Note
Go to the end to download the full example code
Reconstruction of the diffusion signal with the WMTI model#
DKI can also be used to derive concrete biophysical parameters by applying microstructural models to DT and KT estimated from DKI. For instance, Fieremans et al. [Fierem2011] showed that DKI can be used to estimate the contribution of hindered and restricted diffusion for well-aligned fibers - a model that was later referred to as the white matter tract integrity WMTI technique [Fierem2013]. The two tensors of WMTI can be also interpreted as the influences of intra- and extra-cellular compartments and can be used to estimate the axonal volume fraction and diffusion extra-cellular tortuosity. According to previous studies [Fierem2012] [Fierem2013], these latter measures can be used to distinguish processes of axonal loss from processes of myelin degeneration.
In this example, we show how to process a dMRI dataset using the WMTI model.
First, we import all relevant modules:
import numpy as np
import matplotlib.pyplot as plt
import dipy.reconst.dki as dki
import dipy.reconst.dki_micro as dki_micro
from dipy.core.gradients import gradient_table
from dipy.data import get_fnames
from dipy.io.gradients import read_bvals_bvecs
from dipy.io.image import load_nifti
from dipy.segment.mask import median_otsu
from scipy.ndimage import gaussian_filter
As the standard DKI, WMTI requires multi-shell data, i.e. data acquired from more than one non-zero b-value. Here, we use a fetcher to download a multi-shell dataset which was kindly provided by Hansen and Jespersen (more details about the data are provided in their paper [Hansen2016]).
fraw, fbval, fbvec, t1_fname = get_fnames('cfin_multib')
data, affine = load_nifti(fraw)
bvals, bvecs = read_bvals_bvecs(fbval, fbvec)
gtab = gradient_table(bvals, bvecs)
For comparison, this dataset is pre-processed using the same steps used in the example for reconstructing DKI (see Reconstruction of the diffusion signal with the kurtosis tensor model).
# data masking
maskdata, mask = median_otsu(data, vol_idx=[0, 1], median_radius=4, numpass=2,
autocrop=False, dilate=1)
# Smoothing
fwhm = 1.25
gauss_std = fwhm / np.sqrt(8 * np.log(2))
data_smooth = np.zeros(data.shape)
for v in range(data.shape[-1]):
data_smooth[..., v] = gaussian_filter(data[..., v], sigma=gauss_std)
The WMTI model can be defined in DIPY by instantiating the ‘KurtosisMicrostructureModel’ object in the following way:
dki_micro_model = dki_micro.KurtosisMicrostructureModel(gtab)
Before fitting this microstructural model, it is useful to indicate the regions in which this model provides meaningful information (i.e. voxels of well-aligned fibers). Following Fieremans et al. [Fieremans2011], a simple way to select this region is to generate a well-aligned fiber mask based on the values of diffusion sphericity, planarity and linearity. Here we will follow these selection criteria for a better comparison of our figures with the original article published by Fieremans et al. [Fieremans2011]. Nevertheless, it is important to note that voxels with well-aligned fibers can be selected based on other approaches such as using predefined regions of interest.
# Diffusion Tensor is computed based on the standard DKI model
dkimodel = dki.DiffusionKurtosisModel(gtab)
dkifit = dkimodel.fit(data_smooth, mask=mask)
# Initialize well aligned mask with ones
well_aligned_mask = np.ones(data.shape[:-1], dtype='bool')
# Diffusion coefficient of linearity (cl) has to be larger than 0.4, thus
# we exclude voxels with cl < 0.4.
cl = dkifit.linearity.copy()
well_aligned_mask[cl < 0.4] = False
# Diffusion coefficient of planarity (cp) has to be lower than 0.2, thus
# we exclude voxels with cp > 0.2.
cp = dkifit.planarity.copy()
well_aligned_mask[cp > 0.2] = False
# Diffusion coefficient of sphericity (cs) has to be lower than 0.35, thus
# we exclude voxels with cs > 0.35.
cs = dkifit.sphericity.copy()
well_aligned_mask[cs > 0.35] = False
# Removing nan associated with background voxels
well_aligned_mask[np.isnan(cl)] = False
well_aligned_mask[np.isnan(cp)] = False
well_aligned_mask[np.isnan(cs)] = False
0%| | 0/57580 [00:00<?, ?it/s]
0%|▏ | 71/57580 [00:00<01:21, 703.40it/s]
0%|▎ | 185/57580 [00:00<01:00, 952.73it/s]
0%|▌ | 281/57580 [00:00<01:02, 910.05it/s]
1%|▋ | 377/57580 [00:00<01:01, 925.88it/s]
1%|▉ | 470/57580 [00:00<01:03, 892.91it/s]
1%|█ | 560/57580 [00:00<01:03, 891.22it/s]
1%|█▎ | 650/57580 [00:00<01:03, 891.07it/s]
1%|█▍ | 749/57580 [00:00<01:01, 919.05it/s]
2%|█▋ | 870/57580 [00:00<00:56, 1004.56it/s]
2%|█▉ | 971/57580 [00:01<00:59, 945.80it/s]
2%|██ | 1069/57580 [00:01<00:59, 952.74it/s]
2%|██▎ | 1168/57580 [00:01<00:58, 961.12it/s]
2%|██▍ | 1271/57580 [00:01<00:57, 978.80it/s]
2%|██▋ | 1395/57580 [00:01<00:53, 1052.49it/s]
3%|██▉ | 1501/57580 [00:01<00:54, 1020.82it/s]
3%|███ | 1604/57580 [00:01<00:54, 1020.59it/s]
3%|███▎ | 1707/57580 [00:01<00:57, 964.38it/s]
3%|███▌ | 1805/57580 [00:01<00:59, 945.07it/s]
3%|███▋ | 1901/57580 [00:01<00:59, 940.58it/s]
3%|███▉ | 1996/57580 [00:02<01:00, 919.73it/s]
4%|████ | 2089/57580 [00:02<01:02, 889.48it/s]
4%|████▏ | 2181/57580 [00:02<01:01, 895.54it/s]
4%|████▍ | 2280/57580 [00:02<01:00, 920.13it/s]
4%|████▌ | 2373/57580 [00:02<01:01, 894.62it/s]
4%|████▊ | 2467/57580 [00:02<01:00, 904.03it/s]
4%|████▉ | 2558/57580 [00:02<01:00, 903.27it/s]
5%|█████▏ | 2649/57580 [00:02<01:00, 902.71it/s]
5%|█████▎ | 2740/57580 [00:02<01:02, 877.10it/s]
5%|█████▌ | 2828/57580 [00:03<01:03, 864.63it/s]
5%|█████▋ | 2915/57580 [00:03<01:03, 857.63it/s]
5%|█████▊ | 3015/57580 [00:03<01:00, 896.42it/s]
5%|██████ | 3114/57580 [00:03<00:59, 921.89it/s]
6%|██████▏ | 3207/57580 [00:03<01:01, 889.99it/s]
6%|██████▍ | 3297/57580 [00:03<01:02, 865.39it/s]
6%|██████▌ | 3390/57580 [00:03<01:01, 881.31it/s]
6%|██████▊ | 3489/57580 [00:03<00:59, 910.67it/s]
6%|██████▉ | 3584/57580 [00:03<00:58, 918.78it/s]
6%|███████▏ | 3684/57580 [00:03<00:57, 938.93it/s]
7%|███████▎ | 3800/57580 [00:04<00:53, 1000.99it/s]
7%|███████▌ | 3905/57580 [00:04<00:52, 1012.83it/s]
7%|███████▋ | 4007/57580 [00:04<00:52, 1012.70it/s]
7%|███████▉ | 4109/57580 [00:04<00:54, 982.67it/s]
7%|████████▏ | 4208/57580 [00:04<00:54, 982.13it/s]
7%|████████▍ | 4307/57580 [00:04<00:57, 926.53it/s]
8%|████████▌ | 4401/57580 [00:04<01:00, 882.13it/s]
8%|████████▋ | 4490/57580 [00:04<01:00, 882.09it/s]
8%|████████▉ | 4589/57580 [00:04<00:58, 910.93it/s]
8%|█████████ | 4681/57580 [00:05<00:58, 911.38it/s]
8%|█████████▎ | 4773/57580 [00:05<00:57, 911.89it/s]
8%|█████████▍ | 4865/57580 [00:05<00:58, 907.28it/s]
9%|█████████▋ | 4956/57580 [00:05<00:59, 879.67it/s]
9%|█████████▊ | 5045/57580 [00:05<01:01, 855.25it/s]
9%|█████████▉ | 5131/57580 [00:05<01:01, 854.47it/s]
9%|██████████▏ | 5217/57580 [00:05<01:01, 853.76it/s]
9%|██████████▎ | 5311/57580 [00:05<00:59, 876.10it/s]
9%|██████████▌ | 5399/57580 [00:05<00:59, 874.45it/s]
10%|██████████▋ | 5487/57580 [00:05<00:59, 872.82it/s]
10%|██████████▊ | 5577/57580 [00:06<00:59, 878.16it/s]
10%|███████████ | 5665/57580 [00:06<01:00, 855.62it/s]
10%|███████████▏ | 5765/57580 [00:06<00:57, 895.50it/s]
10%|███████████▍ | 5882/57580 [00:06<00:53, 973.76it/s]
10%|███████████▋ | 5983/57580 [00:06<00:52, 981.90it/s]
11%|███████████▊ | 6082/57580 [00:06<00:54, 947.30it/s]
11%|████████████ | 6178/57580 [00:06<00:54, 947.81it/s]
11%|████████████▏ | 6274/57580 [00:06<00:55, 921.67it/s]
11%|████████████▍ | 6367/57580 [00:06<00:56, 900.06it/s]
11%|████████████▌ | 6458/57580 [00:07<00:57, 895.31it/s]
11%|████████████▋ | 6548/57580 [00:07<00:57, 880.12it/s]
12%|████████████▉ | 6637/57580 [00:07<00:58, 875.71it/s]
12%|█████████████ | 6725/57580 [00:07<00:58, 868.55it/s]
12%|█████████████▎ | 6812/57580 [00:07<00:59, 846.47it/s]
12%|█████████████▍ | 6897/57580 [00:07<01:00, 840.47it/s]
12%|█████████████▌ | 6982/57580 [00:07<01:00, 840.58it/s]
12%|█████████████▋ | 7067/57580 [00:07<01:00, 840.79it/s]
12%|█████████████▉ | 7166/57580 [00:07<00:57, 882.65it/s]
13%|██████████████▏ | 7275/57580 [00:07<00:53, 940.06it/s]
13%|██████████████▎ | 7390/57580 [00:08<00:50, 999.12it/s]
13%|██████████████▌ | 7491/57580 [00:08<00:50, 999.39it/s]
13%|██████████████▊ | 7600/57580 [00:08<00:50, 999.22it/s]
13%|██████████████▉ | 7718/57580 [00:08<00:47, 1048.87it/s]
14%|███████████████ | 7824/57580 [00:08<00:47, 1043.03it/s]
14%|███████████████▎ | 7929/57580 [00:08<00:49, 1011.45it/s]
14%|███████████████▌ | 8042/57580 [00:08<00:47, 1042.19it/s]
14%|███████████████▋ | 8151/57580 [00:08<00:46, 1052.49it/s]
14%|███████████████▉ | 8257/57580 [00:08<00:48, 1021.69it/s]
15%|████████████████▎ | 8360/57580 [00:09<00:49, 992.37it/s]
15%|████████████████▍ | 8460/57580 [00:09<00:50, 964.12it/s]
15%|████████████████▋ | 8557/57580 [00:09<00:50, 963.34it/s]
15%|████████████████▊ | 8654/57580 [00:09<00:52, 935.54it/s]
15%|█████████████████ | 8748/57580 [00:09<00:52, 934.15it/s]
15%|█████████████████▏ | 8842/57580 [00:09<00:53, 907.11it/s]
16%|█████████████████▍ | 8933/57580 [00:09<00:55, 884.11it/s]
16%|█████████████████▌ | 9027/57580 [00:09<00:54, 897.37it/s]
16%|█████████████████▋ | 9117/57580 [00:09<00:54, 890.50it/s]
16%|█████████████████▉ | 9212/57580 [00:09<00:53, 905.35it/s]
16%|██████████████████ | 9316/57580 [00:10<00:51, 942.32it/s]
16%|██████████████████▎ | 9416/57580 [00:10<00:50, 956.39it/s]
17%|██████████████████▌ | 9512/57580 [00:10<00:50, 954.55it/s]
17%|██████████████████▋ | 9611/57580 [00:10<00:49, 962.63it/s]
17%|██████████████████▉ | 9715/57580 [00:10<00:48, 983.30it/s]
17%|███████████████████ | 9814/57580 [00:10<00:49, 960.02it/s]
17%|███████████████████▎ | 9911/57580 [00:10<00:51, 926.93it/s]
17%|███████████████████▎ | 10004/57580 [00:10<00:51, 925.06it/s]
18%|███████████████████▍ | 10102/57580 [00:10<00:50, 938.46it/s]
18%|███████████████████▋ | 10199/57580 [00:10<00:50, 945.58it/s]
18%|███████████████████▊ | 10294/57580 [00:11<00:50, 944.17it/s]
18%|████████████████████ | 10389/57580 [00:11<00:51, 923.95it/s]
18%|████████████████████▏ | 10482/57580 [00:11<00:51, 917.06it/s]
18%|████████████████████▍ | 10579/57580 [00:11<00:50, 929.18it/s]
19%|████████████████████▌ | 10674/57580 [00:11<00:50, 932.72it/s]
19%|████████████████████▊ | 10774/57580 [00:11<00:49, 949.97it/s]
19%|████████████████████▉ | 10874/57580 [00:11<00:48, 961.25it/s]
19%|█████████████████████▏ | 10973/57580 [00:11<00:48, 966.21it/s]
19%|█████████████████████▎ | 11081/57580 [00:11<00:46, 999.33it/s]
19%|█████████████████████▎ | 11187/57580 [00:12<00:45, 1017.33it/s]
20%|█████████████████████▌ | 11290/57580 [00:12<00:45, 1018.79it/s]
20%|█████████████████████▊ | 11392/57580 [00:12<00:45, 1009.97it/s]
20%|██████████████████████▏ | 11494/57580 [00:12<00:48, 953.54it/s]
20%|██████████████████████▎ | 11591/57580 [00:12<00:48, 955.35it/s]
20%|██████████████████████▌ | 11688/57580 [00:12<00:49, 934.90it/s]
20%|██████████████████████▋ | 11782/57580 [00:12<00:59, 772.70it/s]
21%|██████████████████████▉ | 11876/57580 [00:12<00:56, 812.81it/s]
21%|███████████████████████ | 11973/57580 [00:12<00:53, 852.82it/s]
21%|███████████████████████▎ | 12075/57580 [00:13<00:50, 895.99it/s]
21%|███████████████████████▍ | 12181/57580 [00:13<00:48, 939.35it/s]
21%|███████████████████████▋ | 12284/57580 [00:13<00:47, 961.86it/s]
22%|███████████████████████▊ | 12382/57580 [00:13<00:46, 963.97it/s]
22%|████████████████████████ | 12480/57580 [00:13<00:46, 965.43it/s]
22%|████████████████████████▏ | 12578/57580 [00:13<00:46, 966.49it/s]
22%|████████████████████████▍ | 12676/57580 [00:13<00:46, 967.01it/s]
22%|████████████████████████▋ | 12778/57580 [00:13<00:45, 978.83it/s]
22%|████████████████████████▊ | 12877/57580 [00:13<00:45, 979.36it/s]
23%|█████████████████████████ | 12976/57580 [00:13<00:46, 950.45it/s]
23%|█████████████████████████▏ | 13072/57580 [00:14<00:46, 950.14it/s]
23%|█████████████████████████▍ | 13184/57580 [00:14<00:44, 996.62it/s]
23%|█████████████████████████▌ | 13284/57580 [00:14<00:45, 971.54it/s]
23%|█████████████████████████▌ | 13393/57580 [00:14<00:44, 1002.82it/s]
23%|██████████████████████████ | 13494/57580 [00:14<00:45, 967.85it/s]
24%|██████████████████████████▏ | 13592/57580 [00:14<00:45, 969.28it/s]
24%|██████████████████████████▏ | 13703/57580 [00:14<00:43, 1007.69it/s]
24%|██████████████████████████▍ | 13807/57580 [00:14<00:43, 1015.12it/s]
24%|██████████████████████████▊ | 13909/57580 [00:14<00:44, 984.89it/s]
24%|███████████████████████████ | 14008/57580 [00:14<00:45, 956.57it/s]
24%|███████████████████████████▏ | 14104/57580 [00:15<00:46, 928.67it/s]
25%|███████████████████████████▎ | 14198/57580 [00:15<00:48, 887.67it/s]
25%|███████████████████████████▌ | 14294/57580 [00:15<00:47, 903.28it/s]
25%|███████████████████████████▊ | 14396/57580 [00:15<00:46, 933.89it/s]
25%|███████████████████████████▉ | 14498/57580 [00:15<00:45, 956.13it/s]
25%|████████████████████████████▏ | 14594/57580 [00:15<00:45, 947.91it/s]
26%|████████████████████████████▎ | 14690/57580 [00:15<00:49, 869.10it/s]
26%|████████████████████████████▌ | 14787/57580 [00:15<00:47, 892.98it/s]
26%|████████████████████████████▋ | 14892/57580 [00:15<00:45, 934.30it/s]
26%|████████████████████████████▉ | 14994/57580 [00:16<00:44, 957.11it/s]
26%|█████████████████████████████ | 15091/57580 [00:16<00:44, 958.74it/s]
26%|█████████████████████████████▎ | 15188/57580 [00:16<00:45, 932.05it/s]
27%|█████████████████████████████▍ | 15282/57580 [00:16<00:46, 905.58it/s]
27%|█████████████████████████████▋ | 15390/57580 [00:16<00:44, 953.10it/s]
27%|█████████████████████████████▊ | 15486/57580 [00:16<00:45, 925.74it/s]
27%|██████████████████████████████ | 15580/57580 [00:16<00:46, 901.70it/s]
27%|██████████████████████████████▏ | 15676/57580 [00:16<00:45, 916.09it/s]
27%|██████████████████████████████▍ | 15790/57580 [00:16<00:42, 977.79it/s]
28%|██████████████████████████████▋ | 15889/57580 [00:17<00:42, 978.71it/s]
28%|██████████████████████████████▊ | 15988/57580 [00:17<00:43, 957.12it/s]
28%|███████████████████████████████ | 16085/57580 [00:17<00:43, 952.86it/s]
28%|███████████████████████████████▏ | 16182/57580 [00:17<00:43, 954.75it/s]
28%|███████████████████████████████▏ | 16299/57580 [00:17<00:40, 1014.74it/s]
28%|███████████████████████████████▎ | 16401/57580 [00:17<00:40, 1013.73it/s]
29%|███████████████████████████████▊ | 16503/57580 [00:17<00:45, 904.75it/s]
29%|███████████████████████████████▉ | 16596/57580 [00:17<00:47, 859.06it/s]
29%|████████████████████████████████▏ | 16684/57580 [00:17<00:47, 861.29it/s]
29%|████████████████████████████████▎ | 16793/57580 [00:17<00:44, 921.89it/s]
29%|████████████████████████████████▌ | 16891/57580 [00:18<00:43, 935.23it/s]
30%|████████████████████████████████▊ | 16990/57580 [00:18<00:42, 948.11it/s]
30%|████████████████████████████████▋ | 17114/57580 [00:18<00:39, 1029.26it/s]
30%|████████████████████████████████▉ | 17221/57580 [00:18<00:38, 1038.35it/s]
30%|█████████████████████████████████ | 17326/57580 [00:18<00:38, 1038.89it/s]
30%|█████████████████████████████████▎ | 17431/57580 [00:18<00:39, 1008.37it/s]
30%|█████████████████████████████████▊ | 17533/57580 [00:18<00:40, 979.63it/s]
31%|█████████████████████████████████▉ | 17632/57580 [00:18<00:40, 980.07it/s]
31%|██████████████████████████████████▏ | 17733/57580 [00:18<00:40, 985.78it/s]
31%|██████████████████████████████████ | 17840/57580 [00:19<00:39, 1007.02it/s]
31%|██████████████████████████████████▎ | 17941/57580 [00:19<00:39, 1005.27it/s]
31%|██████████████████████████████████▊ | 18042/57580 [00:19<00:41, 941.80it/s]
32%|██████████████████████████████████▉ | 18145/57580 [00:19<00:41, 951.02it/s]
32%|███████████████████████████████████▏ | 18250/57580 [00:19<00:40, 976.75it/s]
32%|███████████████████████████████████▎ | 18349/57580 [00:19<00:41, 950.28it/s]
32%|███████████████████████████████████▌ | 18455/57580 [00:19<00:39, 978.80it/s]
32%|███████████████████████████████████▊ | 18554/57580 [00:19<00:41, 946.44it/s]
32%|███████████████████████████████████▉ | 18650/57580 [00:19<00:42, 921.77it/s]
33%|████████████████████████████████████▏ | 18743/57580 [00:20<00:45, 849.01it/s]
33%|████████████████████████████████████▎ | 18830/57580 [00:20<00:45, 849.33it/s]
33%|████████████████████████████████████▍ | 18916/57580 [00:20<00:47, 811.89it/s]
33%|████████████████████████████████████▌ | 18998/57580 [00:20<00:47, 809.22it/s]
33%|████████████████████████████████████▊ | 19099/57580 [00:20<00:44, 861.31it/s]
33%|████████████████████████████████████▉ | 19186/57580 [00:20<00:44, 862.88it/s]
33%|█████████████████████████████████████▏ | 19273/57580 [00:20<00:44, 864.20it/s]
34%|█████████████████████████████████████▎ | 19360/57580 [00:20<00:44, 863.78it/s]
34%|█████████████████████████████████████▌ | 19466/57580 [00:20<00:42, 896.99it/s]
34%|█████████████████████████████████████▋ | 19563/57580 [00:20<00:41, 915.66it/s]
34%|█████████████████████████████████████▉ | 19658/57580 [00:21<00:41, 922.92it/s]
34%|██████████████████████████████████████ | 19751/57580 [00:21<00:41, 916.60it/s]
34%|██████████████████████████████████████▎ | 19843/57580 [00:21<00:42, 887.90it/s]
35%|██████████████████████████████████████▍ | 19933/57580 [00:21<00:42, 888.77it/s]
35%|██████████████████████████████████████▌ | 20023/57580 [00:21<00:43, 869.31it/s]
35%|██████████████████████████████████████▊ | 20112/57580 [00:21<00:42, 872.59it/s]
35%|██████████████████████████████████████▉ | 20216/57580 [00:21<00:40, 918.51it/s]
35%|███████████████████████████████████████▏ | 20317/57580 [00:21<00:39, 942.27it/s]
35%|███████████████████████████████████████▍ | 20431/57580 [00:21<00:37, 997.15it/s]
36%|███████████████████████████████████████▏ | 20535/57580 [00:21<00:36, 1006.69it/s]
36%|███████████████████████████████████████▊ | 20636/57580 [00:22<00:36, 998.65it/s]
36%|███████████████████████████████████████▉ | 20736/57580 [00:22<00:40, 913.22it/s]
36%|████████████████████████████████████████▏ | 20829/57580 [00:22<00:40, 915.87it/s]
36%|████████████████████████████████████████▎ | 20922/57580 [00:22<00:39, 917.35it/s]
36%|████████████████████████████████████████▌ | 21015/57580 [00:22<00:40, 898.13it/s]
37%|████████████████████████████████████████▋ | 21111/57580 [00:22<00:39, 912.60it/s]
37%|████████████████████████████████████████▊ | 21203/57580 [00:22<00:40, 905.94it/s]
37%|█████████████████████████████████████████ | 21299/57580 [00:22<00:39, 918.76it/s]
37%|█████████████████████████████████████████▏ | 21396/57580 [00:22<00:38, 931.14it/s]
37%|█████████████████████████████████████████▍ | 21490/57580 [00:23<00:38, 931.69it/s]
37%|█████████████████████████████████████████▌ | 21584/57580 [00:23<00:38, 931.42it/s]
38%|█████████████████████████████████████████▊ | 21678/57580 [00:23<00:41, 870.04it/s]
38%|█████████████████████████████████████████▉ | 21766/57580 [00:23<00:41, 861.77it/s]
38%|██████████████████████████████████████████▏ | 21853/57580 [00:23<00:42, 837.59it/s]
38%|██████████████████████████████████████████▎ | 21938/57580 [00:23<00:43, 817.05it/s]
38%|██████████████████████████████████████████▍ | 22022/57580 [00:23<00:43, 819.13it/s]
38%|██████████████████████████████████████████▋ | 22121/57580 [00:23<00:40, 865.91it/s]
39%|██████████████████████████████████████████▊ | 22213/57580 [00:23<00:40, 878.84it/s]
39%|███████████████████████████████████████████ | 22334/57580 [00:23<00:36, 972.07it/s]
39%|██████████████████████████████████████████▉ | 22448/57580 [00:24<00:34, 1018.25it/s]
39%|███████████████████████████████████████████ | 22551/57580 [00:24<00:34, 1018.76it/s]
39%|███████████████████████████████████████████▋ | 22654/57580 [00:24<00:35, 983.95it/s]
40%|███████████████████████████████████████████▊ | 22753/57580 [00:24<00:37, 928.56it/s]
40%|████████████████████████████████████████████ | 22847/57580 [00:24<00:37, 929.50it/s]
40%|████████████████████████████████████████████▎ | 22959/57580 [00:24<00:35, 981.23it/s]
40%|████████████████████████████████████████████ | 23079/57580 [00:24<00:33, 1040.86it/s]
40%|████████████████████████████████████████████▋ | 23184/57580 [00:24<00:34, 988.99it/s]
40%|████████████████████████████████████████████▉ | 23284/57580 [00:24<00:35, 956.60it/s]
41%|█████████████████████████████████████████████ | 23381/57580 [00:25<00:36, 931.89it/s]
41%|█████████████████████████████████████████████▎ | 23485/57580 [00:25<00:35, 959.18it/s]
41%|█████████████████████████████████████████████▍ | 23585/57580 [00:25<00:35, 967.93it/s]
41%|█████████████████████████████████████████████▋ | 23683/57580 [00:25<00:34, 968.51it/s]
41%|█████████████████████████████████████████████▊ | 23784/57580 [00:25<00:34, 977.73it/s]
41%|██████████████████████████████████████████████ | 23882/57580 [00:25<00:35, 952.48it/s]
42%|██████████████████████████████████████████████▏ | 23978/57580 [00:25<00:40, 826.86it/s]
42%|██████████████████████████████████████████████▍ | 24064/57580 [00:25<00:40, 833.92it/s]
42%|██████████████████████████████████████████████▌ | 24171/57580 [00:25<00:37, 896.00it/s]
42%|██████████████████████████████████████████████▊ | 24266/57580 [00:26<00:36, 909.17it/s]
42%|██████████████████████████████████████████████▉ | 24359/57580 [00:26<00:37, 887.30it/s]
42%|███████████████████████████████████████████████▏ | 24449/57580 [00:26<00:38, 868.80it/s]
43%|███████████████████████████████████████████████▎ | 24540/57580 [00:26<00:37, 878.00it/s]
43%|███████████████████████████████████████████████▍ | 24633/57580 [00:26<00:37, 890.39it/s]
43%|███████████████████████████████████████████████▋ | 24723/57580 [00:26<00:37, 885.57it/s]
43%|███████████████████████████████████████████████▊ | 24812/57580 [00:26<00:37, 883.82it/s]
43%|████████████████████████████████████████████████ | 24912/57580 [00:26<00:35, 915.34it/s]
43%|████████████████████████████████████████████████▏ | 25004/57580 [00:26<00:35, 914.06it/s]
44%|████████████████████████████████████████████████▍ | 25096/57580 [00:26<00:35, 913.34it/s]
44%|████████████████████████████████████████████████▌ | 25195/57580 [00:27<00:34, 933.26it/s]
44%|████████████████████████████████████████████████▊ | 25289/57580 [00:27<00:34, 933.41it/s]
44%|████████████████████████████████████████████████▉ | 25383/57580 [00:27<00:35, 904.34it/s]
44%|█████████████████████████████████████████████████▏ | 25487/57580 [00:27<00:34, 922.94it/s]
44%|█████████████████████████████████████████████████▎ | 25585/57580 [00:27<00:34, 936.62it/s]
45%|█████████████████████████████████████████████████▌ | 25679/57580 [00:27<00:34, 929.01it/s]
45%|█████████████████████████████████████████████████▋ | 25784/57580 [00:27<00:33, 960.71it/s]
45%|█████████████████████████████████████████████████▉ | 25881/57580 [00:27<00:33, 932.50it/s]
45%|██████████████████████████████████████████████████ | 25975/57580 [00:27<00:34, 904.56it/s]
45%|██████████████████████████████████████████████████▎ | 26069/57580 [00:28<00:34, 912.57it/s]
45%|██████████████████████████████████████████████████▍ | 26191/57580 [00:28<00:31, 998.68it/s]
46%|██████████████████████████████████████████████████▏ | 26296/57580 [00:28<00:30, 1010.79it/s]
46%|██████████████████████████████████████████████████▉ | 26398/57580 [00:28<00:31, 987.74it/s]
46%|███████████████████████████████████████████████████ | 26498/57580 [00:28<00:32, 955.17it/s]
46%|███████████████████████████████████████████████████▎ | 26594/57580 [00:28<00:33, 926.49it/s]
46%|███████████████████████████████████████████████████▍ | 26687/57580 [00:28<00:33, 925.60it/s]
47%|███████████████████████████████████████████████████▋ | 26780/57580 [00:28<00:35, 873.93it/s]
47%|███████████████████████████████████████████████████▊ | 26869/57580 [00:28<00:37, 829.75it/s]
47%|███████████████████████████████████████████████████▉ | 26973/57580 [00:29<00:34, 885.38it/s]
47%|████████████████████████████████████████████████████▏ | 27073/57580 [00:29<00:33, 915.71it/s]
47%|████████████████████████████████████████████████████▍ | 27182/57580 [00:29<00:31, 963.57it/s]
47%|████████████████████████████████████████████████████▌ | 27283/57580 [00:29<00:31, 975.24it/s]
48%|████████████████████████████████████████████████████▊ | 27382/57580 [00:29<00:31, 964.88it/s]
48%|████████████████████████████████████████████████████▉ | 27479/57580 [00:29<00:31, 955.75it/s]
48%|█████████████████████████████████████████████████████▏ | 27575/57580 [00:29<00:31, 949.10it/s]
48%|█████████████████████████████████████████████████████▎ | 27673/57580 [00:29<00:31, 955.65it/s]
48%|█████████████████████████████████████████████████████▌ | 27783/57580 [00:29<00:29, 995.14it/s]
48%|█████████████████████████████████████████████████████▎ | 27908/57580 [00:29<00:27, 1070.27it/s]
49%|█████████████████████████████████████████████████████▌ | 28016/57580 [00:30<00:27, 1066.59it/s]
49%|█████████████████████████████████████████████████████▋ | 28123/57580 [00:30<00:28, 1033.84it/s]
49%|██████████████████████████████████████████████████████▍ | 28227/57580 [00:30<00:30, 975.52it/s]
49%|██████████████████████████████████████████████████████▌ | 28326/57580 [00:30<00:30, 949.47it/s]
49%|██████████████████████████████████████████████████████▊ | 28422/57580 [00:30<00:30, 950.08it/s]
50%|██████████████████████████████████████████████████████▉ | 28518/57580 [00:30<00:30, 946.83it/s]
50%|███████████████████████████████████████████████████████▏ | 28613/57580 [00:30<00:31, 927.00it/s]
50%|███████████████████████████████████████████████████████▎ | 28711/57580 [00:30<00:30, 939.23it/s]
50%|███████████████████████████████████████████████████████▌ | 28806/57580 [00:30<00:30, 934.16it/s]
50%|███████████████████████████████████████████████████████▋ | 28900/57580 [00:30<00:32, 881.85it/s]
50%|███████████████████████████████████████████████████████▉ | 28989/57580 [00:31<00:32, 881.96it/s]
51%|████████████████████████████████████████████████████████ | 29078/57580 [00:31<00:32, 881.59it/s]
51%|████████████████████████████████████████████████████████▏ | 29167/57580 [00:31<00:32, 864.09it/s]
51%|████████████████████████████████████████████████████████▍ | 29284/57580 [00:31<00:29, 948.16it/s]
51%|████████████████████████████████████████████████████████▏ | 29401/57580 [00:31<00:27, 1009.65it/s]
51%|████████████████████████████████████████████████████████▎ | 29503/57580 [00:31<00:27, 1009.99it/s]
51%|█████████████████████████████████████████████████████████ | 29605/57580 [00:31<00:28, 974.86it/s]
52%|█████████████████████████████████████████████████████████▎ | 29703/57580 [00:31<00:29, 945.34it/s]
52%|█████████████████████████████████████████████████████████▍ | 29798/57580 [00:31<00:30, 921.84it/s]
52%|█████████████████████████████████████████████████████████▌ | 29891/57580 [00:32<00:30, 916.17it/s]
52%|█████████████████████████████████████████████████████████▊ | 29987/57580 [00:32<00:29, 925.96it/s]
52%|██████████████████████████████████████████████████████████ | 30096/57580 [00:32<00:28, 970.70it/s]
52%|██████████████████████████████████████████████████████████▏ | 30197/57580 [00:32<00:27, 979.75it/s]
53%|██████████████████████████████████████████████████████████▍ | 30296/57580 [00:32<00:27, 980.04it/s]
53%|██████████████████████████████████████████████████████████▌ | 30401/57580 [00:32<00:27, 997.91it/s]
53%|██████████████████████████████████████████████████████████▊ | 30501/57580 [00:32<00:27, 995.52it/s]
53%|██████████████████████████████████████████████████████████▉ | 30601/57580 [00:32<00:27, 993.64it/s]
53%|███████████████████████████████████████████████████████████▏ | 30701/57580 [00:32<00:27, 992.76it/s]
53%|██████████████████████████████████████████████████████████▊ | 30804/57580 [00:32<00:26, 1001.21it/s]
54%|███████████████████████████████████████████████████████████▌ | 30905/57580 [00:33<00:27, 972.25it/s]
54%|███████████████████████████████████████████████████████████▊ | 31003/57580 [00:33<00:27, 972.17it/s]
54%|███████████████████████████████████████████████████████████▉ | 31101/57580 [00:33<00:27, 971.52it/s]
54%|███████████████████████████████████████████████████████████▋ | 31212/57580 [00:33<00:26, 1008.83it/s]
54%|███████████████████████████████████████████████████████████▊ | 31334/57580 [00:33<00:24, 1067.63it/s]
55%|████████████████████████████████████████████████████████████▌ | 31441/57580 [00:33<00:26, 998.94it/s]
55%|████████████████████████████████████████████████████████████▊ | 31542/57580 [00:33<00:27, 950.14it/s]
55%|████████████████████████████████████████████████████████████▉ | 31638/57580 [00:33<00:28, 925.09it/s]
55%|█████████████████████████████████████████████████████████████▏ | 31732/57580 [00:33<00:28, 900.26it/s]
55%|█████████████████████████████████████████████████████████████▎ | 31823/57580 [00:34<00:28, 900.82it/s]
55%|█████████████████████████████████████████████████████████████▌ | 31925/57580 [00:34<00:27, 932.66it/s]
56%|█████████████████████████████████████████████████████████████▋ | 32027/57580 [00:34<00:26, 955.04it/s]
56%|█████████████████████████████████████████████████████████████▍ | 32142/57580 [00:34<00:25, 1008.51it/s]
56%|█████████████████████████████████████████████████████████████▋ | 32267/57580 [00:34<00:23, 1075.45it/s]
56%|█████████████████████████████████████████████████████████████▊ | 32375/57580 [00:34<00:23, 1073.86it/s]
56%|██████████████████████████████████████████████████████████████ | 32483/57580 [00:34<00:24, 1017.93it/s]
57%|██████████████████████████████████████████████████████████████▎ | 32587/57580 [00:34<00:24, 1021.28it/s]
57%|███████████████████████████████████████████████████████████████ | 32690/57580 [00:34<00:25, 959.98it/s]
57%|███████████████████████████████████████████████████████████████▏ | 32787/57580 [00:35<00:27, 908.89it/s]
57%|███████████████████████████████████████████████████████████████▍ | 32892/57580 [00:35<00:26, 945.22it/s]
57%|███████████████████████████████████████████████████████████████▌ | 32988/57580 [00:35<00:27, 896.72it/s]
57%|███████████████████████████████████████████████████████████████▊ | 33079/57580 [00:35<00:28, 864.31it/s]
58%|███████████████████████████████████████████████████████████████▉ | 33172/57580 [00:35<00:28, 859.32it/s]
58%|████████████████████████████████████████████████████████████████ | 33259/57580 [00:35<00:28, 853.29it/s]
58%|████████████████████████████████████████████████████████████████▎ | 33358/57580 [00:35<00:28, 864.30it/s]
58%|████████████████████████████████████████████████████████████████▍ | 33445/57580 [00:35<00:27, 865.75it/s]
58%|████████████████████████████████████████████████████████████████▋ | 33534/57580 [00:35<00:27, 870.58it/s]
58%|████████████████████████████████████████████████████████████████▊ | 33643/57580 [00:35<00:25, 931.27it/s]
59%|█████████████████████████████████████████████████████████████████ | 33737/57580 [00:36<00:25, 930.71it/s]
59%|█████████████████████████████████████████████████████████████████▏ | 33834/57580 [00:36<00:25, 939.25it/s]
59%|█████████████████████████████████████████████████████████████████▍ | 33929/57580 [00:36<00:25, 934.74it/s]
59%|█████████████████████████████████████████████████████████████████▌ | 34023/57580 [00:36<00:25, 930.58it/s]
59%|█████████████████████████████████████████████████████████████████▊ | 34117/57580 [00:36<00:25, 906.46it/s]
59%|█████████████████████████████████████████████████████████████████▉ | 34208/57580 [00:36<00:26, 884.43it/s]
60%|██████████████████████████████████████████████████████████████████▏ | 34324/57580 [00:36<00:24, 960.38it/s]
60%|██████████████████████████████████████████████████████████████████▎ | 34421/57580 [00:36<00:24, 927.75it/s]
60%|██████████████████████████████████████████████████████████████████▌ | 34526/57580 [00:36<00:24, 959.41it/s]
60%|██████████████████████████████████████████████████████████████████▋ | 34623/57580 [00:37<00:23, 959.51it/s]
60%|██████████████████████████████████████████████████████████████████▉ | 34720/57580 [00:37<00:23, 959.96it/s]
60%|███████████████████████████████████████████████████████████████████ | 34817/57580 [00:37<00:24, 937.97it/s]
61%|███████████████████████████████████████████████████████████████████▎ | 34912/57580 [00:37<00:24, 933.74it/s]
61%|███████████████████████████████████████████████████████████████████▌ | 35019/57580 [00:37<00:23, 971.44it/s]
61%|███████████████████████████████████████████████████████████████████▋ | 35117/57580 [00:37<00:24, 922.69it/s]
61%|███████████████████████████████████████████████████████████████████▉ | 35210/57580 [00:37<00:25, 891.07it/s]
61%|████████████████████████████████████████████████████████████████████ | 35300/57580 [00:37<00:25, 866.07it/s]
61%|████████████████████████████████████████████████████████████████████▏ | 35390/57580 [00:37<00:25, 873.37it/s]
62%|████████████████████████████████████████████████████████████████████▍ | 35478/57580 [00:37<00:26, 848.92it/s]
62%|████████████████████████████████████████████████████████████████████▌ | 35578/57580 [00:38<00:24, 889.34it/s]
62%|████████████████████████████████████████████████████████████████████▊ | 35688/57580 [00:38<00:23, 947.93it/s]
62%|████████████████████████████████████████████████████████████████████▉ | 35790/57580 [00:38<00:22, 966.78it/s]
62%|█████████████████████████████████████████████████████████████████████▏ | 35888/57580 [00:38<00:22, 945.26it/s]
62%|█████████████████████████████████████████████████████████████████████▎ | 35983/57580 [00:38<00:23, 917.21it/s]
63%|█████████████████████████████████████████████████████████████████████▌ | 36076/57580 [00:38<00:23, 912.51it/s]
63%|█████████████████████████████████████████████████████████████████████▋ | 36178/57580 [00:38<00:22, 939.72it/s]
63%|█████████████████████████████████████████████████████████████████████▉ | 36273/57580 [00:38<00:23, 918.49it/s]
63%|██████████████████████████████████████████████████████████████████████ | 36369/57580 [00:38<00:22, 927.75it/s]
63%|██████████████████████████████████████████████████████████████████████▎ | 36463/57580 [00:39<00:22, 928.52it/s]
63%|██████████████████████████████████████████████████████████████████████▍ | 36558/57580 [00:39<00:22, 932.35it/s]
64%|██████████████████████████████████████████████████████████████████████▋ | 36659/57580 [00:39<00:21, 953.17it/s]
64%|██████████████████████████████████████████████████████████████████████▊ | 36755/57580 [00:39<00:21, 947.55it/s]
64%|███████████████████████████████████████████████████████████████████████ | 36863/57580 [00:39<00:21, 986.41it/s]
64%|██████████████████████████████████████████████████████████████████████▋ | 36971/57580 [00:39<00:20, 1012.34it/s]
64%|███████████████████████████████████████████████████████████████████████▍ | 37073/57580 [00:39<00:20, 981.99it/s]
65%|███████████████████████████████████████████████████████████████████████▋ | 37172/57580 [00:39<00:21, 932.03it/s]
65%|███████████████████████████████████████████████████████████████████████▏ | 37291/57580 [00:39<00:20, 1002.16it/s]
65%|███████████████████████████████████████████████████████████████████████▍ | 37411/57580 [00:39<00:19, 1055.18it/s]
65%|███████████████████████████████████████████████████████████████████████▋ | 37518/57580 [00:40<00:19, 1050.59it/s]
65%|███████████████████████████████████████████████████████████████████████▉ | 37624/57580 [00:40<00:18, 1050.34it/s]
66%|████████████████████████████████████████████████████████████████████████▋ | 37730/57580 [00:40<00:20, 991.74it/s]
66%|████████████████████████████████████████████████████████████████████████▎ | 37839/57580 [00:40<00:19, 1017.01it/s]
66%|████████████████████████████████████████████████████████████████████████▌ | 37958/57580 [00:40<00:18, 1063.25it/s]
66%|████████████████████████████████████████████████████████████████████████▋ | 38066/57580 [00:40<00:19, 1023.02it/s]
66%|█████████████████████████████████████████████████████████████████████████▌ | 38170/57580 [00:40<00:20, 929.70it/s]
66%|█████████████████████████████████████████████████████████████████████████▊ | 38265/57580 [00:40<00:20, 932.81it/s]
67%|█████████████████████████████████████████████████████████████████████████▉ | 38360/57580 [00:40<00:20, 935.68it/s]
67%|██████████████████████████████████████████████████████████████████████████▏ | 38455/57580 [00:41<00:21, 891.51it/s]
67%|██████████████████████████████████████████████████████████████████████████▎ | 38563/57580 [00:41<00:20, 941.83it/s]
67%|██████████████████████████████████████████████████████████████████████████▌ | 38659/57580 [00:41<00:20, 917.85it/s]
67%|██████████████████████████████████████████████████████████████████████████▋ | 38752/57580 [00:41<00:20, 913.45it/s]
67%|██████████████████████████████████████████████████████████████████████████▉ | 38844/57580 [00:41<00:20, 912.53it/s]
68%|███████████████████████████████████████████████████████████████████████████ | 38936/57580 [00:41<00:20, 912.70it/s]
68%|███████████████████████████████████████████████████████████████████████████▏ | 39033/57580 [00:41<00:20, 926.26it/s]
68%|███████████████████████████████████████████████████████████████████████████▍ | 39132/57580 [00:41<00:19, 942.14it/s]
68%|███████████████████████████████████████████████████████████████████████████▌ | 39227/57580 [00:41<00:19, 941.67it/s]
68%|███████████████████████████████████████████████████████████████████████████▊ | 39322/57580 [00:41<00:19, 940.51it/s]
68%|████████████████████████████████████████████████████████████████████████████ | 39427/57580 [00:42<00:18, 970.32it/s]
69%|████████████████████████████████████████████████████████████████████████████▏ | 39525/57580 [00:42<00:19, 942.70it/s]
69%|████████████████████████████████████████████████████████████████████████████▍ | 39620/57580 [00:42<00:19, 942.41it/s]
69%|████████████████████████████████████████████████████████████████████████████▌ | 39715/57580 [00:42<00:18, 941.91it/s]
69%|████████████████████████████████████████████████████████████████████████████▊ | 39828/57580 [00:42<00:17, 994.74it/s]
69%|████████████████████████████████████████████████████████████████████████████▉ | 39928/57580 [00:42<00:20, 868.94it/s]
69%|█████████████████████████████████████████████████████████████████████████████▏ | 40018/57580 [00:42<00:21, 830.15it/s]
70%|█████████████████████████████████████████████████████████████████████████████▎ | 40110/57580 [00:42<00:20, 852.46it/s]
70%|█████████████████████████████████████████████████████████████████████████████▍ | 40198/57580 [00:42<00:20, 858.44it/s]
70%|█████████████████████████████████████████████████████████████████████████████▋ | 40296/57580 [00:43<00:19, 890.72it/s]
70%|█████████████████████████████████████████████████████████████████████████████▊ | 40387/57580 [00:43<00:19, 868.82it/s]
70%|██████████████████████████████████████████████████████████████████████████████ | 40482/57580 [00:43<00:19, 889.54it/s]
70%|██████████████████████████████████████████████████████████████████████████████▏ | 40572/57580 [00:43<00:20, 839.35it/s]
71%|██████████████████████████████████████████████████████████████████████████████▍ | 40668/57580 [00:43<00:19, 870.40it/s]
71%|██████████████████████████████████████████████████████████████████████████████▌ | 40756/57580 [00:43<00:19, 870.89it/s]
71%|██████████████████████████████████████████████████████████████████████████████▋ | 40844/57580 [00:43<00:19, 871.48it/s]
71%|██████████████████████████████████████████████████████████████████████████████▉ | 40932/57580 [00:43<00:19, 871.80it/s]
71%|███████████████████████████████████████████████████████████████████████████████ | 41020/57580 [00:43<00:19, 871.53it/s]
71%|███████████████████████████████████████████████████████████████████████████████▎ | 41144/57580 [00:44<00:16, 976.84it/s]
72%|██████████████████████████████████████████████████████████████████████████████▊ | 41260/57580 [00:44<00:15, 1028.19it/s]
72%|███████████████████████████████████████████████████████████████████████████████ | 41371/57580 [00:44<00:15, 1049.13it/s]
72%|███████████████████████████████████████████████████████████████████████████████▎ | 41491/57580 [00:44<00:14, 1090.83it/s]
72%|███████████████████████████████████████████████████████████████████████████████▍ | 41606/57580 [00:44<00:14, 1105.55it/s]
72%|███████████████████████████████████████████████████████████████████████████████▋ | 41717/57580 [00:44<00:14, 1071.95it/s]
73%|████████████████████████████████████████████████████████████████████████████████▋ | 41825/57580 [00:44<00:17, 915.26it/s]
73%|████████████████████████████████████████████████████████████████████████████████▊ | 41930/57580 [00:44<00:16, 948.32it/s]
73%|█████████████████████████████████████████████████████████████████████████████████ | 42044/57580 [00:44<00:15, 998.52it/s]
73%|████████████████████████████████████████████████████████████████████████████████▌ | 42164/57580 [00:44<00:14, 1052.09it/s]
73%|████████████████████████████████████████████████████████████████████████████████▊ | 42289/57580 [00:45<00:13, 1105.11it/s]
74%|█████████████████████████████████████████████████████████████████████████████████ | 42402/57580 [00:45<00:14, 1043.02it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▏ | 42516/57580 [00:45<00:14, 1067.26it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▍ | 42625/57580 [00:45<00:14, 1047.49it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▋ | 42744/57580 [00:45<00:13, 1085.32it/s]
74%|█████████████████████████████████████████████████████████████████████████████████▊ | 42854/57580 [00:45<00:14, 1049.45it/s]
75%|██████████████████████████████████████████████████████████████████████████████████ | 42960/57580 [00:45<00:14, 1019.65it/s]
75%|███████████████████████████████████████████████████████████████████████████████████ | 43063/57580 [00:45<00:14, 991.37it/s]
75%|███████████████████████████████████████████████████████████████████████████████████▏ | 43163/57580 [00:45<00:14, 990.32it/s]
75%|███████████████████████████████████████████████████████████████████████████████████▍ | 43263/57580 [00:46<00:14, 962.84it/s]
75%|███████████████████████████████████████████████████████████████████████████████████▌ | 43361/57580 [00:46<00:14, 964.88it/s]
75%|███████████████████████████████████████████████████████████████████████████████████▊ | 43470/57580 [00:46<00:14, 998.49it/s]
76%|███████████████████████████████████████████████████████████████████████████████████▉ | 43571/57580 [00:46<00:14, 970.42it/s]
76%|████████████████████████████████████████████████████████████████████████████████████▏ | 43669/57580 [00:46<00:14, 970.67it/s]
76%|████████████████████████████████████████████████████████████████████████████████████▎ | 43767/57580 [00:46<00:14, 949.26it/s]
76%|████████████████████████████████████████████████████████████████████████████████████▌ | 43875/57580 [00:46<00:13, 984.15it/s]
76%|████████████████████████████████████████████████████████████████████████████████████ | 43986/57580 [00:46<00:13, 1017.78it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▎ | 44110/57580 [00:46<00:12, 1079.75it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▍ | 44219/57580 [00:47<00:12, 1041.99it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▋ | 44324/57580 [00:47<00:13, 1011.70it/s]
77%|████████████████████████████████████████████████████████████████████████████████████▉ | 44433/57580 [00:47<00:12, 1031.44it/s]
77%|█████████████████████████████████████████████████████████████████████████████████████ | 44537/57580 [00:47<00:12, 1030.70it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████ | 44641/57580 [00:47<00:13, 992.57it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▏ | 44741/57580 [00:47<00:13, 924.50it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▍ | 44835/57580 [00:47<00:14, 899.48it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▌ | 44927/57580 [00:47<00:14, 903.01it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▊ | 45018/57580 [00:47<00:13, 903.48it/s]
78%|██████████████████████████████████████████████████████████████████████████████████████▉ | 45109/57580 [00:47<00:13, 902.41it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▏ | 45201/57580 [00:48<00:13, 905.59it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▎ | 45292/57580 [00:48<00:13, 905.08it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▍ | 45383/57580 [00:48<00:14, 854.17it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▋ | 45470/57580 [00:48<00:14, 855.89it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▊ | 45557/57580 [00:48<00:14, 857.56it/s]
79%|███████████████████████████████████████████████████████████████████████████████████████▉ | 45644/57580 [00:48<00:14, 834.39it/s]
79%|████████████████████████████████████████████████████████████████████████████████████████▏ | 45736/57580 [00:48<00:13, 856.12it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▎ | 45830/57580 [00:48<00:13, 877.52it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▌ | 45922/57580 [00:48<00:13, 887.26it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▋ | 46016/57580 [00:49<00:12, 900.17it/s]
80%|████████████████████████████████████████████████████████████████████████████████████████▉ | 46114/57580 [00:49<00:12, 921.28it/s]
80%|█████████████████████████████████████████████████████████████████████████████████████████ | 46219/57580 [00:49<00:11, 956.43it/s]
80%|█████████████████████████████████████████████████████████████████████████████████████████▎ | 46315/57580 [00:49<00:11, 954.82it/s]
81%|█████████████████████████████████████████████████████████████████████████████████████████▍ | 46411/57580 [00:49<00:12, 926.42it/s]
81%|█████████████████████████████████████████████████████████████████████████████████████████▋ | 46512/57580 [00:49<00:11, 948.61it/s]
81%|█████████████████████████████████████████████████████████████████████████████████████████▊ | 46616/57580 [00:49<00:11, 971.77it/s]
81%|██████████████████████████████████████████████████████████████████████████████████████████ | 46714/57580 [00:49<00:11, 943.45it/s]
81%|██████████████████████████████████████████████████████████████████████████████████████████▏ | 46809/57580 [00:49<00:12, 895.85it/s]
81%|██████████████████████████████████████████████████████████████████████████████████████████▍ | 46911/57580 [00:49<00:11, 928.26it/s]
82%|██████████████████████████████████████████████████████████████████████████████████████████▌ | 47005/57580 [00:50<00:11, 923.51it/s]
82%|██████████████████████████████████████████████████████████████████████████████████████████▊ | 47103/57580 [00:50<00:11, 936.54it/s]
82%|██████████████████████████████████████████████████████████████████████████████████████████▉ | 47197/57580 [00:50<00:11, 934.81it/s]
82%|███████████████████████████████████████████████████████████████████████████████████████████▏ | 47291/57580 [00:50<00:11, 858.29it/s]
82%|███████████████████████████████████████████████████████████████████████████████████████████▎ | 47395/57580 [00:50<00:11, 906.74it/s]
82%|███████████████████████████████████████████████████████████████████████████████████████████▌ | 47487/57580 [00:50<00:11, 908.39it/s]
83%|███████████████████████████████████████████████████████████████████████████████████████████▊ | 47595/57580 [00:50<00:10, 955.60it/s]
83%|███████████████████████████████████████████████████████████████████████████████████████████▉ | 47692/57580 [00:50<00:10, 929.59it/s]
83%|████████████████████████████████████████████████████████████████████████████████████████████▏ | 47795/57580 [00:50<00:10, 955.39it/s]
83%|████████████████████████████████████████████████████████████████████████████████████████████▎ | 47892/57580 [00:51<00:10, 934.53it/s]
83%|████████████████████████████████████████████████████████████████████████████████████████████▌ | 47986/57580 [00:51<00:10, 927.74it/s]
84%|████████████████████████████████████████████████████████████████████████████████████████████▋ | 48080/57580 [00:51<00:10, 928.50it/s]
84%|████████████████████████████████████████████████████████████████████████████████████████████▊ | 48174/57580 [00:51<00:10, 929.20it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████████ | 48268/57580 [00:51<00:10, 903.01it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████████▏ | 48359/57580 [00:51<00:10, 888.15it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████████▍ | 48460/57580 [00:51<00:09, 917.32it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████████▌ | 48552/57580 [00:51<00:09, 909.62it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████████▊ | 48653/57580 [00:51<00:09, 936.29it/s]
85%|█████████████████████████████████████████████████████████████████████████████████████████████▉ | 48758/57580 [00:51<00:09, 966.38it/s]
85%|██████████████████████████████████████████████████████████████████████████████████████████████▏ | 48855/57580 [00:52<00:09, 891.37it/s]
85%|██████████████████████████████████████████████████████████████████████████████████████████████▎ | 48954/57580 [00:52<00:09, 916.97it/s]
85%|██████████████████████████████████████████████████████████████████████████████████████████████▌ | 49050/57580 [00:52<00:09, 927.46it/s]
85%|██████████████████████████████████████████████████████████████████████████████████████████████▋ | 49144/57580 [00:52<00:09, 923.66it/s]
86%|██████████████████████████████████████████████████████████████████████████████████████████████▉ | 49237/57580 [00:52<00:09, 848.71it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████ | 49324/57580 [00:52<00:09, 852.16it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████▎ | 49421/57580 [00:52<00:09, 882.86it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████▍ | 49516/57580 [00:52<00:08, 899.36it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████▋ | 49607/57580 [00:52<00:09, 879.14it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████▊ | 49700/57580 [00:53<00:08, 891.07it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████████▉ | 49793/57580 [00:53<00:08, 899.52it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████▏ | 49884/57580 [00:53<00:08, 894.60it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████▎ | 49974/57580 [00:53<00:08, 868.08it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████▌ | 50068/57580 [00:53<00:08, 885.85it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████▋ | 50162/57580 [00:53<00:08, 899.13it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████████▉ | 50253/57580 [00:53<00:08, 899.36it/s]
87%|█████████████████████████████████████████████████████████████████████████████████████████████████ | 50351/57580 [00:53<00:07, 919.97it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████████▏ | 50444/57580 [00:53<00:07, 920.07it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████████▍ | 50537/57580 [00:53<00:07, 919.59it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████████▌ | 50633/57580 [00:54<00:07, 928.84it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████████▊ | 50730/57580 [00:54<00:07, 938.61it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████████▉ | 50824/57580 [00:54<00:07, 936.53it/s]
88%|██████████████████████████████████████████████████████████████████████████████████████████████████▏ | 50938/57580 [00:54<00:06, 994.27it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████▍ | 51038/57580 [00:54<00:06, 992.81it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████▌ | 51138/57580 [00:54<00:07, 912.37it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████▊ | 51231/57580 [00:54<00:07, 887.16it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████████▉ | 51321/57580 [00:54<00:07, 845.66it/s]
89%|███████████████████████████████████████████████████████████████████████████████████████████████████ | 51412/57580 [00:54<00:07, 860.64it/s]
89%|███████████████████████████████████████████████████████████████████████████████████████████████████▎ | 51504/57580 [00:55<00:06, 874.81it/s]
90%|███████████████████████████████████████████████████████████████████████████████████████████████████▌ | 51615/57580 [00:55<00:06, 939.23it/s]
90%|███████████████████████████████████████████████████████████████████████████████████████████████████▋ | 51710/57580 [00:55<00:06, 933.90it/s]
90%|███████████████████████████████████████████████████████████████████████████████████████████████████▊ | 51804/57580 [00:55<00:06, 933.73it/s]
90%|████████████████████████████████████████████████████████████████████████████████████████████████████ | 51898/57580 [00:55<00:06, 906.36it/s]
90%|████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 51989/57580 [00:55<00:06, 900.15it/s]
90%|████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 52080/57580 [00:55<00:06, 886.64it/s]
91%|████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 52181/57580 [00:55<00:05, 920.25it/s]
91%|████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 52276/57580 [00:55<00:05, 926.41it/s]
91%|████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 52370/57580 [00:55<00:05, 928.36it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 52463/57580 [00:56<00:05, 921.45it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 52556/57580 [00:56<00:05, 894.69it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 52650/57580 [00:56<00:05, 905.18it/s]
92%|█████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 52746/57580 [00:56<00:05, 918.70it/s]
92%|█████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 52845/57580 [00:56<00:05, 937.00it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████████ | 52939/57580 [00:56<00:05, 904.24it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 53030/57580 [00:56<00:05, 856.58it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 53117/57580 [00:56<00:05, 816.53it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 53204/57580 [00:56<00:05, 829.10it/s]
93%|██████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 53292/57580 [00:57<00:05, 840.79it/s]
93%|██████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 53391/57580 [00:57<00:04, 880.75it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████████ | 53486/57580 [00:57<00:04, 897.84it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 53584/57580 [00:57<00:04, 919.58it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 53678/57580 [00:57<00:04, 923.27it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 53780/57580 [00:57<00:04, 949.42it/s]
94%|███████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 53876/57580 [00:57<00:04, 921.76it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████████ | 53975/57580 [00:57<00:03, 938.07it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 54070/57580 [00:57<00:03, 938.79it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 54165/57580 [00:57<00:03, 906.93it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 54257/57580 [00:58<00:03, 882.39it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 54357/57580 [00:58<00:03, 913.66it/s]
95%|████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 54449/57580 [00:58<00:03, 912.94it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 54547/57580 [00:58<00:03, 929.55it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 54641/57580 [00:58<00:03, 930.07it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 54735/57580 [00:58<00:03, 878.75it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 54833/57580 [00:58<00:03, 904.59it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 54928/57580 [00:58<00:02, 914.74it/s]
96%|██████████████████████████████████████████████████████████████████████████████████████████████████████████ | 55038/57580 [00:58<00:02, 965.82it/s]
96%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 55136/57580 [00:58<00:02, 966.86it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 55248/57580 [00:59<00:02, 1009.97it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 55357/57580 [00:59<00:02, 1030.23it/s]
96%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 55461/57580 [00:59<00:02, 1030.02it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 55567/57580 [00:59<00:01, 1036.17it/s]
97%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 55671/57580 [00:59<00:01, 1033.91it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 55775/57580 [00:59<00:01, 951.43it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 55872/57580 [00:59<00:01, 925.86it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 55967/57580 [00:59<00:01, 929.74it/s]
97%|████████████████████████████████████████████████████████████████████████████████████████████████████████████ | 56061/57580 [00:59<00:01, 904.43it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 56152/57580 [01:00<00:01, 855.40it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 56247/57580 [01:00<00:01, 879.42it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 56336/57580 [01:00<00:01, 880.10it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 56446/57580 [01:00<00:01, 940.29it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 56541/57580 [01:00<00:01, 940.88it/s]
98%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 56636/57580 [01:00<00:01, 919.34it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 56734/57580 [01:00<00:00, 933.19it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 56829/57580 [01:00<00:00, 934.92it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 56930/57580 [01:00<00:00, 954.38it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 57026/57580 [01:00<00:00, 920.53it/s]
99%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████ | 57126/57580 [01:01<00:00, 940.83it/s]
99%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████▎| 57221/57580 [01:01<00:00, 913.93it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████▍| 57316/57580 [01:01<00:00, 921.39it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████▋| 57421/57580 [01:01<00:00, 955.86it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████▉| 57521/57580 [01:01<00:00, 966.43it/s]
100%|███████████████████████████████████████████████████████████████████████████████████████████████████████████████| 57580/57580 [01:01<00:00, 934.79it/s]
Analogous to DKI, the data fit can be done by calling the fit
function of
the model’s object as follows:
dki_micro_fit = dki_micro_model.fit(data_smooth, mask=well_aligned_mask)
0%| | 0/465 [00:00<?, ?it/s]
22%|█████████████████████████ | 102/465 [00:00<00:00, 1006.79it/s]
45%|██████████████████████████████████████████████████▋ | 207/465 [00:00<00:00, 1023.95it/s]
67%|████████████████████████████████████████████████████████████████████████████ | 310/465 [00:00<00:00, 1021.17it/s]
89%|█████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 413/465 [00:00<00:00, 1020.00it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████████| 465/465 [00:00<00:00, 1021.03it/s]
The KurtosisMicrostructureFit object created by this fit
function can
then be used to extract model parameters such as the axonal water fraction
and diffusion hindered tortuosity:
AWF = dki_micro_fit.awf
TORT = dki_micro_fit.tortuosity
These parameters are plotted below on top of the mean kurtosis maps:
MK = dkifit.mk(0, 3)
axial_slice = 9
fig1, ax = plt.subplots(1, 2, figsize=(9, 4),
subplot_kw={'xticks': [], 'yticks': []})
AWF[AWF == 0] = np.nan
TORT[TORT == 0] = np.nan
ax[0].imshow(MK[:, :, axial_slice].T, cmap=plt.cm.gray,
interpolation='nearest', origin='lower')
im0 = ax[0].imshow(AWF[:, :, axial_slice].T, cmap=plt.cm.Reds, alpha=0.9,
vmin=0.3, vmax=0.7, interpolation='nearest', origin='lower')
fig1.colorbar(im0, ax=ax.flat[0])
ax[1].imshow(MK[:, :, axial_slice].T, cmap=plt.cm.gray,
interpolation='nearest', origin='lower')
im1 = ax[1].imshow(TORT[:, :, axial_slice].T, cmap=plt.cm.Blues, alpha=0.9,
vmin=2, vmax=6, interpolation='nearest', origin='lower')
fig1.colorbar(im1, ax=ax.flat[1])
fig1.savefig('Kurtosis_Microstructural_measures.png')
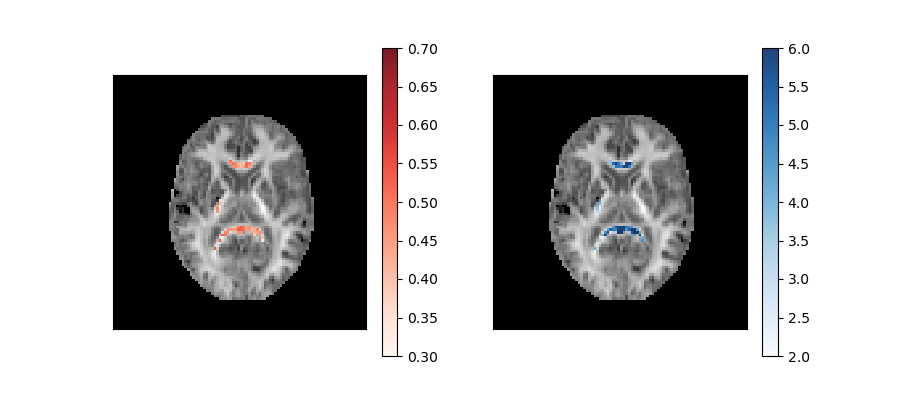
Axonal water fraction (left panel) and tortuosity (right panel) values of well-aligned fiber regions overlaid on a top of a mean kurtosis all-brain image.
References#
Fieremans E, Jensen JH, Helpern JA (2011). White matter characterization with diffusion kurtosis imaging. NeuroImage 58: 177-188
Fieremans E, Jensen JH, Helpern JA, Kim S, Grossman RI, Inglese M, Novikov DS. (2012). Diffusion distinguishes between axonal loss and demyelination in brain white matter. Proceedings of the 20th Annual Meeting of the International Society for Magnetic Resonance Medicine; Melbourne, Australia. May 5-11.
Fieremans, E., Benitez, A., Jensen, J.H., Falangola, M.F., Tabesh, A., Deardorff, R.L., Spampinato, M.V., Babb, J.S., Novikov, D.S., Ferris, S.H., Helpern, J.A., 2013. Novel white matter tract integrity metrics sensitive to Alzheimer disease progression. AJNR Am. J. Neuroradiol. 34(11), 2105-2112. doi: 10.3174/ajnr.A3553
Hansen, B, Jespersen, SN (2016). Data for evaluation of fast kurtosis strategies, b-value optimization and exploration of diffusion MRI contrast. Scientific Data 3: 160072 doi:10.1038/sdata.2016.72
Total running time of the script: (4 minutes 27.107 seconds)