%|███████████████████████████████████████████████████████████████████████████████████████████▊ | 47872/57378 [00:13<00:02, 3416.79it/s]
84%|████████████████████████████████████████████████████████████████████████████████████████████▌ | 48285/57378 [00:13<00:02, 3616.80it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▎ | 48648/57378 [00:13<00:02, 3510.74it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▉ | 49001/57378 [00:13<00:02, 3488.19it/s]
86%|██████████████████████████████████████████████████████████████████████████████████████████████▋ | 49359/57378 [00:13<00:02, 3509.43it/s]
87%|███████████████████████████████████████████████████████████████████████████████████████████████▎ | 49749/57378 [00:13<00:02, 3616.89it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████ | 50112/57378 [00:13<00:02, 3611.61it/s]
88%|████████████████████████████████████████████████████████████████████████████████████████████████▊ | 50474/57378 [00:14<00:01, 3501.64it/s]
89%|█████████████████████████████████████████████████████████████████████████████████████████████████▍ | 50826/57378 [00:14<00:01, 3305.41it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████▏ | 51238/57378 [00:14<00:01, 3525.47it/s]
90%|██████████████████████████████████████████████████████████████████████████████████████████████████▉ | 51594/57378 [00:14<00:01, 3525.42it/s]
91%|███████████████████████████████████████████████████████████████████████████████████████████████████▋ | 51973/57378 [00:14<00:01, 3594.18it/s]
91%|████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 52357/57378 [00:14<00:01, 3656.68it/s]
92%|█████████████████████████████████████████████████████████████████████████████████████████████████████ | 52724/57378 [00:14<00:01, 3653.59it/s]
93%|█████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 53091/57378 [00:14<00:01, 3649.51it/s]
93%|██████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 53457/57378 [00:14<00:01, 3559.63it/s]
94%|███████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 53814/57378 [00:14<00:01, 3553.82it/s]
95%|████████████████████████████████████████████████████████████████████████████████████████████████████████ | 54305/57378 [00:15<00:00, 3941.86it/s]
95%|████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 54701/57378 [00:15<00:00, 3719.88it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 55077/57378 [00:15<00:00, 3620.37it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 55487/57378 [00:15<00:00, 3749.18it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████████ | 55865/57378 [00:15<00:00, 3623.83it/s]
98%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 56230/57378 [00:15<00:00, 3445.82it/s]
99%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 56578/57378 [00:15<00:00, 3428.63it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▏| 56923/57378 [00:15<00:00, 3331.91it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▊| 57302/57378 [00:15<00:00, 3455.18it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████| 57378/57378 [00:15<00:00, 3588.71it/s]
0%| | 0/57378 [00:00<?, ?it/s]
1%|▋ | 321/57378 [00:00<00:17, 3187.55it/s]
1%|█▍ | 747/57378 [00:00<00:14, 3799.04it/s]
2%|██▏ | 1127/57378 [00:00<00:15, 3620.64it/s]
3%|██▉ | 1537/57378 [00:00<00:14, 3792.84it/s]
3%|███▋ | 1918/57378 [00:00<00:15, 3666.52it/s]
4%|████▍ | 2302/57378 [00:00<00:14, 3715.00it/s]
5%|█████▏ | 2675/57378 [00:00<00:14, 3713.34it/s]
5%|█████▉ | 3047/57378 [00:00<00:14, 3709.26it/s]
6%|██████▋ | 3430/57378 [00:00<00:14, 3737.11it/s]
7%|███████▍ | 3873/57378 [00:01<00:13, 3940.01it/s]
7%|████████▎ | 4268/57378 [00:01<00:13, 3935.18it/s]
8%|█████████▏ | 4748/57378 [00:01<00:12, 4187.14it/s]
9%|█████████▉ | 5167/57378 [00:01<00:13, 4012.54it/s]
10%|██████████▊ | 5570/57378 [00:01<00:13, 3830.37it/s]
10%|███████████▌ | 5956/57378 [00:01<00:13, 3724.47it/s]
11%|████████████▏ | 6331/57378 [00:01<00:14, 3640.22it/s]
12%|█████████████ | 6743/57378 [00:01<00:13, 3768.85it/s]
12%|█████████████▊ | 7122/57378 [00:01<00:13, 3662.09it/s]
13%|██████████████▍ | 7490/57378 [00:01<00:13, 3639.06it/s]
14%|███████████████▏ | 7855/57378 [00:02<00:13, 3634.83it/s]
14%|███████████████▉ | 8245/57378 [00:02<00:13, 3702.74it/s]
15%|████████████████▋ | 8616/57378 [00:02<00:13, 3588.01it/s]
16%|█████████████████▎ | 8976/57378 [00:02<00:13, 3482.44it/s]
16%|██████████████████▏ | 9382/57378 [00:02<00:13, 3640.92it/s]
17%|███████████████████ | 9862/57378 [00:02<00:11, 3969.52it/s]
18%|███████████████████▋ | 10262/57378 [00:02<00:12, 3751.72it/s]
19%|████████████████████▍ | 10641/57378 [00:02<00:12, 3755.20it/s]
19%|█████████████████████▏ | 11020/57378 [00:02<00:12, 3653.24it/s]
20%|█████████████████████▊ | 11388/57378 [00:03<00:12, 3655.46it/s]
20%|██████████████████████▌ | 11755/57378 [00:03<00:13, 3449.48it/s]
21%|███████████████████████▏ | 12103/57378 [00:03<00:13, 3266.18it/s]
22%|███████████████████████▊ | 12433/57378 [00:03<00:14, 3181.66it/s]
22%|████████████████████████▌ | 12793/57378 [00:03<00:13, 3291.09it/s]
23%|█████████████████████████▏ | 13148/57378 [00:03<00:13, 3356.38it/s]
24%|█████████████████████████▉ | 13512/57378 [00:03<00:12, 3429.72it/s]
24%|██████████████████████████▌ | 13857/57378 [00:03<00:13, 3238.63it/s]
25%|███████████████████████████▏ | 14184/57378 [00:04<00:19, 2228.80it/s]
25%|███████████████████████████▉ | 14600/57378 [00:04<00:16, 2640.85it/s]
26%|████████████████████████████▊ | 15006/57378 [00:04<00:14, 2967.62it/s]
27%|█████████████████████████████▋ | 15510/57378 [00:04<00:12, 3481.68it/s]
28%|██████████████████████████████▍ | 15897/57378 [00:04<00:12, 3440.79it/s]
28%|███████████████████████████████▏ | 16268/57378 [00:04<00:11, 3461.21it/s]
29%|███████████████████████████████▉ | 16633/57378 [00:04<00:11, 3412.20it/s]
30%|████████████████████████████████▌ | 16999/57378 [00:04<00:11, 3471.52it/s]
30%|█████████████████████████████████▎ | 17395/57378 [00:04<00:11, 3601.13it/s]
31%|██████████████████████████████████▏ | 17863/57378 [00:05<00:10, 3901.52it/s]
32%|███████████████████████████████████ | 18260/57378 [00:05<00:10, 3824.66it/s]
33%|███████████████████████████████████▊ | 18648/57378 [00:05<00:10, 3700.86it/s]
33%|████████████████████████████████████▍ | 19022/57378 [00:05<00:10, 3704.60it/s]
34%|█████████████████████████████████████▎ | 19450/57378 [00:05<00:09, 3861.72it/s]
35%|██████████████████████████████████████▏ | 19918/57378 [00:05<00:09, 4090.18it/s]
35%|██████████████████████████████████████▉ | 20330/57378 [00:05<00:09, 3974.86it/s]
36%|███████████████████████████████████████▋ | 20730/57378 [00:05<00:09, 3673.68it/s]
37%|████████████████████████████████████████▌ | 21131/57378 [00:05<00:09, 3757.53it/s]
37%|█████████████████████████████████████████▏ | 21512/57378 [00:06<00:11, 3216.33it/s]
38%|█████████████████████████████████████████▉ | 21888/57378 [00:06<00:10, 3351.24it/s]
39%|██████████████████████████████████████████▋ | 22245/57378 [00:06<00:10, 3402.56it/s]
39%|███████████████████████████████████████████▎ | 22595/57378 [00:06<00:10, 3403.10it/s]
40%|███████████████████████████████████████████▉ | 22943/57378 [00:06<00:10, 3416.16it/s]
41%|████████████████████████████████████████████▋ | 23339/57378 [00:06<00:09, 3564.73it/s]
41%|█████████████████████████████████████████████▍ | 23716/57378 [00:06<00:09, 3617.15it/s]
42%|██████████████████████████████████████████████▏ | 24081/57378 [00:06<00:09, 3516.59it/s]
43%|██████████████████████████████████████████████▉ | 24495/57378 [00:06<00:08, 3689.63it/s]
43%|███████████████████████████████████████████████▊ | 24931/57378 [00:06<00:08, 3874.73it/s]
44%|████████████████████████████████████████████████▌ | 25321/57378 [00:07<00:08, 3782.57it/s]
45%|█████████████████████████████████████████████████▎ | 25702/57378 [00:07<00:08, 3761.46it/s]
45%|█████████████████████████████████████████████████▉ | 26080/57378 [00:07<00:08, 3650.05it/s]
46%|██████████████████████████████████████████████████▊ | 26496/57378 [00:07<00:08, 3789.79it/s]
47%|███████████████████████████████████████████████████▌ | 26877/57378 [00:07<00:08, 3783.25it/s]
48%|████████████████████████████████████████████████████▎ | 27257/57378 [00:07<00:07, 3779.00it/s]
48%|████████████████████████████████████████████████████▉ | 27636/57378 [00:07<00:07, 3774.70it/s]
49%|█████████████████████████████████████████████████████▋ | 28014/57378 [00:07<00:07, 3769.49it/s]
49%|██████████████████████████████████████████████████████▍ | 28392/57378 [00:07<00:07, 3762.99it/s]
50%|███████████████████████████████████████████████████████▏ | 28769/57378 [00:08<00:07, 3671.59it/s]
51%|███████████████████████████████████████████████████████▊ | 29137/57378 [00:08<00:07, 3561.20it/s]
51%|████████████████████████████████████████████████████████▌ | 29495/57378 [00:08<00:07, 3558.29it/s]
52%|█████████████████████████████████████████████████████████▏ | 29852/57378 [00:08<00:07, 3532.96it/s]
53%|█████████████████████████████████████████████████████████▉ | 30206/57378 [00:08<00:07, 3528.28it/s]
53%|██████████████████████████████████████████████████████████▋ | 30580/57378 [00:08<00:07, 3583.82it/s]
54%|███████████████████████████████████████████████████████████▎ | 30939/57378 [00:08<00:07, 3380.53it/s]
55%|███████████████████████████████████████████████████████████▉ | 31280/57378 [00:08<00:07, 3288.71it/s]
55%|████████████████████████████████████████████████████████████▋ | 31673/57378 [00:08<00:07, 3464.33it/s]
56%|█████████████████████████████████████████████████████████████▌ | 32090/57378 [00:08<00:06, 3659.87it/s]
57%|██████████████████████████████████████████████████████████████▏ | 32459/57378 [00:09<00:07, 3557.08it/s]
57%|███████████████████████████████████████████████████████████████▏ | 32958/57378 [00:09<00:06, 3960.85it/s]
58%|███████████████████████████████████████████████████████████████▉ | 33358/57378 [00:09<00:06, 3766.26it/s]
59%|████████████████████████████████████████████████████████████████▋ | 33739/57378 [00:09<00:06, 3644.26it/s]
59%|█████████████████████████████████████████████████████████████████▍ | 34107/57378 [00:09<00:06, 3451.33it/s]
60%|██████████████████████████████████████████████████████████████████▏ | 34506/57378 [00:09<00:06, 3592.91it/s]
61%|██████████████████████████████████████████████████████████████████▊ | 34870/57378 [00:09<00:06, 3597.35it/s]
61%|███████████████████████████████████████████████████████████████████▌ | 35233/57378 [00:09<00:06, 3525.99it/s]
62%|████████████████████████████████████████████████████████████████████▏ | 35588/57378 [00:09<00:06, 3483.12it/s]
63%|████████████████████████████████████████████████████████████████████▉ | 35938/57378 [00:10<00:06, 3419.33it/s]
63%|█████████████████████████████████████████████████████████████████████▌ | 36281/57378 [00:10<00:06, 3422.38it/s]
64%|██████████████████████████████████████████████████████████████████████▎ | 36648/57378 [00:10<00:05, 3494.29it/s]
65%|██████████████████████████████████████████████████████████████████████▉ | 37015/57378 [00:10<00:05, 3545.80it/s]
65%|███████████████████████████████████████████████████████████████████████▋ | 37371/57378 [00:10<00:05, 3399.44it/s]
66%|████████████████████████████████████████████████████████████████████████▎ | 37713/57378 [00:10<00:05, 3395.59it/s]
66%|████████████████████████████████████████████████████████████████████████▉ | 38054/57378 [00:10<00:05, 3358.71it/s]
67%|█████████████████████████████████████████████████████████████████████████▌ | 38391/57378 [00:10<00:05, 3355.20it/s]
67%|██████████████████████████████████████████████████████████████████████████▏ | 38728/57378 [00:10<00:05, 3354.41it/s]
68%|██████████████████████████████████████████████████████████████████████████▉ | 39064/57378 [00:10<00:05, 3348.31it/s]
69%|███████████████████████████████████████████████████████████████████████████▋ | 39505/57378 [00:11<00:04, 3652.15it/s]
70%|████████████████████████████████████████████████████████████████████████████▋ | 39980/57378 [00:11<00:04, 3969.45it/s]
70%|█████████████████████████████████████████████████████████████████████████████▍ | 40378/57378 [00:11<00:04, 3871.00it/s]
71%|██████████████████████████████████████████████████████████████████████████████▏ | 40766/57378 [00:11<00:04, 3844.04it/s]
72%|██████████████████████████████████████████████████████████████████████████████▉ | 41176/57378 [00:11<00:04, 3910.29it/s]
72%|███████████████████████████████████████████████████████████████████████████████▋ | 41568/57378 [00:11<00:04, 3902.58it/s]
73%|████████████████████████████████████████████████████████████████████████████████▍ | 41959/57378 [00:11<00:03, 3895.83it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▏ | 42349/57378 [00:11<00:03, 3798.36it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▉ | 42730/57378 [00:11<00:03, 3663.56it/s]
75%|██████████████████████████████████████████████████████████████████████████████████▌ | 43098/57378 [00:12<00:04, 3461.24it/s]
76%|███████████████████████████████████████████████████████████████████████████████████▍ | 43502/57378 [00:12<00:03, 3614.86it/s]
76%|████████████████████████████████████████████████████████████████████████████████████ | 43867/57378 [00:12<00:03, 3616.62it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▊ | 44231/57378 [00:12<00:03, 3615.47it/s]
78%|█████████████████████████████████████████████████████████████████████████████████████▍ | 44594/57378 [00:12<00:03, 3613.10it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▏ | 44957/57378 [00:12<00:03, 3465.85it/s]
79%|██████████████████████████████████████████████████████████████████████████████████████▉ | 45362/57378 [00:12<00:03, 3592.01it/s]
80%|███████████████████████████████████████████████████████████████████████████████████████▋ | 45723/57378 [00:12<00:03, 3591.35it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▎ | 46088/57378 [00:12<00:03, 3599.40it/s]
81%|█████████████████████████████████████████████████████████████████████████████████████████ | 46449/57378 [00:12<00:03, 3573.89it/s]
82%|█████████████████████████████████████████████████████████████████████████████████████████▉ | 46906/57378 [00:13<00:02, 3856.24it/s]
83%|██████████████████████████████████████████████████████████████████████████████████████████▊ | 47397/57378 [00:13<00:02, 4159.00it/s]
83%|███████████████████████████████████████████████████████████████████████████████████████████▋ | 47814/57378 [00:13<00:02, 3919.82it/s]
84%|████████████████████████████████████████████████████████████████████████████████████████████▍ | 48210/57378 [00:13<00:02, 3522.19it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▏ | 48587/57378 [00:13<00:02, 3582.92it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▊ | 48965/57378 [00:13<00:02, 3631.66it/s]
86%|██████████████████████████████████████████████████████████████████████████████████████████████▌ | 49334/57378 [00:13<00:02, 3642.74it/s]
87%|███████████████████████████████████████████████████████████████████████████████████████████████▎ | 49703/57378 [00:13<00:02, 3650.11it/s]
87%|███████████████████████████████████████████████████████████████████████████████████████████████▉ | 50071/57378 [00:13<00:02, 3547.89it/s]
88%|████████████████████████████████████████████████████████████████████████████████████████████████▋ | 50431/57378 [00:14<00:01, 3554.55it/s]
89%|█████████████████████████████████████████████████████████████████████████████████████████████████▍ | 50813/57378 [00:14<00:01, 3625.17it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████ | 51177/57378 [00:14<00:01, 3598.81it/s]
90%|██████████████████████████████████████████████████████████████████████████████████████████████████▊ | 51538/57378 [00:14<00:01, 3513.47it/s]
90%|███████████████████████████████████████████████████████████████████████████████████████████████████▍ | 51897/57378 [00:14<00:01, 3530.30it/s]
91%|████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 52251/57378 [00:14<00:01, 3527.04it/s]
92%|████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 52605/57378 [00:14<00:01, 3523.26it/s]
92%|█████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 52981/57378 [00:14<00:01, 3586.11it/s]
93%|██████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 53340/57378 [00:14<00:01, 3579.79it/s]
94%|███████████████████████████████████████████████████████████████████████████████████████████████████████ | 53740/57378 [00:14<00:00, 3696.00it/s]
94%|███████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 54110/57378 [00:15<00:00, 3688.61it/s]
95%|████████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 54479/57378 [00:15<00:00, 3420.06it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████ | 54825/57378 [00:15<00:00, 3283.72it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 55157/57378 [00:15<00:00, 3232.72it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 55483/57378 [00:15<00:00, 3131.64it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 55798/57378 [00:15<00:00, 2964.36it/s]
98%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 56220/57378 [00:15<00:00, 3301.62it/s]
99%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 56656/57378 [00:15<00:00, 3590.72it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▍| 57098/57378 [00:15<00:00, 3819.39it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████| 57378/57378 [00:16<00:00, 3584.66it/s]
We use the following code to plot the MD, FA and MK estimates from the two data fits:
FA_orig = dki_orig.fa
FA_den = dki_den.fa
MD_orig = dki_orig.md
MD_den = dki_den.md
MK_orig = dki_orig.mk(0, 3)
MK_den = dki_den.mk(0, 3)
fig2, ax = plt.subplots(2, 3, figsize=(10, 6),
subplot_kw={'xticks': [], 'yticks': []})
fig2.subplots_adjust(hspace=0.3, wspace=0.03)
ax.flat[0].imshow(MD_orig[:, :, sli].T, cmap='gray', vmin=0, vmax=2.0e-3,
origin='lower')
ax.flat[0].set_title('MD (DKI)')
ax.flat[1].imshow(FA_orig[:, :, sli].T, cmap='gray', vmin=0, vmax=0.7,
origin='lower')
ax.flat[1].set_title('FA (DKI)')
ax.flat[2].imshow(MK_orig[:, :, sli].T, cmap='gray', vmin=0, vmax=1.5,
origin='lower')
ax.flat[2].set_title('AD (DKI)')
ax.flat[3].imshow(MD_den[:, :, sli].T, cmap='gray', vmin=0, vmax=2.0e-3,
origin='lower')
ax.flat[3].set_title('MD (DKI)')
ax.flat[4].imshow(FA_den[:, :, sli].T, cmap='gray', vmin=0, vmax=0.7,
origin='lower')
ax.flat[4].set_title('FA (DKI)')
ax.flat[5].imshow(MK_den[:, :, sli].T, cmap='gray', vmin=0, vmax=1.5,
origin='lower')
ax.flat[5].set_title('AD (DKI)')
plt.show()
fig2.savefig('denoised_dki.png')
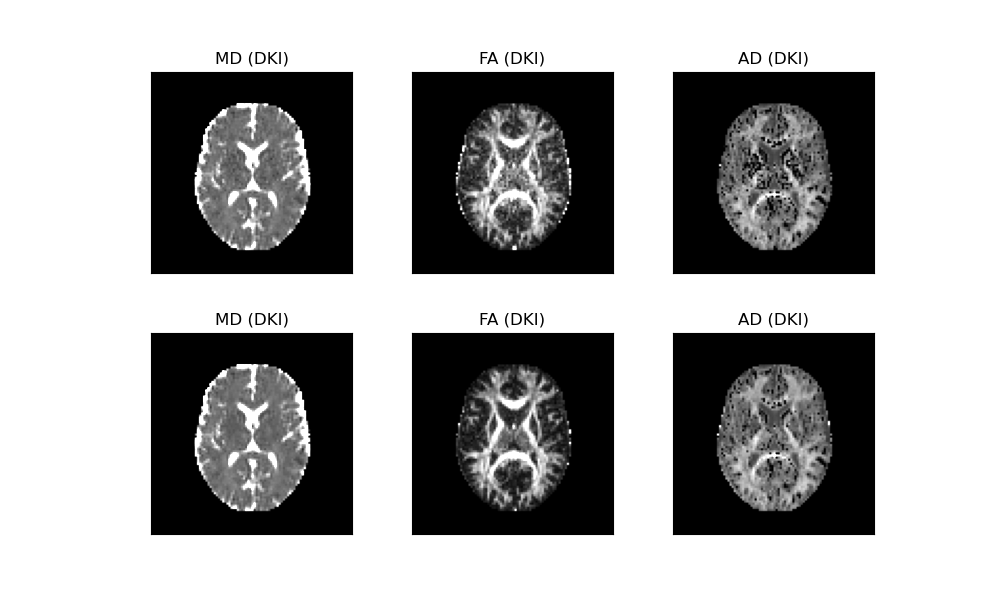
In the above figure, the DKI maps obtained from the original data are shown in the upper panels, while the DKI maps from the denoised data are shown in the lower panels. Substantial improvements in measurement robustness can be visually appreciated, particularly for the FA and MK estimates.
Noise standard deviation estimation using the Marchenko-Pastur PCA algorithm#
As mentioned above, the Marcenko-Pastur PCA algorithm can also be used to
estimate the image’s noise standard deviation (std). The noise std
automatically computed from the mppca
function can be returned by
setting the optional input parameter return_sigma
to True.
denoised_arr, sigma = mppca(data, patch_radius=2, return_sigma=True)
Let’s plot the noise standard deviation estimate:
fig3 = plt.figure('PCA Noise standard deviation estimation')
plt.imshow(sigma[..., sli].T, cmap='gray', origin='lower')
plt.axis('off')
plt.show()
fig3.savefig('pca_sigma.png')
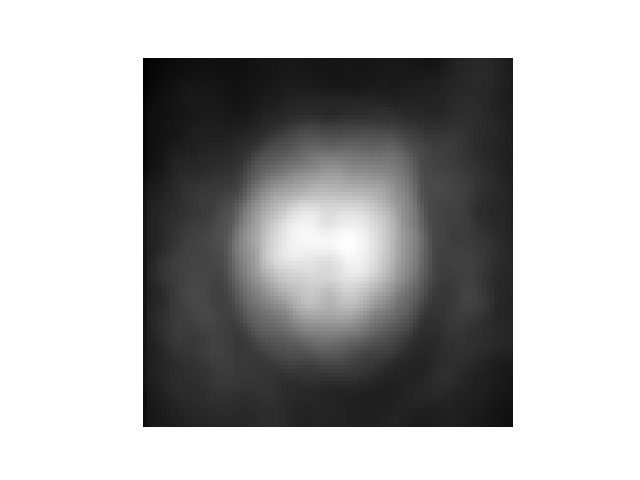
The above figure shows that the Marchenko-Pastur PCA algorithm computes a 3D spatial varying noise level map. To obtain the mean noise std across all voxels, you can use the following lines of code:
mean_sigma = np.mean(sigma[mask])
print(mean_sigma)
4.6821175
Below we use this mean noise level estimate to compute the nominal SNR of the data (i.e. SNR at b-value=0):
b0 = denoised_arr[..., 0]
mean_signal = np.mean(b0[mask])
snr = mean_signal / mean_sigma
print(snr)
34.55836398873534
References#
Manjon JV, Coupe P, Concha L, Buades A, Collins DL “Diffusion Weighted Image Denoising Using Overcomplete Local PCA” (2013) PLoS ONE 8(9): e73021. doi:10.1371/journal.pone.0073021.
Veraart J, Fieremans E, Novikov DS. 2016. Diffusion MRI noise mapping using random matrix theory. Magnetic Resonance in Medicine. doi: 10.1002/mrm.26059.
Henriques, R., 2018. Advanced Methods for Diffusion MRI Data Analysis and their Application to the Healthy Ageing Brain (Doctoral thesis). https://doi.org/10.17863/CAM.29356
Veraart J, Novikov DS, Christiaens D, Ades-aron B, Sijbers, Fieremans E, 2016. Denoising of Diffusion MRI using random matrix theory. Neuroimage 142:394-406. doi: 10.1016/j.neuroimage.2016.08.016
Total running time of the script: (10 minutes 29.439 seconds)