Note
Go to the end to download the full example code
Mean signal diffusion kurtosis imaging (MSDKI)#
Diffusion Kurtosis Imaging (DKI) is one of the conventional ways to estimate the degree of non-Gaussian diffusion (see Reconstruction of the diffusion signal with the kurtosis tensor model) [Jensen2005]. However, a limitation of DKI is that its measures are highly sensitive to noise and image artefacts. For instance, due to the low radial diffusivities, standard kurtosis estimates in regions of well-aligned voxel may be corrupted by implausible low or even negative values.
A way to overcome this issue is to characterize kurtosis from average signals across all directions acquired for each data b-value (also known as powder-averaged signals). Moreover, as previously pointed [NetoHe2015], standard kurtosis measures (e.g. radial, axial and standard mean kurtosis) do not only depend on microstructural properties but also on mesoscopic properties such as fiber dispersion or the intersection angle of crossing fibers. In contrary, the kurtosis from powder-average signals has the advantage of not depending on the fiber distribution functions [NetoHe2018], [NetoHe2019].
In short, in this tutorial we show how to characterize non-Gaussian diffusion in a more precise way and decoupled from confounding effects of tissue dispersion and crossing.
In the first part of this example, we illustrate the properties of the measures obtained from the mean signal diffusion kurtosis imaging (MSDKI)[NetoHe2018]_ using synthetic data. Secondly, the mean signal diffusion kurtosis imaging will be applied to in-vivo MRI data. Finally, we show how MSDKI provides the same information than common microstructural models such as the spherical mean technique [NetoHe2019], [Kaden2016b].
Letβs import all relevant modules:
import numpy as np
import matplotlib.pyplot as plt
# Reconstruction modules
import dipy.reconst.dki as dki
import dipy.reconst.msdki as msdki
# For simulations
from dipy.sims.voxel import multi_tensor
from dipy.core.gradients import gradient_table
from dipy.core.sphere import disperse_charges, HemiSphere
# For in-vivo data
from dipy.data import get_fnames
from dipy.io.gradients import read_bvals_bvecs
from dipy.io.image import load_nifti
from dipy.segment.mask import median_otsu
Testing MSDKI in synthetic data#
We simulate representative diffusion-weighted signals using MultiTensor simulations (for more information on this type of simulations see MultiTensor Simulation). For this example, simulations are produced based on the sum of four diffusion tensors to represent the intra- and extra-cellular spaces of two fiber populations. The parameters of these tensors are adjusted according to [NetoHe2015] (see also DKI MultiTensor Simulation).
mevals = np.array([[0.00099, 0, 0],
[0.00226, 0.00087, 0.00087],
[0.00099, 0, 0],
[0.00226, 0.00087, 0.00087]])
For the acquisition parameters of the synthetic data, we use 60 gradient
directions for two non-zero b-values (1000 and 2000
# Sample the spherical coordinates of 60 random diffusion-weighted directions.
rng = np.random.default_rng()
n_pts = 60
theta = np.pi * rng.random(n_pts)
phi = 2 * np.pi * rng.random(n_pts)
# Convert direction to cartesian coordinates.
hsph_initial = HemiSphere(theta=theta, phi=phi)
# Evenly distribute the 60 directions
hsph_updated, potential = disperse_charges(hsph_initial, 5000)
directions = hsph_updated.vertices
# Reconstruct acquisition parameters for 2 non-zero=b-values and 2 b0s
bvals = np.hstack((np.zeros(2), 1000 * np.ones(n_pts), 2000 * np.ones(n_pts)))
bvecs = np.vstack((np.zeros((2, 3)), directions, directions))
gtab = gradient_table(bvals, bvecs)
Simulations are looped for different intra- and extra-cellular water volume fractions and different intersection angles of the two-fiber populations.
# Array containing the intra-cellular volume fractions tested
f = np.linspace(20, 80.0, num=7)
# Array containing the intersection angle
ang = np.linspace(0, 90.0, num=91)
# Matrix where synthetic signals will be stored
dwi = np.empty((f.size, ang.size, bvals.size))
for f_i in range(f.size):
# estimating volume fractions for individual tensors
fractions = np.array([100 - f[f_i], f[f_i], 100 - f[f_i], f[f_i]]) * 0.5
for a_i in range(ang.size):
# defining the directions for individual tensors
angles = [(ang[a_i], 0.0), (ang[a_i], 0.0), (0.0, 0.0), (0.0, 0.0)]
# producing signals using Dipy's function multi_tensor
signal, sticks = multi_tensor(gtab, mevals, S0=100, angles=angles,
fractions=fractions, snr=None)
dwi[f_i, a_i, :] = signal
Now that all synthetic signals were produced, we can go forward with MSDKI fitting. As other Dipyβs reconstruction techniques, the MSDKI model has to be first defined for the specific GradientTable object of the synthetic data. For MSDKI, this is done by instantiating the MeanDiffusionKurtosisModel object in the following way:
msdki_model = msdki.MeanDiffusionKurtosisModel(gtab)
MSDKI can then be fitted to the synthetic data by calling the fit
function of this object:
msdki_fit = msdki_model.fit(dwi)
From the above fit object we can extract the two main parameters of the MSDKI, i.e.: 1) the mean signal diffusion (MSD); and 2) the mean signal kurtosis (MSK)
MSD = msdki_fit.msd
MSK = msdki_fit.msk
For a reference, we also calculate the mean diffusivity (MD) and mean kurtosis (MK) from the standard DKI.
dki_model = dki.DiffusionKurtosisModel(gtab)
dki_fit = dki_model.fit(dwi)
MD = dki_fit.md
MK = dki_fit.mk(0, 3)
0%| | 0/637 [00:00<?, ?it/s]
56%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 355/637 [00:00<00:00, 3528.57it/s]
100%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 637/637 [00:00<00:00, 3301.70it/s]
Now we plot the results as a function of the ground truth intersection angle and for different volume fractions of intra-cellular water.
fig1, axs = plt.subplots(nrows=2, ncols=2, figsize=(10, 10))
for f_i in range(f.size):
axs[0, 0].plot(ang, MSD[f_i], linewidth=1.0,
label=':math:`F: %.2f`' % f[f_i])
axs[0, 1].plot(ang, MSK[f_i], linewidth=1.0,
label=':math:`F: %.2f`' % f[f_i])
axs[1, 0].plot(ang, MD[f_i], linewidth=1.0,
label=':math:`F: %.2f`' % f[f_i])
axs[1, 1].plot(ang, MK[f_i], linewidth=1.0,
label=':math:`F: %.2f`' % f[f_i])
# Adjust properties of the first panel of the figure
axs[0, 0].set_xlabel('Intersection angle')
axs[0, 0].set_ylabel('MSD')
axs[0, 1].set_xlabel('Intersection angle')
axs[0, 1].set_ylabel('MSK')
axs[0, 1].legend(loc='center left', bbox_to_anchor=(1, 0.5))
axs[1, 0].set_xlabel('Intersection angle')
axs[1, 0].set_ylabel('MD')
axs[1, 1].set_xlabel('Intersection angle')
axs[1, 1].set_ylabel('MK')
axs[1, 1].legend(loc='center left', bbox_to_anchor=(1, 0.5))
plt.show()
fig1.savefig('MSDKI_simulations.png')
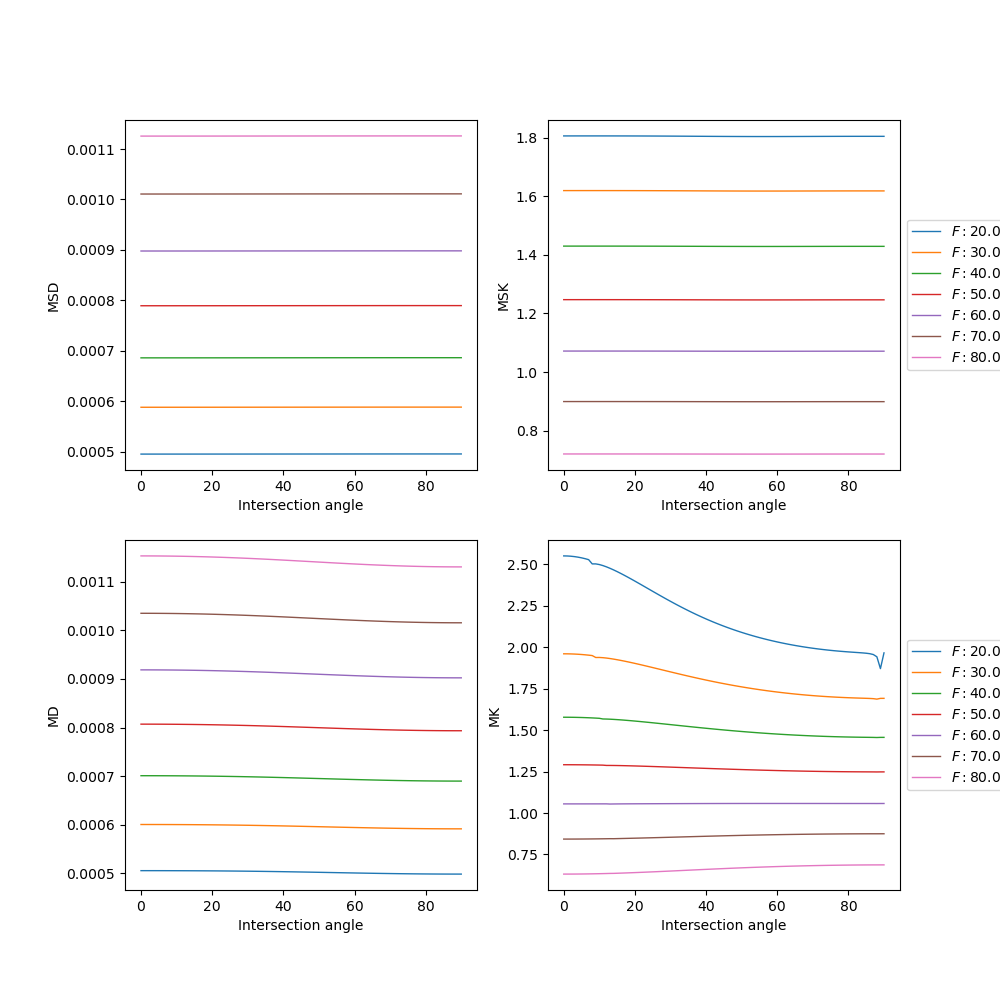
MSDKI and DKI measures for data of two crossing synthetic fibers. Upper panels show the MSDKI measures: 1) mean signal diffusivity (left panel); and 2) mean signal kurtosis (right panel). For reference, lower panels show the measures obtained by standard DKI: 1) mean diffusivity (left panel); and 2) mean kurtosis (right panel). All estimates are plotted as a function of the intersecting angle of the two crossing fibers. Different curves correspond to different ground truth axonal volume fraction of intra-cellular space.
The results of the above figure, demonstrate that both MSD and MSK are sensitive to axonal volume fraction (i.e. a microstructure property) but are independent to the intersection angle of the two crossing fibers (i.e. independent to properties regarding fiber orientation). In contrast, DKI measures seem to be independent to both axonal volume fraction and intersection angle.
Reconstructing diffusion data using MSDKI#
Now that the properties of MSDKI were illustrated, letβs apply MSDKI to in-vivo diffusion-weighted data. As the example for the standard DKI (see Reconstruction of the diffusion signal with the kurtosis tensor model), we use fetch to download a multi-shell dataset which was kindly provided by Hansen and Jespersen (more details about the data are provided in their paper [Hansen2016]). The total size of the downloaded data is 192 MBytes, however you only need to fetch it once.
fraw, fbval, fbvec, t1_fname = get_fnames('cfin_multib')
data, affine = load_nifti(fraw)
bvals, bvecs = read_bvals_bvecs(fbval, fbvec)
gtab = gradient_table(bvals, bvecs)
Before fitting the data, we perform some data pre-processing. For illustration, we only mask the data to avoid unnecessary calculations on the background of the image; however, you could also apply other pre-processing techniques. For example, some state of the art denoising algorithms are available in DIPY (e.g. the non-local means filter Denoise images using Non-Local Means (NLMEANS) or the local pca Denoise images using Local PCA via empirical thresholds).
maskdata, mask = median_otsu(data, vol_idx=[0, 1], median_radius=4, numpass=2,
autocrop=False, dilate=1)
Now that we have loaded and pre-processed the data we can go forward with MSDKI fitting. As for the synthetic data, the MSDKI model has to be first defined for the dataβs GradientTable object:
msdki_model = msdki.MeanDiffusionKurtosisModel(gtab)
The data can then be fitted by calling the fit
function of this object:
msdki_fit = msdki_model.fit(data, mask=mask)
Letβs then extract the two main MSDKIβs parameters: 1) mean signal diffusion (MSD); and 2) mean signal kurtosis (MSK).
MSD = msdki_fit.msd
MSK = msdki_fit.msk
For comparison, we calculate also the mean diffusivity (MD) and mean kurtosis (MK) from the standard DKI.
dki_model = dki.DiffusionKurtosisModel(gtab)
dki_fit = dki_model.fit(data, mask=mask)
MD = dki_fit.md
MK = dki_fit.mk(0, 3)
0%| | 0/57580 [00:00<?, ?it/s]
0%|β | 86/57580 [00:00<01:08, 842.54it/s]
0%|β | 180/57580 [00:00<01:04, 893.90it/s]
0%|β | 270/57580 [00:00<01:04, 884.92it/s]
1%|β | 359/57580 [00:00<01:04, 882.16it/s]
1%|β | 448/57580 [00:00<01:04, 881.93it/s]
1%|β | 548/57580 [00:00<01:02, 918.66it/s]
1%|ββ | 642/57580 [00:00<01:01, 922.88it/s]
1%|ββ | 735/57580 [00:00<01:01, 922.98it/s]
1%|ββ | 828/57580 [00:00<01:01, 922.78it/s]
2%|ββ | 921/57580 [00:01<01:01, 916.99it/s]
2%|ββ | 1013/57580 [00:01<01:04, 873.12it/s]
2%|βββ | 1101/57580 [00:01<01:08, 819.37it/s]
2%|βββ | 1184/57580 [00:01<01:08, 820.55it/s]
2%|βββ | 1267/57580 [00:01<01:08, 821.02it/s]
2%|βββ | 1365/57580 [00:01<01:05, 864.72it/s]
3%|βββ | 1452/57580 [00:01<01:06, 839.32it/s]
3%|βββ | 1549/57580 [00:01<01:04, 875.43it/s]
3%|ββββ | 1637/57580 [00:01<01:05, 855.37it/s]
3%|ββββ | 1727/57580 [00:01<01:04, 865.95it/s]
3%|ββββ | 1814/57580 [00:02<01:04, 859.77it/s]
3%|ββββ | 1901/57580 [00:02<01:04, 860.47it/s]
3%|ββββ | 1988/57580 [00:02<01:08, 813.43it/s]
4%|ββββ | 2079/57580 [00:02<01:06, 837.77it/s]
4%|βββββ | 2181/57580 [00:02<01:02, 887.49it/s]
4%|βββββ | 2273/57580 [00:02<01:01, 893.71it/s]
4%|βββββ | 2365/57580 [00:02<01:01, 899.07it/s]
4%|βββββ | 2456/57580 [00:02<01:01, 900.40it/s]
4%|βββββ | 2547/57580 [00:02<01:01, 901.41it/s]
5%|ββββββ | 2638/57580 [00:03<01:00, 901.70it/s]
5%|ββββββ | 2729/57580 [00:03<01:04, 850.24it/s]
5%|ββββββ | 2815/57580 [00:03<01:04, 850.60it/s]
5%|ββββββ | 2906/57580 [00:03<01:03, 865.50it/s]
5%|ββββββ | 2993/57580 [00:03<01:04, 840.31it/s]
5%|ββββββ | 3082/57580 [00:03<01:03, 852.79it/s]
6%|βββββββ | 3179/57580 [00:03<01:01, 884.84it/s]
6%|βββββββ | 3269/57580 [00:03<01:01, 886.38it/s]
6%|βββββββ | 3358/57580 [00:03<01:03, 859.58it/s]
6%|βββββββ | 3445/57580 [00:03<01:02, 860.71it/s]
6%|βββββββ | 3532/57580 [00:04<01:02, 861.57it/s]
6%|βββββββ | 3619/57580 [00:04<01:02, 861.66it/s]
6%|ββββββββ | 3723/57580 [00:04<00:59, 912.17it/s]
7%|ββββββββ | 3819/57580 [00:04<00:58, 923.99it/s]
7%|ββββββββ | 3914/57580 [00:04<00:57, 928.91it/s]
7%|ββββββββ | 4007/57580 [00:04<00:59, 900.57it/s]
7%|ββββββββ | 4107/57580 [00:04<00:57, 927.53it/s]
7%|βββββββββ | 4200/57580 [00:04<01:01, 874.62it/s]
7%|βββββββββ | 4289/57580 [00:04<01:00, 877.02it/s]
8%|βββββββββ | 4378/57580 [00:04<01:00, 878.97it/s]
8%|βββββββββ | 4467/57580 [00:05<01:01, 860.19it/s]
8%|βββββββββ | 4564/57580 [00:05<00:59, 890.02it/s]
8%|βββββββββ | 4657/57580 [00:05<00:58, 899.18it/s]
8%|ββββββββββ | 4748/57580 [00:05<00:59, 894.33it/s]
8%|ββββββββββ | 4838/57580 [00:05<00:59, 893.79it/s]
9%|ββββββββββ | 4938/57580 [00:05<00:57, 922.53it/s]
9%|ββββββββββ | 5032/57580 [00:05<00:56, 924.84it/s]
9%|ββββββββββ | 5125/57580 [00:05<00:58, 893.03it/s]
9%|βββββββββββ | 5217/57580 [00:05<00:59, 884.43it/s]
9%|βββββββββββ | 5306/57580 [00:06<01:00, 857.91it/s]
9%|βββββββββββ | 5393/57580 [00:06<01:02, 830.57it/s]
10%|βββββββββββ | 5477/57580 [00:06<01:04, 808.12it/s]
10%|βββββββββββ | 5559/57580 [00:06<01:04, 806.52it/s]
10%|βββββββββββ | 5651/57580 [00:06<01:03, 820.32it/s]
10%|ββββββββββββ | 5737/57580 [00:06<01:02, 829.94it/s]
10%|ββββββββββββ | 5821/57580 [00:06<01:02, 825.93it/s]
10%|ββββββββββββ | 5915/57580 [00:06<01:00, 856.99it/s]
10%|ββββββββββββ | 6001/57580 [00:06<01:03, 807.64it/s]
11%|ββββββββββββ | 6083/57580 [00:07<01:03, 808.99it/s]
11%|ββββββββββββ | 6165/57580 [00:07<01:04, 792.15it/s]
11%|βββββββββββββ | 6257/57580 [00:07<01:02, 826.85it/s]
11%|βββββββββββββ | 6344/57580 [00:07<01:01, 837.33it/s]
11%|βββββββββββββ | 6429/57580 [00:07<01:00, 839.07it/s]
11%|βββββββββββββ | 6514/57580 [00:07<01:01, 835.26it/s]
11%|βββββββββββββ | 6610/57580 [00:07<00:58, 870.06it/s]
12%|βββββββββββββ | 6712/57580 [00:07<00:55, 912.57it/s]
12%|ββββββββββββββ | 6804/57580 [00:07<00:55, 912.29it/s]
12%|ββββββββββββββ | 6896/57580 [00:07<00:55, 911.73it/s]
12%|ββββββββββββββ | 6988/57580 [00:08<00:56, 896.64it/s]
12%|ββββββββββββββ | 7078/57580 [00:08<00:57, 882.66it/s]
12%|ββββββββββββββ | 7167/57580 [00:08<00:57, 882.47it/s]
13%|ββββββββββββββ | 7256/57580 [00:08<00:58, 856.82it/s]
13%|βββββββββββββββ | 7342/57580 [00:08<00:58, 855.03it/s]
13%|βββββββββββββββ | 7428/57580 [00:08<00:58, 854.10it/s]
13%|βββββββββββββββ | 7514/57580 [00:08<01:00, 833.67it/s]
13%|βββββββββββββββ | 7607/57580 [00:08<00:58, 859.12it/s]
13%|βββββββββββββββ | 7694/57580 [00:08<00:58, 859.87it/s]
14%|ββββββββββββββββ | 7786/57580 [00:08<00:56, 874.55it/s]
14%|ββββββββββββββββ | 7874/57580 [00:09<00:58, 842.91it/s]
14%|ββββββββββββββββ | 7968/57580 [00:09<00:57, 868.68it/s]
14%|ββββββββββββββββ | 8062/57580 [00:09<00:55, 887.05it/s]
14%|ββββββββββββββββ | 8151/57580 [00:09<00:55, 885.28it/s]
14%|ββββββββββββββββ | 8240/57580 [00:09<00:55, 884.25it/s]
14%|βββββββββββββββββ | 8329/57580 [00:09<00:55, 884.26it/s]
15%|βββββββββββββββββ | 8423/57580 [00:09<00:54, 898.23it/s]
15%|βββββββββββββββββ | 8513/57580 [00:09<00:54, 896.59it/s]
15%|βββββββββββββββββ | 8603/57580 [00:09<00:55, 886.40it/s]
15%|βββββββββββββββββ | 8718/57580 [00:09<00:51, 950.81it/s]
15%|ββββββββββββββββββ | 8814/57580 [00:10<00:52, 923.26it/s]
15%|ββββββββββββββββββ | 8907/57580 [00:10<00:53, 917.36it/s]
16%|ββββββββββββββββββ | 8999/57580 [00:10<00:59, 819.60it/s]
16%|ββββββββββββββββββ | 9083/57580 [00:10<00:58, 822.77it/s]
16%|ββββββββββββββββββ | 9167/57580 [00:10<00:58, 825.81it/s]
16%|ββββββββββββββββββ | 9251/57580 [00:10<00:59, 809.24it/s]
16%|βββββββββββββββββββ | 9333/57580 [00:10<00:59, 805.89it/s]
16%|βββββββββββββββββββ | 9435/57580 [00:10<00:55, 864.35it/s]
17%|βββββββββββββββββββ | 9531/57580 [00:10<00:54, 889.42it/s]
17%|βββββββββββββββββββ | 9621/57580 [00:11<00:53, 890.03it/s]
17%|βββββββββββββββββββ | 9711/57580 [00:11<00:56, 840.62it/s]
17%|βββββββββββββββββββ | 9800/57580 [00:11<00:56, 852.08it/s]
17%|ββββββββββββββββββββ | 9897/57580 [00:11<00:53, 883.54it/s]
17%|ββββββββββββββββββββ | 9987/57580 [00:11<00:53, 885.73it/s]
18%|ββββββββββββββββββββ | 10077/57580 [00:11<00:53, 887.86it/s]
18%|ββββββββββββββββββββ | 10167/57580 [00:11<00:53, 889.23it/s]
18%|ββββββββββββββββββββ | 10257/57580 [00:11<00:53, 889.84it/s]
18%|ββββββββββββββββββββ | 10348/57580 [00:11<00:52, 893.33it/s]
18%|ββββββββββββββββββββ | 10438/57580 [00:11<00:52, 893.36it/s]
18%|βββββββββββββββββββββ | 10528/57580 [00:12<00:52, 892.79it/s]
18%|βββββββββββββββββββββ | 10625/57580 [00:12<00:51, 913.50it/s]
19%|βββββββββββββββββββββ | 10717/57580 [00:12<00:51, 912.83it/s]
19%|βββββββββββββββββββββ | 10809/57580 [00:12<00:54, 864.43it/s]
19%|βββββββββββββββββββββ | 10896/57580 [00:12<00:55, 839.33it/s]
19%|ββββββββββββββββββββββ | 10981/57580 [00:12<00:55, 835.59it/s]
19%|ββββββββββββββββββββββ | 11080/57580 [00:12<00:52, 877.48it/s]
19%|ββββββββββββββββββββββ | 11176/57580 [00:12<00:51, 898.97it/s]
20%|ββββββββββββββββββββββ | 11267/57580 [00:12<00:52, 874.44it/s]
20%|ββββββββββββββββββββββ | 11355/57580 [00:13<00:54, 843.20it/s]
20%|ββββββββββββββββββββββ | 11440/57580 [00:13<00:56, 822.20it/s]
20%|βββββββββββββββββββββββ | 11523/57580 [00:13<00:59, 778.02it/s]
20%|βββββββββββββββββββββββ | 11612/57580 [00:13<00:56, 807.20it/s]
20%|βββββββββββββββββββββββ | 11701/57580 [00:13<00:55, 828.84it/s]
21%|βββββββββββββββββββββββ | 11805/57580 [00:13<00:51, 887.04it/s]
21%|βββββββββββββββββββββββ | 11895/57580 [00:13<00:51, 888.55it/s]
21%|βββββββββββββββββββββββ | 11985/57580 [00:13<00:51, 889.61it/s]
21%|ββββββββββββββββββββββββ | 12075/57580 [00:13<00:53, 854.57it/s]
21%|ββββββββββββββββββββββββ | 12161/57580 [00:14<00:55, 817.25it/s]
21%|ββββββββββββββββββββββββ | 12244/57580 [00:14<00:56, 796.59it/s]
21%|ββββββββββββββββββββββββ | 12337/57580 [00:14<00:54, 832.05it/s]
22%|ββββββββββββββββββββββββ | 12421/57580 [00:14<00:54, 832.48it/s]
22%|ββββββββββββββββββββββββ | 12505/57580 [00:14<00:54, 832.28it/s]
22%|βββββββββββββββββββββββββ | 12593/57580 [00:14<00:53, 844.37it/s]
22%|βββββββββββββββββββββββββ | 12692/57580 [00:14<00:50, 884.87it/s]
22%|βββββββββββββββββββββββββ | 12802/57580 [00:14<00:47, 945.34it/s]
22%|βββββββββββββββββββββββββ | 12897/57580 [00:14<00:48, 916.92it/s]
23%|βββββββββββββββββββββββββ | 12989/57580 [00:14<00:48, 915.13it/s]
23%|ββββββββββββββββββββββββββ | 13081/57580 [00:15<00:49, 897.71it/s]
23%|ββββββββββββββββββββββββββ | 13171/57580 [00:15<00:51, 860.85it/s]
23%|ββββββββββββββββββββββββββ | 13258/57580 [00:15<00:51, 861.03it/s]
23%|ββββββββββββββββββββββββββ | 13345/57580 [00:15<00:51, 861.16it/s]
23%|ββββββββββββββββββββββββββ | 13432/57580 [00:15<00:51, 861.84it/s]
23%|ββββββββββββββββββββββββββ | 13519/57580 [00:15<00:51, 861.44it/s]
24%|βββββββββββββββββββββββββββ | 13606/57580 [00:15<00:53, 817.78it/s]
24%|βββββββββββββββββββββββββββ | 13689/57580 [00:15<00:53, 814.67it/s]
24%|βββββββββββββββββββββββββββ | 13775/57580 [00:15<00:53, 825.54it/s]
24%|βββββββββββββββββββββββββββ | 13861/57580 [00:15<00:52, 833.41it/s]
24%|βββββββββββββββββββββββββββ | 13945/57580 [00:16<00:53, 808.84it/s]
24%|βββββββββββββββββββββββββββ | 14027/57580 [00:16<00:53, 809.58it/s]
25%|ββββββββββββββββββββββββββββ | 14109/57580 [00:16<00:53, 809.90it/s]
25%|ββββββββββββββββββββββββββββ | 14191/57580 [00:16<00:53, 810.04it/s]
25%|ββββββββββββββββββββββββββββ | 14273/57580 [00:16<00:54, 787.89it/s]
25%|ββββββββββββββββββββββββββββ | 14354/57580 [00:16<00:54, 792.16it/s]
25%|ββββββββββββββββββββββββββββ | 14441/57580 [00:16<00:53, 812.77it/s]
25%|ββββββββββββββββββββββββββββ | 14530/57580 [00:16<00:51, 832.94it/s]
25%|βββββββββββββββββββββββββββββ | 14614/57580 [00:16<00:51, 832.75it/s]
26%|βββββββββββββββββββββββββββββ | 14698/57580 [00:17<00:53, 808.37it/s]
26%|βββββββββββββββββββββββββββββ | 14802/57580 [00:17<00:48, 873.04it/s]
26%|βββββββββββββββββββββββββββββ | 14914/57580 [00:17<00:45, 943.37it/s]
26%|βββββββββββββββββββββββββββββ | 15012/57580 [00:17<00:44, 951.56it/s]
26%|βββββββββββββββββββββββββββββ | 15108/57580 [00:17<00:44, 951.89it/s]
26%|ββββββββββββββββββββββββββββββ | 15204/57580 [00:17<00:44, 951.68it/s]
27%|ββββββββββββββββββββββββββββββ | 15300/57580 [00:17<00:45, 929.78it/s]
27%|ββββββββββββββββββββββββββββββ | 15394/57580 [00:17<00:46, 898.58it/s]
27%|ββββββββββββββββββββββββββββββ | 15485/57580 [00:17<00:46, 899.69it/s]
27%|ββββββββββββββββββββββββββββββ | 15576/57580 [00:17<00:47, 885.26it/s]
27%|βββββββββββββββββββββββββββββββ | 15665/57580 [00:18<00:50, 826.23it/s]
27%|βββββββββββββββββββββββββββββββ | 15749/57580 [00:18<00:51, 809.43it/s]
27%|βββββββββββββββββββββββββββββββ | 15831/57580 [00:18<00:53, 783.67it/s]
28%|βββββββββββββββββββββββββββββββ | 15912/57580 [00:18<00:52, 788.70it/s]
28%|βββββββββββββββββββββββββββββββ | 16009/57580 [00:18<00:49, 838.11it/s]
28%|βββββββββββββββββββββββββββββββ | 16104/57580 [00:18<00:47, 868.12it/s]
28%|ββββββββββββββββββββββββββββββββ | 16192/57580 [00:18<00:49, 844.26it/s]
28%|ββββββββββββββββββββββββββββββββ | 16277/57580 [00:18<00:48, 843.23it/s]
28%|ββββββββββββββββββββββββββββββββ | 16363/57580 [00:18<00:48, 845.30it/s]
29%|ββββββββββββββββββββββββββββββββ | 16449/57580 [00:19<00:48, 847.29it/s]
29%|ββββββββββββββββββββββββββββββββ | 16534/57580 [00:19<00:49, 821.52it/s]
29%|ββββββββββββββββββββββββββββββββ | 16625/57580 [00:19<00:48, 845.66it/s]
29%|βββββββββββββββββββββββββββββββββ | 16732/57580 [00:19<00:44, 909.17it/s]
29%|βββββββββββββββββββββββββββββββββ | 16846/57580 [00:19<00:41, 973.90it/s]
29%|βββββββββββββββββββββββββββββββββ | 16944/57580 [00:19<00:43, 944.91it/s]
30%|βββββββββββββββββββββββββββββββββ | 17039/57580 [00:19<00:44, 917.37it/s]
30%|βββββββββββββββββββββββββββββββββ | 17145/57580 [00:19<00:42, 955.30it/s]
30%|ββββββββββββββββββββββββββββββββββ | 17241/57580 [00:19<00:42, 954.40it/s]
30%|ββββββββββββββββββββββββββββββββββ | 17337/57580 [00:19<00:42, 953.51it/s]
30%|ββββββββββββββββββββββββββββββββββ | 17433/57580 [00:20<00:42, 953.02it/s]
30%|ββββββββββββββββββββββββββββββββββ | 17529/57580 [00:20<00:42, 952.58it/s]
31%|ββββββββββββββββββββββββββββββββββ | 17625/57580 [00:20<00:41, 951.57it/s]
31%|βββββββββββββββββββββββββββββββββββ | 17726/57580 [00:20<00:41, 968.69it/s]
31%|βββββββββββββββββββββββββββββββββββ | 17823/57580 [00:20<00:42, 940.55it/s]
31%|βββββββββββββββββββββββββββββββββββ | 17918/57580 [00:20<00:42, 935.19it/s]
31%|βββββββββββββββββββββββββββββββββββ | 18012/57580 [00:20<00:43, 907.36it/s]
31%|βββββββββββββββββββββββββββββββββββ | 18103/57580 [00:20<00:43, 905.87it/s]
32%|βββββββββββββββββββββββββββββββββββ | 18201/57580 [00:20<00:42, 925.11it/s]
32%|ββββββββββββββββββββββββββββββββββββ | 18294/57580 [00:21<00:42, 922.64it/s]
32%|ββββββββββββββββββββββββββββββββββββ | 18387/57580 [00:21<00:44, 871.45it/s]
32%|ββββββββββββββββββββββββββββββββββββ | 18475/57580 [00:21<00:44, 870.46it/s]
32%|ββββββββββββββββββββββββββββββββββββ | 18563/57580 [00:21<00:44, 870.90it/s]
32%|ββββββββββββββββββββββββββββββββββββ | 18659/57580 [00:21<00:43, 895.11it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 18749/57580 [00:21<00:43, 893.89it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 18839/57580 [00:21<00:44, 872.20it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 18927/57580 [00:21<00:44, 866.60it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 19014/57580 [00:21<00:44, 865.30it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 19101/57580 [00:21<00:45, 838.80it/s]
33%|βββββββββββββββββββββββββββββββββββββ | 19187/57580 [00:22<00:45, 842.56it/s]
33%|ββββββββββββββββββββββββββββββββββββββ | 19272/57580 [00:22<00:45, 844.72it/s]
34%|ββββββββββββββββββββββββββββββββββββββ | 19364/57580 [00:22<00:44, 864.60it/s]
34%|ββββββββββββββββββββββββββββββββββββββ | 19467/57580 [00:22<00:41, 910.68it/s]
34%|ββββββββββββββββββββββββββββββββββββββ | 19559/57580 [00:22<00:41, 910.44it/s]
34%|ββββββββββββββββββββββββββββββββββββββ | 19659/57580 [00:22<00:40, 934.65it/s]
34%|ββββββββββββββββββββββββββββββββββββββ | 19765/57580 [00:22<00:39, 968.78it/s]
34%|βββββββββββββββββββββββββββββββββββββββ | 19862/57580 [00:22<00:39, 966.07it/s]
35%|βββββββββββββββββββββββββββββββββββββββ | 19959/57580 [00:22<00:39, 964.43it/s]
35%|βββββββββββββββββββββββββββββββββββββββ | 20067/57580 [00:22<00:37, 995.25it/s]
35%|βββββββββββββββββββββββββββββββββββββββ | 20167/57580 [00:23<00:39, 957.91it/s]
35%|βββββββββββββββββββββββββββββββββββββββ | 20264/57580 [00:23<00:42, 885.35it/s]
35%|ββββββββββββββββββββββββββββββββββββββββ | 20354/57580 [00:23<00:44, 840.56it/s]
35%|ββββββββββββββββββββββββββββββββββββββββ | 20440/57580 [00:23<00:43, 844.44it/s]
36%|ββββββββββββββββββββββββββββββββββββββββ | 20526/57580 [00:23<00:43, 846.83it/s]
36%|ββββββββββββββββββββββββββββββββββββββββ | 20612/57580 [00:23<00:43, 848.02it/s]
36%|ββββββββββββββββββββββββββββββββββββββββ | 20698/57580 [00:23<00:43, 849.14it/s]
36%|ββββββββββββββββββββββββββββββββββββββββ | 20784/57580 [00:23<00:43, 849.52it/s]
36%|βββββββββββββββββββββββββββββββββββββββββ | 20870/57580 [00:23<00:43, 850.22it/s]
36%|βββββββββββββββββββββββββββββββββββββββββ | 20965/57580 [00:24<00:41, 877.30it/s]
37%|βββββββββββββββββββββββββββββββββββββββββ | 21056/57580 [00:24<00:41, 884.73it/s]
37%|βββββββββββββββββββββββββββββββββββββββββ | 21145/57580 [00:24<00:41, 883.97it/s]
37%|βββββββββββββββββββββββββββββββββββββββββ | 21247/57580 [00:24<00:39, 921.49it/s]
37%|ββββββββββββββββββββββββββββββββββββββββββ | 21350/57580 [00:24<00:38, 950.17it/s]
37%|ββββββββββββββββββββββββββββββββββββββββββ | 21454/57580 [00:24<00:37, 973.48it/s]
37%|ββββββββββββββββββββββββββββββββββββββββββ | 21552/57580 [00:24<00:37, 972.46it/s]
38%|ββββββββββββββββββββββββββββββββββββββββββ | 21650/57580 [00:24<00:38, 943.98it/s]
38%|ββββββββββββββββββββββββββββββββββββββββββ | 21745/57580 [00:24<00:39, 916.68it/s]
38%|ββββββββββββββββββββββββββββββββββββββββββ | 21837/57580 [00:24<00:39, 915.19it/s]
38%|βββββββββββββββββββββββββββββββββββββββββββ | 21929/57580 [00:25<00:39, 913.53it/s]
38%|βββββββββββββββββββββββββββββββββββββββββββ | 22021/57580 [00:25<00:40, 887.04it/s]
38%|βββββββββββββββββββββββββββββββββββββββββββ | 22110/57580 [00:25<00:40, 885.39it/s]
39%|βββββββββββββββββββββββββββββββββββββββββββ | 22203/57580 [00:25<00:39, 895.53it/s]
39%|βββββββββββββββββββββββββββββββββββββββββββ | 22293/57580 [00:25<00:40, 874.03it/s]
39%|ββββββββββββββββββββββββββββββββββββββββββββ | 22385/57580 [00:25<00:39, 884.80it/s]
39%|ββββββββββββββββββββββββββββββββββββββββββββ | 22484/57580 [00:25<00:38, 913.04it/s]
39%|ββββββββββββββββββββββββββββββββββββββββββββ | 22581/57580 [00:25<00:37, 926.51it/s]
39%|ββββββββββββββββββββββββββββββββββββββββββββ | 22674/57580 [00:25<00:37, 925.30it/s]
40%|ββββββββββββββββββββββββββββββββββββββββββββ | 22780/57580 [00:25<00:36, 962.36it/s]
40%|ββββββββββββββββββββββββββββββββββββββββββββ | 22877/57580 [00:26<00:36, 961.91it/s]
40%|βββββββββββββββββββββββββββββββββββββββββββββ | 22974/57580 [00:26<00:36, 959.04it/s]
40%|βββββββββββββββββββββββββββββββββββββββββββββ | 23070/57580 [00:26<00:36, 957.12it/s]
40%|βββββββββββββββββββββββββββββββββββββββββββββ | 23166/57580 [00:26<00:36, 955.24it/s]
40%|βββββββββββββββββββββββββββββββββββββββββββββ | 23262/57580 [00:26<00:36, 931.15it/s]
41%|βββββββββββββββββββββββββββββββββββββββββββββ | 23356/57580 [00:26<00:36, 925.58it/s]
41%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23449/57580 [00:26<00:37, 903.57it/s]
41%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23540/57580 [00:26<00:39, 872.32it/s]
41%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23634/57580 [00:26<00:38, 888.37it/s]
41%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23727/57580 [00:27<00:37, 897.80it/s]
41%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23817/57580 [00:27<00:38, 875.38it/s]
42%|ββββββββββββββββββββββββββββββββββββββββββββββ | 23905/57580 [00:27<00:38, 869.11it/s]
42%|βββββββββββββββββββββββββββββββββββββββββββββββ | 23997/57580 [00:27<00:38, 881.36it/s]
42%|βββββββββββββββββββββββββββββββββββββββββββββββ | 24097/57580 [00:27<00:36, 913.56it/s]
42%|βββββββββββββββββββββββββββββββββββββββββββββββ | 24208/57580 [00:27<00:34, 968.30it/s]
42%|βββββββββββββββββββββββββββββββββββββββββββββββ | 24307/57580 [00:27<00:34, 972.12it/s]
42%|βββββββββββββββββββββββββββββββββββββββββββββββ | 24405/57580 [00:27<00:34, 948.88it/s]
43%|ββββββββββββββββββββββββββββββββββββββββββββββββ | 24501/57580 [00:27<00:35, 922.70it/s]
43%|ββββββββββββββββββββββββββββββββββββββββββββββββ | 24602/57580 [00:27<00:34, 944.92it/s]
43%|ββββββββββββββββββββββββββββββββββββββββββββββββ | 24697/57580 [00:28<00:35, 916.53it/s]
43%|ββββββββββββββββββββββββββββββββββββββββββββββββ | 24789/57580 [00:28<00:36, 909.87it/s]
43%|ββββββββββββββββββββββββββββββββββββββββββββββββ | 24886/57580 [00:28<00:36, 904.19it/s]
43%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 24988/57580 [00:28<00:34, 935.01it/s]
44%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25095/57580 [00:28<00:33, 971.40it/s]
44%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25205/57580 [00:28<00:32, 1006.60it/s]
44%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25306/57580 [00:28<00:32, 999.37it/s]
44%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25417/57580 [00:28<00:31, 1028.62it/s]
44%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25523/57580 [00:28<00:30, 1034.87it/s]
45%|βββββββββββββββββββββββββββββββββββββββββββββββββ | 25639/57580 [00:28<00:29, 1069.03it/s]
45%|ββββββββββββββββββββββββββββββββββββββββββββββββββ | 25747/57580 [00:29<00:32, 981.05it/s]
45%|ββββββββββββββββββββββββββββββββββββββββββββββββββ | 25847/57580 [00:29<00:34, 931.03it/s]
45%|ββββββββββββββββββββββββββββββββββββββββββββββββββ | 25942/57580 [00:29<00:33, 934.14it/s]
45%|βββββββββββββββββββββββββββββββββββββββββββββββββββ | 26046/57580 [00:29<00:32, 961.69it/s]
45%|βββββββββββββββββββββββββββββββββββββββββββββββββββ | 26144/57580 [00:29<00:33, 937.55it/s]
46%|βββββββββββββββββββββββββββββββββββββββββββββββββββ | 26250/57580 [00:29<00:32, 969.24it/s]
46%|βββββββββββββββββββββββββββββββββββββββββββββββββββ | 26355/57580 [00:29<00:31, 989.39it/s]
46%|βββββββββββββββββββββββββββββββββββββββββββββββββββ | 26455/57580 [00:29<00:34, 909.48it/s]
46%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26548/57580 [00:30<00:34, 900.06it/s]
46%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26640/57580 [00:30<00:35, 869.00it/s]
46%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26728/57580 [00:30<00:35, 869.66it/s]
47%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26816/57580 [00:30<00:35, 869.96it/s]
47%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26904/57580 [00:30<00:35, 869.61it/s]
47%|ββββββββββββββββββββββββββββββββββββββββββββββββββββ | 26992/57580 [00:30<00:35, 870.16it/s]
47%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27089/57580 [00:30<00:33, 896.93it/s]
47%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27179/57580 [00:30<00:33, 895.65it/s]
47%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27269/57580 [00:30<00:33, 894.69it/s]
48%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27359/57580 [00:30<00:34, 873.68it/s]
48%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27447/57580 [00:31<00:35, 852.31it/s]
48%|βββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27539/57580 [00:31<00:34, 865.25it/s]
48%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27626/57580 [00:31<00:36, 811.92it/s]
48%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27713/57580 [00:31<00:36, 826.38it/s]
48%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27803/57580 [00:31<00:35, 845.65it/s]
48%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27889/57580 [00:31<00:35, 847.98it/s]
49%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 27975/57580 [00:31<00:34, 849.43it/s]
49%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28063/57580 [00:31<00:34, 856.35it/s]
49%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28149/57580 [00:31<00:35, 835.28it/s]
49%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28237/57580 [00:31<00:34, 845.76it/s]
49%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28324/57580 [00:32<00:34, 850.39it/s]
49%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28420/57580 [00:32<00:33, 880.15it/s]
50%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28521/57580 [00:32<00:31, 916.27it/s]
50%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28621/57580 [00:32<00:30, 937.64it/s]
50%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28715/57580 [00:32<00:30, 936.04it/s]
50%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28816/57580 [00:32<00:30, 955.01it/s]
50%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 28912/57580 [00:32<00:30, 948.59it/s]
50%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29007/57580 [00:32<00:30, 924.70it/s]
51%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29100/57580 [00:32<00:31, 892.85it/s]
51%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29195/57580 [00:33<00:31, 906.88it/s]
51%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29292/57580 [00:33<00:30, 922.81it/s]
51%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29398/57580 [00:33<00:29, 960.64it/s]
51%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29504/57580 [00:33<00:28, 986.94it/s]
51%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29603/57580 [00:33<00:28, 985.42it/s]
52%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29702/57580 [00:33<00:28, 984.15it/s]
52%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29801/57580 [00:33<00:29, 954.26it/s]
52%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29899/57580 [00:33<00:28, 958.61it/s]
52%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 29996/57580 [00:33<00:29, 931.97it/s]
52%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30090/57580 [00:33<00:30, 905.32it/s]
52%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30181/57580 [00:34<00:32, 853.23it/s]
53%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30276/57580 [00:34<00:31, 862.79it/s]
53%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30377/57580 [00:34<00:30, 901.96it/s]
53%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30468/57580 [00:34<00:30, 896.53it/s]
53%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30559/57580 [00:34<00:30, 897.84it/s]
53%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30674/57580 [00:34<00:27, 968.01it/s]
53%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30772/57580 [00:34<00:27, 968.77it/s]
54%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30870/57580 [00:34<00:27, 968.98it/s]
54%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 30968/57580 [00:34<00:28, 941.30it/s]
54%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31063/57580 [00:35<00:29, 914.29it/s]
54%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31155/57580 [00:35<00:28, 913.11it/s]
54%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31259/57580 [00:35<00:27, 947.57it/s]
54%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31355/57580 [00:35<00:28, 921.68it/s]
55%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31448/57580 [00:35<00:28, 922.31it/s]
55%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31541/57580 [00:35<00:28, 900.79it/s]
55%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31662/57580 [00:35<00:26, 986.68it/s]
55%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31768/57580 [00:35<00:25, 1005.38it/s]
55%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31876/57580 [00:35<00:25, 1024.18it/s]
56%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 31984/57580 [00:35<00:24, 1037.54it/s]
56%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32088/57580 [00:36<00:25, 996.27it/s]
56%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32189/57580 [00:36<00:26, 945.77it/s]
56%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32290/57580 [00:36<00:26, 961.62it/s]
56%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32387/57580 [00:36<00:26, 940.02it/s]
56%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32482/57580 [00:36<00:27, 909.81it/s]
57%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32574/57580 [00:36<00:27, 910.77it/s]
57%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32666/57580 [00:36<00:27, 890.78it/s]
57%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32756/57580 [00:36<00:28, 865.79it/s]
57%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32851/57580 [00:36<00:27, 887.97it/s]
57%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 32941/57580 [00:37<00:27, 883.42it/s]
57%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33036/57580 [00:37<00:27, 899.69it/s]
58%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33127/57580 [00:37<00:27, 900.44it/s]
58%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33218/57580 [00:37<00:27, 880.31it/s]
58%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33310/57580 [00:37<00:27, 889.76it/s]
58%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33409/57580 [00:37<00:26, 916.50it/s]
58%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33502/57580 [00:37<00:26, 918.54it/s]
58%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33605/57580 [00:37<00:25, 946.15it/s]
59%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33700/57580 [00:37<00:25, 938.55it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33794/57580 [00:37<00:25, 915.13it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33886/57580 [00:38<00:26, 900.14it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 33977/57580 [00:38<00:28, 819.58it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34069/57580 [00:38<00:27, 845.15it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34159/57580 [00:38<00:27, 858.04it/s]
59%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34249/57580 [00:38<00:26, 867.19it/s]
60%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34338/57580 [00:38<00:26, 871.80it/s]
60%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34443/57580 [00:38<00:25, 922.00it/s]
60%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34552/57580 [00:38<00:23, 968.78it/s]
60%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34669/57580 [00:38<00:22, 1024.85it/s]
60%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34772/57580 [00:39<00:23, 988.20it/s]
61%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34872/57580 [00:39<00:22, 988.31it/s]
61%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 34972/57580 [00:39<00:23, 960.90it/s]
61%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35069/57580 [00:39<00:23, 938.84it/s]
61%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35165/57580 [00:39<00:23, 941.88it/s]
61%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35260/57580 [00:39<00:23, 936.08it/s]
61%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35366/57580 [00:39<00:22, 969.38it/s]
62%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35468/57580 [00:39<00:22, 981.13it/s]
62%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35586/57580 [00:39<00:21, 1036.70it/s]
62%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35690/57580 [00:39<00:22, 976.39it/s]
62%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35789/57580 [00:40<00:22, 977.04it/s]
62%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35888/57580 [00:40<00:22, 949.68it/s]
62%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 35987/57580 [00:40<00:22, 958.09it/s]
63%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36084/57580 [00:40<00:22, 960.74it/s]
63%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36183/57580 [00:40<00:22, 969.11it/s]
63%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36281/57580 [00:40<00:22, 964.25it/s]
63%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36378/57580 [00:40<00:22, 935.78it/s]
63%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36472/57580 [00:40<00:23, 908.21it/s]
64%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36564/57580 [00:40<00:24, 859.34it/s]
64%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36651/57580 [00:41<00:24, 856.76it/s]
64%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36738/57580 [00:41<00:24, 836.62it/s]
64%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36822/57580 [00:41<00:24, 835.17it/s]
64%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36906/57580 [00:41<00:24, 834.21it/s]
64%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 36990/57580 [00:41<00:25, 810.36it/s]
64%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37072/57580 [00:41<00:25, 810.92it/s]
65%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37155/57580 [00:41<00:25, 813.74it/s]
65%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37245/57580 [00:41<00:24, 836.30it/s]
65%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37331/57580 [00:41<00:24, 840.74it/s]
65%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37417/57580 [00:41<00:23, 843.70it/s]
65%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37502/57580 [00:42<00:24, 818.17it/s]
65%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37591/57580 [00:42<00:23, 837.18it/s]
65%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37675/57580 [00:42<00:23, 836.78it/s]
66%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37777/57580 [00:42<00:22, 887.98it/s]
66%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37866/57580 [00:42<00:22, 860.06it/s]
66%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 37977/57580 [00:42<00:21, 929.80it/s]
66%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38086/57580 [00:42<00:20, 973.88it/s]
66%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38184/57580 [00:42<00:19, 973.50it/s]
66%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38282/57580 [00:42<00:21, 918.71it/s]
67%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38375/57580 [00:43<00:21, 894.14it/s]
67%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38465/57580 [00:43<00:22, 844.97it/s]
67%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38551/57580 [00:43<00:22, 847.04it/s]
67%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38637/57580 [00:43<00:22, 825.42it/s]
67%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38739/57580 [00:43<00:21, 878.34it/s]
67%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38837/57580 [00:43<00:20, 905.17it/s]
68%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 38929/57580 [00:43<00:21, 881.75it/s]
68%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39018/57580 [00:43<00:21, 881.87it/s]
68%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39107/57580 [00:43<00:21, 862.22it/s]
68%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39194/57580 [00:43<00:21, 862.58it/s]
68%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39295/57580 [00:44<00:20, 903.39it/s]
68%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39391/57580 [00:44<00:19, 917.57it/s]
69%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39483/57580 [00:44<00:20, 889.31it/s]
69%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39573/57580 [00:44<00:20, 876.92it/s]
69%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39676/57580 [00:44<00:19, 905.23it/s]
69%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39767/57580 [00:44<00:19, 898.71it/s]
69%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39866/57580 [00:44<00:19, 922.41it/s]
69%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 39967/57580 [00:44<00:18, 944.85it/s]
70%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40073/57580 [00:44<00:17, 975.80it/s]
70%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40181/57580 [00:44<00:17, 1004.01it/s]
70%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40282/57580 [00:45<00:17, 1003.43it/s]
70%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40383/57580 [00:45<00:17, 978.71it/s]
70%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40482/57580 [00:45<00:17, 974.08it/s]
70%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40580/57580 [00:45<00:17, 944.61it/s]
71%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40675/57580 [00:45<00:17, 943.29it/s]
71%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40770/57580 [00:45<00:18, 890.73it/s]
71%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40873/57580 [00:45<00:18, 927.15it/s]
71%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 40967/57580 [00:45<00:18, 902.54it/s]
71%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41075/57580 [00:45<00:17, 950.04it/s]
72%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41171/57580 [00:46<00:17, 946.42it/s]
72%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41267/57580 [00:46<00:17, 931.02it/s]
72%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41361/57580 [00:46<00:18, 853.96it/s]
72%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41448/57580 [00:46<00:18, 855.47it/s]
72%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41538/57580 [00:46<00:18, 865.73it/s]
72%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41629/57580 [00:46<00:18, 875.45it/s]
72%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41723/57580 [00:46<00:17, 891.22it/s]
73%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41814/57580 [00:46<00:17, 894.29it/s]
73%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 41922/57580 [00:46<00:16, 945.79it/s]
73%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42017/57580 [00:47<00:16, 944.20it/s]
73%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42112/57580 [00:47<00:17, 890.55it/s]
73%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42202/57580 [00:47<00:17, 890.90it/s]
73%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42292/57580 [00:47<00:17, 891.41it/s]
74%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42382/57580 [00:47<00:17, 891.74it/s]
74%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42472/57580 [00:47<00:16, 891.53it/s]
74%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42562/57580 [00:47<00:16, 891.97it/s]
74%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42652/57580 [00:47<00:17, 866.00it/s]
74%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42739/57580 [00:47<00:17, 865.01it/s]
74%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42826/57580 [00:47<00:17, 864.08it/s]
75%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 42919/57580 [00:48<00:16, 882.49it/s]
75%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43008/57580 [00:48<00:17, 853.07it/s]
75%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43094/57580 [00:48<00:17, 850.12it/s]
75%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43196/57580 [00:48<00:16, 895.63it/s]
75%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43295/57580 [00:48<00:15, 919.94it/s]
75%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43388/57580 [00:48<00:15, 893.59it/s]
76%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43478/57580 [00:48<00:15, 892.68it/s]
76%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43568/57580 [00:48<00:15, 891.92it/s]
76%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43671/57580 [00:48<00:14, 929.38it/s]
76%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43784/57580 [00:48<00:14, 984.99it/s]
76%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43883/57580 [00:49<00:14, 933.90it/s]
76%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 43980/57580 [00:49<00:14, 941.70it/s]
77%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44075/57580 [00:49<00:16, 797.93it/s]
77%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44159/57580 [00:49<00:16, 807.36it/s]
77%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44266/57580 [00:49<00:15, 876.47it/s]
77%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44358/57580 [00:49<00:14, 886.49it/s]
77%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44450/57580 [00:49<00:14, 893.50it/s]
77%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44541/57580 [00:49<00:14, 896.54it/s]
78%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44646/57580 [00:49<00:13, 938.87it/s]
78%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44763/57580 [00:50<00:12, 1003.79it/s]
78%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44865/57580 [00:50<00:13, 977.15it/s]
78%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 44964/57580 [00:50<00:14, 845.75it/s]
78%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45085/57580 [00:50<00:13, 929.07it/s]
78%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45182/57580 [00:50<00:13, 912.38it/s]
79%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45276/57580 [00:50<00:13, 913.06it/s]
79%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45370/57580 [00:50<00:13, 918.14it/s]
79%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45463/57580 [00:50<00:13, 919.80it/s]
79%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45564/57580 [00:50<00:12, 943.89it/s]
79%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45660/57580 [00:51<00:12, 937.00it/s]
79%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45755/57580 [00:51<00:13, 898.66it/s]
80%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45850/57580 [00:51<00:12, 911.00it/s]
80%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 45955/57580 [00:51<00:12, 948.72it/s]
80%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46052/57580 [00:51<00:12, 954.86it/s]
80%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46148/57580 [00:51<00:12, 898.78it/s]
80%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46255/57580 [00:51<00:11, 944.47it/s]
81%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46363/57580 [00:51<00:11, 980.82it/s]
81%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46462/57580 [00:51<00:11, 947.14it/s]
81%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46558/57580 [00:52<00:11, 921.21it/s]
81%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46651/57580 [00:52<00:12, 901.30it/s]
81%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46742/57580 [00:52<00:12, 901.36it/s]
81%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46833/57580 [00:52<00:12, 875.71it/s]
81%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 46925/57580 [00:52<00:12, 885.12it/s]
82%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47024/57580 [00:52<00:11, 912.66it/s]
82%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47116/57580 [00:52<00:11, 911.89it/s]
82%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47212/57580 [00:52<00:11, 923.14it/s]
82%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47305/57580 [00:52<00:11, 917.06it/s]
82%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47397/57580 [00:52<00:11, 915.34it/s]
82%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47489/57580 [00:53<00:12, 838.61it/s]
83%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47575/57580 [00:53<00:11, 840.18it/s]
83%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47660/57580 [00:53<00:12, 815.10it/s]
83%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47746/57580 [00:53<00:11, 825.88it/s]
83%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47830/57580 [00:53<00:11, 827.97it/s]
83%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 47915/57580 [00:53<00:11, 832.24it/s]
83%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48000/57580 [00:53<00:11, 834.69it/s]
84%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48091/57580 [00:53<00:11, 854.26it/s]
84%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48188/57580 [00:53<00:10, 885.68it/s]
84%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48296/57580 [00:54<00:09, 940.29it/s]
84%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48391/57580 [00:54<00:09, 939.60it/s]
84%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48486/57580 [00:54<00:09, 939.94it/s]
84%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48581/57580 [00:54<00:09, 927.73it/s]
85%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48674/57580 [00:54<00:09, 911.72it/s]
85%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48766/57580 [00:54<00:09, 884.86it/s]
85%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48855/57580 [00:54<00:10, 858.88it/s]
85%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 48945/57580 [00:54<00:09, 868.46it/s]
85%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49046/57580 [00:54<00:09, 906.91it/s]
85%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49140/57580 [00:54<00:09, 914.51it/s]
86%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49244/57580 [00:55<00:08, 950.53it/s]
86%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49340/57580 [00:55<00:08, 926.44it/s]
86%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49433/57580 [00:55<00:08, 919.67it/s]
86%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49526/57580 [00:55<00:09, 894.49it/s]
86%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49623/57580 [00:55<00:08, 913.97it/s]
86%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49719/57580 [00:55<00:08, 924.65it/s]
87%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49837/57580 [00:55<00:07, 995.91it/s]
87%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 49937/57580 [00:55<00:07, 994.36it/s]
87%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50037/57580 [00:55<00:08, 937.33it/s]
87%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50132/57580 [00:56<00:08, 912.30it/s]
87%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50224/57580 [00:56<00:08, 887.32it/s]
87%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50314/57580 [00:56<00:08, 889.32it/s]
88%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50413/57580 [00:56<00:07, 914.95it/s]
88%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50508/57580 [00:56<00:07, 921.67it/s]
88%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50622/57580 [00:56<00:07, 981.99it/s]
88%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50721/57580 [00:56<00:07, 952.86it/s]
88%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50817/57580 [00:56<00:07, 952.24it/s]
88%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 50913/57580 [00:56<00:07, 924.54it/s]
89%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51009/57580 [00:56<00:07, 932.32it/s]
89%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51111/57580 [00:57<00:06, 955.74it/s]
89%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51207/57580 [00:57<00:06, 954.09it/s]
89%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51303/57580 [00:57<00:06, 930.72it/s]
89%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51397/57580 [00:57<00:06, 899.47it/s]
89%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51488/57580 [00:57<00:06, 899.90it/s]
90%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51579/57580 [00:57<00:06, 900.36it/s]
90%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51670/57580 [00:57<00:06, 874.58it/s]
90%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51758/57580 [00:57<00:06, 873.40it/s]
90%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51849/57580 [00:57<00:06, 881.52it/s]
90%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 51944/57580 [00:57<00:06, 899.25it/s]
90%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52035/57580 [00:58<00:06, 873.75it/s]
91%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52123/57580 [00:58<00:06, 848.47it/s]
91%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52209/57580 [00:58<00:06, 818.25it/s]
91%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52297/57580 [00:58<00:06, 822.82it/s]
91%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52391/57580 [00:58<00:06, 853.47it/s]
91%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52478/57580 [00:58<00:05, 855.86it/s]
91%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52564/57580 [00:58<00:05, 849.46it/s]
91%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52652/57580 [00:58<00:05, 855.73it/s]
92%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52738/57580 [00:58<00:05, 854.89it/s]
92%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52824/57580 [00:59<00:05, 854.29it/s]
92%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 52912/57580 [00:59<00:05, 860.08it/s]
92%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53006/57580 [00:59<00:05, 881.58it/s]
92%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53095/57580 [00:59<00:05, 855.47it/s]
92%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53184/57580 [00:59<00:05, 863.36it/s]
93%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53276/57580 [00:59<00:04, 877.08it/s]
93%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53380/57580 [00:59<00:04, 922.54it/s]
93%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53490/57580 [00:59<00:04, 971.66it/s]
93%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53588/57580 [00:59<00:04, 971.56it/s]
93%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53686/57580 [00:59<00:04, 942.60it/s]
93%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53781/57580 [01:00<00:04, 941.43it/s]
94%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53876/57580 [01:00<00:03, 941.12it/s]
94%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 53971/57580 [01:00<00:04, 893.01it/s]
94%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54066/57580 [01:00<00:03, 906.18it/s]
94%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54158/57580 [01:00<00:03, 908.07it/s]
94%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54253/57580 [01:00<00:03, 918.05it/s]
94%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54359/57580 [01:00<00:03, 956.86it/s]
95%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54455/57580 [01:00<00:03, 949.14it/s]
95%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54551/57580 [01:00<00:03, 949.38it/s]
95%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54647/57580 [01:00<00:03, 950.39it/s]
95%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54743/57580 [01:01<00:03, 872.20it/s]
95%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54832/57580 [01:01<00:03, 851.53it/s]
95%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 54919/57580 [01:01<00:03, 831.39it/s]
96%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55003/57580 [01:01<00:03, 831.50it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55091/57580 [01:01<00:02, 842.50it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55184/57580 [01:01<00:02, 864.59it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55271/57580 [01:01<00:02, 839.05it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55363/57580 [01:01<00:02, 859.31it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55450/57580 [01:01<00:02, 859.81it/s]
96%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55544/57580 [01:02<00:02, 880.57it/s]
97%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55642/57580 [01:02<00:02, 907.43it/s]
97%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55733/57580 [01:02<00:02, 906.00it/s]
97%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55824/57580 [01:02<00:01, 900.79it/s]
97%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 55915/57580 [01:02<00:01, 880.67it/s]
97%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56004/57580 [01:02<00:01, 880.80it/s]
97%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56093/57580 [01:02<00:01, 880.89it/s]
98%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56182/57580 [01:02<00:01, 881.20it/s]
98%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56271/57580 [01:02<00:01, 880.88it/s]
98%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56368/57580 [01:02<00:01, 904.56it/s]
98%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56459/57580 [01:03<00:01, 876.17it/s]
98%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56547/57580 [01:03<00:01, 847.61it/s]
98%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56633/57580 [01:03<00:01, 822.81it/s]
98%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56716/57580 [01:03<00:01, 823.35it/s]
99%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56813/57580 [01:03<00:00, 863.26it/s]
99%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56906/57580 [01:03<00:00, 880.07it/s]
99%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 56995/57580 [01:03<00:00, 855.27it/s]
99%|ββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ | 57084/57580 [01:03<00:00, 863.22it/s]
99%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57171/57580 [01:03<00:00, 843.12it/s]
99%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57256/57580 [01:04<00:00, 818.95it/s]
100%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57341/57580 [01:04<00:00, 825.97it/s]
100%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57438/57580 [01:04<00:00, 865.13it/s]
100%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57538/57580 [01:04<00:00, 902.23it/s]
100%|βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ| 57580/57580 [01:04<00:00, 893.99it/s]
Letβs now visualize the data using matplotlib for a selected axial slice.
axial_slice = 9
fig2, ax = plt.subplots(2, 2, figsize=(6, 6),
subplot_kw={'xticks': [], 'yticks': []})
fig2.subplots_adjust(hspace=0.3, wspace=0.05)
im0 = ax.flat[0].imshow(MSD[:, :, axial_slice].T * 1000, cmap='gray',
vmin=0, vmax=2, origin='lower')
ax.flat[0].set_title('MSD (MSDKI)')
im1 = ax.flat[1].imshow(MSK[:, :, axial_slice].T, cmap='gray',
vmin=0, vmax=2, origin='lower')
ax.flat[1].set_title('MSK (MSDKI)')
im2 = ax.flat[2].imshow(MD[:, :, axial_slice].T * 1000, cmap='gray',
vmin=0, vmax=2, origin='lower')
ax.flat[2].set_title('MD (DKI)')
im3 = ax.flat[3].imshow(MK[:, :, axial_slice].T, cmap='gray',
vmin=0, vmax=2, origin='lower')
ax.flat[3].set_title('MK (DKI)')
fig2.colorbar(im0, ax=ax.flat[0])
fig2.colorbar(im1, ax=ax.flat[1])
fig2.colorbar(im2, ax=ax.flat[2])
fig2.colorbar(im3, ax=ax.flat[3])
plt.show()
fig2.savefig('MSDKI_invivo.png')
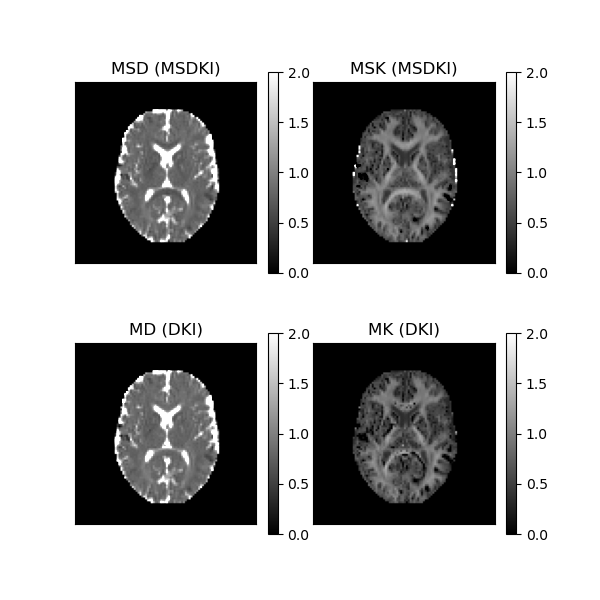
MSDKI measures (upper panels) and DKI standard measures (lower panels).
This figure shows that the contrast of in-vivo MSD and MSK maps (upper panels) are similar to the contrast of MD and MSK maps (lower panels); however, in the upper part we insure that direct contributions of fiber dispersion were removed. The upper panels also reveal that MSDKI measures are let sensitive to noise artefacts than standard DKI measures (as pointed by [NetoHe2018]), particularly one can observe that MSK maps always present positive values in brain white matter regions, while implausible negative kurtosis values are present in the MK maps in the same regions.
Relationship between MSDKI and SMT2#
As showed in [NetoHe2019], MSDKI captures the same information than the spherical mean technique (SMT) microstructural models [Kaden2016b]. In this way, the SMT model parameters can be directly computed from MSDKI. For instance, the axonal volume fraction (f), the intrisic diffusivity (di), and the microscopic anisotropy of the SMT 2-compartmental model [NetoHe2019] can be extracted using the following lines of code:
F = msdki_fit.smt2f
DI = msdki_fit.smt2di
uFA2 = msdki_fit.smt2uFA
The SMT2 model parameters extracted from MSDKI are displayed below:
fig3, ax = plt.subplots(1, 3, figsize=(9, 2.5),
subplot_kw={'xticks': [], 'yticks': []})
fig3.subplots_adjust(hspace=0.4, wspace=0.1)
im0 = ax.flat[0].imshow(F[:, :, axial_slice].T,
cmap='gray', vmin=0, vmax=1, origin='lower')
ax.flat[0].set_title('SMT2 f (MSDKI)')
im1 = ax.flat[1].imshow(DI[:, :, axial_slice].T * 1000, cmap='gray',
vmin=0, vmax=2, origin='lower')
ax.flat[1].set_title('SMT2 di (MSDKI)')
im2 = ax.flat[2].imshow(uFA2[:, :, axial_slice].T, cmap='gray',
vmin=0, vmax=1, origin='lower')
ax.flat[2].set_title('SMT2 uFA (MSDKI)')
fig3.colorbar(im0, ax=ax.flat[0])
fig3.colorbar(im1, ax=ax.flat[1])
fig3.colorbar(im2, ax=ax.flat[2])
plt.show()
fig3.savefig('MSDKI_SMT2_invivo.png')
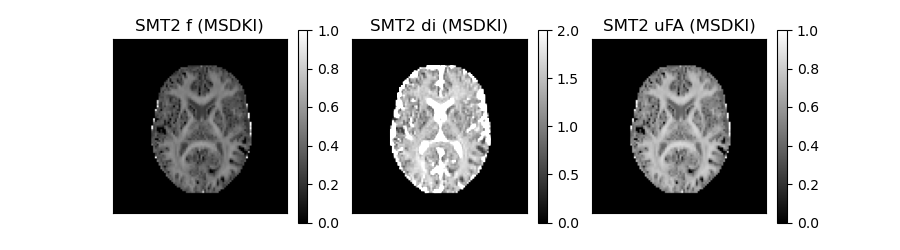
SMT2 model quantities extracted from MSDKI. From left to right, the figure shows the axonal volume fraction (f), the intrinsic diffusivity (di), and the microscopic anisotropy of the SMT 2-compartmental model [NetoHe2019].
The similar contrast of SMT2 f-parameter maps in comparison to MSK (first panel of Figure 3 vs second panel of Figure 2) confirms than MSK and F captures the same tissue information but on different scales (but rescaled to values between 0 and 1). It is important to note that SMT model parameters estimates should be used with care, because the SMT model was shown to be invalid NetoHe2019]_. For instance, although SMT2 parameter f and uFA may be a useful normalization of the degree of non-Gaussian diffusion (note than both metrics have a range between 0 and 1), these cannot be interpreted as a real biophysical estimates of axonal water fraction and tissue microscopic anisotropy.
References#
Jensen JH, Helpern JA, Ramani A, Lu H, Kaczynski K (2005). Diffusional Kurtosis Imaging: The Quantification of Non_Gaussian Water Diffusion by Means of Magnetic Resonance Imaging. Magnetic Resonance in Medicine 53: 1432-1440
Neto Henriques R, Correia MM, Nunes RG, Ferreira HA (2015). Exploring the 3D geometry of the diffusion kurtosis tensor - Impact on the development of robust tractography procedures and novel biomarkers, NeuroImage 111: 85-99
Henriques RN, 2018. Advanced Methods for Diffusion MRI Data Analysis and their Application to the Healthy Ageing Brain (Doctoral thesis). Downing College, University of Cambridge. https://doi.org/10.17863/CAM.29356
Neto Henriques R, Jespersen SN, Shemesh N (2019). Microscopic anisotropy misestimation in sphericalβmean single diffusion encoding MRI. Magnetic Resonance in Medicine (In press). doi: 10.1002/mrm.27606
Kaden E, Kelm ND, Carson RP, Does MD, Alexander DC (2016) Multi-compartment microscopic diffusion imaging. NeuroImage 139: 346-359.
Hansen, B, Jespersen, SN (2016). Data for evaluation of fast kurtosis strategies, b-value optimization and exploration of diffusion MRI contrast. Scientific Data 3: 160072 doi:10.1038/sdata.2016.72
Total running time of the script: (4 minutes 57.039 seconds)