Note
Go to the end to download the full example code
Particle Filtering Tractography#
Particle Filtering Tractography (PFT) [Girard2014] uses tissue partial volume estimation (PVE) to reconstruct trajectories connecting the gray matter, and not incorrectly stopping in the white matter or in the corticospinal fluid. It relies on a stopping criterion that identifies the tissue where the streamline stopped. If the streamline correctly stopped in the gray matter, the trajectory is kept. If the streamline incorrectly stopped in the white matter or in the corticospinal fluid, PFT uses anatomical information to find an alternative streamline segment to extend the trajectory. When this segment is found, the tractography continues until the streamline correctly stops in the gray matter.
PFT finds an alternative streamline segment whenever the stopping criterion returns a position classified as ‘INVALIDPOINT’.
This example is an extension of An introduction to the Probabilistic Direction Getter and Using Various Stopping Criterion for Tractography examples. We begin by loading the data, fitting a Constrained Spherical Deconvolution (CSD) reconstruction model, creating the probabilistic direction getter and defining the seeds.
from dipy.tracking.stopping_criterion import CmcStoppingCriterion
import numpy as np
from dipy.core.gradients import gradient_table
from dipy.data import get_fnames, default_sphere
from dipy.direction import ProbabilisticDirectionGetter
from dipy.io.gradients import read_bvals_bvecs
from dipy.io.image import load_nifti, load_nifti_data
from dipy.io.stateful_tractogram import Space, StatefulTractogram
from dipy.io.streamline import save_trk
from dipy.reconst.csdeconv import (ConstrainedSphericalDeconvModel,
auto_response_ssst)
from dipy.tracking.local_tracking import (LocalTracking,
ParticleFilteringTracking)
from dipy.tracking.streamline import Streamlines
from dipy.tracking import utils
from dipy.viz import window, actor, colormap, has_fury
# Enables/disables interactive visualization
interactive = False
hardi_fname, hardi_bval_fname, hardi_bvec_fname = get_fnames('stanford_hardi')
label_fname = get_fnames('stanford_labels')
f_pve_csf, f_pve_gm, f_pve_wm = get_fnames('stanford_pve_maps')
data, affine, hardi_img = load_nifti(hardi_fname, return_img=True)
labels = load_nifti_data(label_fname)
bvals, bvecs = read_bvals_bvecs(hardi_bval_fname, hardi_bvec_fname)
gtab = gradient_table(bvals, bvecs)
pve_csf_data = load_nifti_data(f_pve_csf)
pve_gm_data = load_nifti_data(f_pve_gm)
pve_wm_data, _, voxel_size = load_nifti(f_pve_wm, return_voxsize=True)
shape = labels.shape
response, ratio = auto_response_ssst(gtab, data, roi_radii=10, fa_thr=0.7)
csd_model = ConstrainedSphericalDeconvModel(gtab, response)
csd_fit = csd_model.fit(data, mask=pve_wm_data)
dg = ProbabilisticDirectionGetter.from_shcoeff(csd_fit.shm_coeff,
max_angle=20.,
sphere=default_sphere,
sh_to_pmf=True)
seed_mask = (labels == 2)
seed_mask[pve_wm_data < 0.5] = 0
seeds = utils.seeds_from_mask(seed_mask, affine, density=2)
0%| | 0/77848.12 [00:00<?, ?it/s]
0%|▏ | 159/77848.12 [00:00<00:49, 1579.53it/s]
0%|▌ | 362/77848.12 [00:00<00:42, 1836.60it/s]
1%|▉ | 688/77848.12 [00:00<00:31, 2476.77it/s]
1%|█▍ | 984/77848.12 [00:00<00:28, 2655.98it/s]
2%|█▋ | 1250/77848.12 [00:00<00:31, 2410.56it/s]
2%|██▏ | 1548/77848.12 [00:00<00:29, 2580.60it/s]
2%|██▌ | 1865/77848.12 [00:00<00:27, 2755.32it/s]
3%|██▉ | 2144/77848.12 [00:00<00:27, 2742.88it/s]
3%|███▎ | 2432/77848.12 [00:00<00:27, 2778.41it/s]
3%|███▊ | 2712/77848.12 [00:01<00:27, 2697.94it/s]
4%|████▏ | 2984/77848.12 [00:01<00:27, 2699.71it/s]
4%|████▌ | 3296/77848.12 [00:01<00:26, 2818.58it/s]
5%|████▉ | 3600/77848.12 [00:01<00:26, 2811.54it/s]
5%|█████▍ | 3887/77848.12 [00:01<00:26, 2822.03it/s]
5%|█████▊ | 4170/77848.12 [00:01<00:26, 2803.32it/s]
6%|██████▏ | 4451/77848.12 [00:01<00:28, 2571.81it/s]
6%|██████▋ | 4794/77848.12 [00:01<00:26, 2803.65it/s]
7%|███████ | 5088/77848.12 [00:01<00:25, 2836.70it/s]
7%|███████▌ | 5410/77848.12 [00:01<00:24, 2941.43it/s]
7%|███████▉ | 5713/77848.12 [00:02<00:24, 2960.71it/s]
8%|████████▎ | 6032/77848.12 [00:02<00:23, 3020.24it/s]
8%|████████▊ | 6336/77848.12 [00:02<00:24, 2896.99it/s]
9%|█████████▏ | 6628/77848.12 [00:02<00:24, 2862.69it/s]
9%|█████████▋ | 6989/77848.12 [00:02<00:23, 3070.15it/s]
9%|██████████▏ | 7304/77848.12 [00:02<00:22, 3085.69it/s]
10%|██████████▌ | 7614/77848.12 [00:02<00:22, 3082.97it/s]
10%|██████████▉ | 7924/77848.12 [00:02<00:23, 2992.35it/s]
11%|███████████▍ | 8229/77848.12 [00:02<00:23, 3001.59it/s]
11%|███████████▉ | 8569/77848.12 [00:03<00:22, 3111.44it/s]
11%|████████████▎ | 8912/77848.12 [00:03<00:21, 3200.34it/s]
12%|████████████▊ | 9233/77848.12 [00:03<00:22, 3105.61it/s]
12%|█████████████▏ | 9545/77848.12 [00:03<00:22, 3103.53it/s]
13%|█████████████▋ | 9859/77848.12 [00:03<00:21, 3106.44it/s]
13%|█████████████▉ | 10171/77848.12 [00:03<00:22, 2946.00it/s]
13%|██████████████▍ | 10468/77848.12 [00:03<00:22, 2930.33it/s]
14%|██████████████▊ | 10819/77848.12 [00:03<00:21, 3088.67it/s]
14%|███████████████▎ | 11136/77848.12 [00:03<00:21, 3104.84it/s]
15%|███████████████▋ | 11448/77848.12 [00:03<00:22, 3012.92it/s]
15%|████████████████▏ | 11751/77848.12 [00:04<00:22, 2925.18it/s]
15%|████████████████▌ | 12045/77848.12 [00:04<00:22, 2923.13it/s]
16%|█████████████████ | 12378/77848.12 [00:04<00:21, 3032.94it/s]
16%|█████████████████▍ | 12683/77848.12 [00:04<00:21, 3032.39it/s]
17%|█████████████████▊ | 12987/77848.12 [00:04<00:22, 2874.12it/s]
17%|██████████████████▏ | 13277/77848.12 [00:04<00:22, 2875.86it/s]
18%|██████████████████▊ | 13732/77848.12 [00:04<00:19, 3349.95it/s]
18%|███████████████████▎ | 14070/77848.12 [00:04<00:19, 3330.04it/s]
19%|███████████████████▊ | 14405/77848.12 [00:04<00:19, 3327.80it/s]
19%|████████████████████▎ | 14790/77848.12 [00:05<00:18, 3473.58it/s]
19%|████████████████████▊ | 15139/77848.12 [00:05<00:18, 3368.74it/s]
20%|█████████████████████▎ | 15478/77848.12 [00:05<00:18, 3366.67it/s]
20%|█████████████████████▋ | 15816/77848.12 [00:05<00:19, 3193.01it/s]
21%|██████████████████████▏ | 16138/77848.12 [00:05<00:19, 3088.24it/s]
21%|██████████████████████▌ | 16449/77848.12 [00:05<00:20, 3004.48it/s]
22%|███████████████████████ | 16751/77848.12 [00:05<00:20, 2920.27it/s]
22%|███████████████████████▍ | 17083/77848.12 [00:05<00:20, 3023.03it/s]
22%|████████████████████████ | 17476/77848.12 [00:05<00:18, 3275.70it/s]
23%|████████████████████████▍ | 17806/77848.12 [00:05<00:18, 3183.40it/s]
23%|█████████████████████████ | 18215/77848.12 [00:06<00:17, 3433.52it/s]
24%|█████████████████████████▌ | 18561/77848.12 [00:06<00:17, 3434.81it/s]
24%|██████████████████████████ | 18957/77848.12 [00:06<00:16, 3581.09it/s]
25%|██████████████████████████▌ | 19317/77848.12 [00:06<00:16, 3444.16it/s]
25%|███████████████████████████ | 19664/77848.12 [00:06<00:19, 3024.95it/s]
26%|███████████████████████████▍ | 19977/77848.12 [00:06<00:19, 2900.17it/s]
26%|███████████████████████████▉ | 20310/77848.12 [00:06<00:19, 3008.93it/s]
26%|████████████████████████████▎ | 20619/77848.12 [00:06<00:18, 3026.17it/s]
27%|████████████████████████████▊ | 20941/77848.12 [00:06<00:18, 3073.35it/s]
27%|█████████████████████████████▏ | 21267/77848.12 [00:07<00:18, 3119.94it/s]
28%|█████████████████████████████▊ | 21701/77848.12 [00:07<00:16, 3463.47it/s]
28%|██████████████████████████████▎ | 22051/77848.12 [00:07<00:16, 3445.06it/s]
29%|██████████████████████████████▊ | 22398/77848.12 [00:07<00:16, 3444.17it/s]
29%|███████████████████████████████▎ | 22744/77848.12 [00:07<00:16, 3441.31it/s]
30%|███████████████████████████████▋ | 23090/77848.12 [00:07<00:15, 3439.90it/s]
30%|████████████████████████████████▏ | 23435/77848.12 [00:07<00:16, 3335.21it/s]
31%|████████████████████████████████▋ | 23770/77848.12 [00:07<00:16, 3330.88it/s]
31%|█████████████████████████████████▏ | 24104/77848.12 [00:07<00:16, 3324.35it/s]
31%|█████████████████████████████████▌ | 24437/77848.12 [00:08<00:16, 3318.41it/s]
32%|██████████████████████████████████ | 24774/77848.12 [00:08<00:15, 3325.32it/s]
32%|██████████████████████████████████▌ | 25107/77848.12 [00:08<00:15, 3317.29it/s]
33%|██████████████████████████████████▉ | 25439/77848.12 [00:08<00:16, 3212.52it/s]
33%|███████████████████████████████████▍ | 25762/77848.12 [00:08<00:17, 3032.60it/s]
34%|███████████████████████████████████▊ | 26081/77848.12 [00:08<00:16, 3070.23it/s]
34%|████████████████████████████████████▎ | 26390/77848.12 [00:08<00:16, 3070.90it/s]
34%|████████████████████████████████████▋ | 26734/77848.12 [00:08<00:16, 3169.81it/s]
35%|█████████████████████████████████████▏ | 27057/77848.12 [00:08<00:15, 3180.62it/s]
35%|█████████████████████████████████████▋ | 27391/77848.12 [00:08<00:15, 3219.65it/s]
36%|██████████████████████████████████████ | 27726/77848.12 [00:09<00:15, 3250.74it/s]
36%|██████████████████████████████████████▌ | 28071/77848.12 [00:09<00:15, 3299.98it/s]
37%|███████████████████████████████████████ | 28455/77848.12 [00:09<00:14, 3452.33it/s]
37%|███████████████████████████████████████▌ | 28801/77848.12 [00:09<00:14, 3344.60it/s]
37%|████████████████████████████████████████ | 29137/77848.12 [00:09<00:15, 3067.27it/s]
38%|████████████████████████████████████████▍ | 29449/77848.12 [00:09<00:16, 2992.16it/s]
38%|████████████████████████████████████████▉ | 29752/77848.12 [00:09<00:16, 2840.05it/s]
39%|█████████████████████████████████████████▎ | 30058/77848.12 [00:09<00:16, 2893.73it/s]
39%|█████████████████████████████████████████▋ | 30350/77848.12 [00:09<00:17, 2741.82it/s]
39%|██████████████████████████████████████████▏ | 30656/77848.12 [00:10<00:16, 2824.03it/s]
40%|██████████████████████████████████████████▌ | 30965/77848.12 [00:10<00:16, 2890.60it/s]
40%|███████████████████████████████████████████ | 31301/77848.12 [00:10<00:15, 3017.36it/s]
41%|███████████████████████████████████████████▍ | 31616/77848.12 [00:10<00:15, 3053.45it/s]
41%|███████████████████████████████████████████▉ | 31942/77848.12 [00:10<00:14, 3109.60it/s]
41%|████████████████████████████████████████████▎ | 32279/77848.12 [00:10<00:14, 3177.69it/s]
42%|████████████████████████████████████████████▊ | 32600/77848.12 [00:10<00:14, 3178.13it/s]
42%|█████████████████████████████████████████████▎ | 32961/77848.12 [00:10<00:13, 3297.63it/s]
43%|█████████████████████████████████████████████▊ | 33292/77848.12 [00:10<00:14, 3127.10it/s]
43%|██████████████████████████████████████████████▏ | 33637/77848.12 [00:10<00:13, 3212.41it/s]
44%|██████████████████████████████████████████████▋ | 33996/77848.12 [00:11<00:13, 3313.98it/s]
44%|███████████████████████████████████████████████▏ | 34329/77848.12 [00:11<00:13, 3292.80it/s]
45%|███████████████████████████████████████████████▋ | 34667/77848.12 [00:11<00:13, 3309.04it/s]
45%|████████████████████████████████████████████████ | 34999/77848.12 [00:11<00:13, 3119.58it/s]
45%|████████████████████████████████████████████████▌ | 35314/77848.12 [00:11<00:14, 3032.33it/s]
46%|████████████████████████████████████████████████▉ | 35620/77848.12 [00:11<00:14, 2948.27it/s]
46%|█████████████████████████████████████████████████▎ | 35920/77848.12 [00:11<00:14, 2956.04it/s]
47%|█████████████████████████████████████████████████▊ | 36238/77848.12 [00:11<00:13, 3012.80it/s]
47%|██████████████████████████████████████████████████▏ | 36551/77848.12 [00:11<00:13, 3041.22it/s]
47%|██████████████████████████████████████████████████▋ | 36892/77848.12 [00:12<00:13, 3144.56it/s]
48%|███████████████████████████████████████████████████▏ | 37208/77848.12 [00:12<00:13, 3051.09it/s]
48%|███████████████████████████████████████████████████▋ | 37648/77848.12 [00:12<00:11, 3433.04it/s]
49%|████████████████████████████████████████████████████▏ | 37994/77848.12 [00:12<00:12, 3261.68it/s]
49%|████████████████████████████████████████████████████▋ | 38323/77848.12 [00:12<00:12, 3245.90it/s]
50%|█████████████████████████████████████████████████████ | 38650/77848.12 [00:12<00:12, 3172.20it/s]
50%|█████████████████████████████████████████████████████▌ | 38969/77848.12 [00:12<00:12, 3170.45it/s]
50%|██████████████████████████████████████████████████████ | 39288/77848.12 [00:12<00:12, 3149.48it/s]
51%|██████████████████████████████████████████████████████▍ | 39604/77848.12 [00:12<00:12, 2971.41it/s]
51%|██████████████████████████████████████████████████████▊ | 39908/77848.12 [00:12<00:12, 2983.11it/s]
52%|███████████████████████████████████████████████████████▎ | 40208/77848.12 [00:13<00:12, 2981.90it/s]
52%|███████████████████████████████████████████████████████▋ | 40508/77848.12 [00:13<00:12, 2980.17it/s]
52%|████████████████████████████████████████████████████████ | 40807/77848.12 [00:13<00:12, 2976.49it/s]
53%|████████████████████████████████████████████████████████▌ | 41109/77848.12 [00:13<00:12, 2981.98it/s]
53%|█████████████████████████████████████████████████████████ | 41505/77848.12 [00:13<00:11, 3261.49it/s]
54%|█████████████████████████████████████████████████████████▌ | 41866/77848.12 [00:13<00:10, 3357.16it/s]
54%|██████████████████████████████████████████████████████████ | 42203/77848.12 [00:13<00:10, 3353.11it/s]
55%|██████████████████████████████████████████████████████████▍ | 42539/77848.12 [00:13<00:10, 3246.73it/s]
55%|██████████████████████████████████████████████████████████▉ | 42865/77848.12 [00:13<00:10, 3241.81it/s]
55%|███████████████████████████████████████████████████████████▎ | 43190/77848.12 [00:14<00:11, 3143.74it/s]
56%|███████████████████████████████████████████████████████████▊ | 43506/77848.12 [00:14<00:11, 2906.32it/s]
56%|████████████████████████████████████████████████████████████▎ | 43897/77848.12 [00:14<00:10, 3177.76it/s]
57%|████████████████████████████████████████████████████████████▊ | 44266/77848.12 [00:14<00:10, 3315.83it/s]
57%|█████████████████████████████████████████████████████████████▎ | 44602/77848.12 [00:14<00:10, 3173.01it/s]
58%|█████████████████████████████████████████████████████████████▋ | 44924/77848.12 [00:14<00:11, 2969.34it/s]
58%|██████████████████████████████████████████████████████████████▏ | 45226/77848.12 [00:14<00:12, 2683.19it/s]
58%|██████████████████████████████████████████████████████████████▌ | 45507/77848.12 [00:14<00:11, 2711.08it/s]
59%|██████████████████████████████████████████████████████████████▉ | 45826/77848.12 [00:14<00:11, 2835.73it/s]
59%|███████████████████████████████████████████████████████████████▍ | 46147/77848.12 [00:15<00:10, 2932.33it/s]
60%|███████████████████████████████████████████████████████████████▉ | 46497/77848.12 [00:15<00:10, 3089.15it/s]
60%|████████████████████████████████████████████████████████████████▎ | 46810/77848.12 [00:15<00:10, 2941.80it/s]
61%|████████████████████████████████████████████████████████████████▋ | 47108/77848.12 [00:15<00:10, 2931.49it/s]
61%|█████████████████████████████████████████████████████████████████▏ | 47404/77848.12 [00:15<00:11, 2701.35it/s]
61%|█████████████████████████████████████████████████████████████████▌ | 47679/77848.12 [00:15<00:11, 2638.64it/s]
62%|█████████████████████████████████████████████████████████████████▉ | 47946/77848.12 [00:15<00:11, 2643.26it/s]
62%|██████████████████████████████████████████████████████████████████▎ | 48242/77848.12 [00:15<00:10, 2723.82it/s]
62%|██████████████████████████████████████████████████████████████████▊ | 48571/77848.12 [00:15<00:10, 2879.70it/s]
63%|███████████████████████████████████████████████████████████████████▏ | 48896/77848.12 [00:15<00:09, 2981.92it/s]
63%|███████████████████████████████████████████████████████████████████▋ | 49275/77848.12 [00:16<00:08, 3210.41it/s]
64%|████████████████████████████████████████████████████████████████████▏ | 49605/77848.12 [00:16<00:08, 3229.29it/s]
64%|████████████████████████████████████████████████████████████████████▋ | 49930/77848.12 [00:16<00:08, 3227.11it/s]
65%|█████████████████████████████████████████████████████████████████████ | 50254/77848.12 [00:16<00:09, 2975.74it/s]
65%|█████████████████████████████████████████████████████████████████████▍ | 50556/77848.12 [00:16<00:09, 2965.44it/s]
65%|█████████████████████████████████████████████████████████████████████▉ | 50856/77848.12 [00:16<00:09, 2902.75it/s]
66%|██████████████████████████████████████████████████████████████████████▎ | 51172/77848.12 [00:16<00:08, 2968.52it/s]
66%|██████████████████████████████████████████████████████████████████████▋ | 51471/77848.12 [00:16<00:08, 2948.78it/s]
67%|███████████████████████████████████████████████████████████████████████▏ | 51772/77848.12 [00:16<00:08, 2960.33it/s]
67%|███████████████████████████████████████████████████████████████████████▌ | 52098/77848.12 [00:17<00:08, 3038.93it/s]
67%|████████████████████████████████████████████████████████████████████████ | 52458/77848.12 [00:17<00:07, 3196.63it/s]
68%|████████████████████████████████████████████████████████████████████████▌ | 52779/77848.12 [00:17<00:08, 3012.55it/s]
68%|████████████████████████████████████████████████████████████████████████▉ | 53083/77848.12 [00:17<00:08, 3014.57it/s]
69%|█████████████████████████████████████████████████████████████████████████▍ | 53387/77848.12 [00:17<00:08, 2813.55it/s]
69%|█████████████████████████████████████████████████████████████████████████▊ | 53672/77848.12 [00:17<00:08, 2774.07it/s]
69%|██████████████████████████████████████████████████████████████████████████▏ | 53990/77848.12 [00:17<00:08, 2882.72it/s]
70%|██████████████████████████████████████████████████████████████████████████▊ | 54425/77848.12 [00:17<00:07, 3291.35it/s]
70%|███████████████████████████████████████████████████████████████████████████▎ | 54762/77848.12 [00:17<00:06, 3305.54it/s]
71%|███████████████████████████████████████████████████████████████████████████▋ | 55095/77848.12 [00:18<00:07, 3211.57it/s]
71%|████████████████████████████████████████████████████████████████████████████▏ | 55419/77848.12 [00:18<00:06, 3214.63it/s]
72%|████████████████████████████████████████████████████████████████████████████▌ | 55742/77848.12 [00:18<00:06, 3213.60it/s]
72%|█████████████████████████████████████████████████████████████████████████████ | 56065/77848.12 [00:18<00:06, 3121.05it/s]
72%|█████████████████████████████████████████████████████████████████████████████▍ | 56379/77848.12 [00:18<00:07, 2957.42it/s]
73%|█████████████████████████████████████████████████████████████████████████████▉ | 56678/77848.12 [00:18<00:07, 2952.56it/s]
73%|██████████████████████████████████████████████████████████████████████████████▍ | 57039/77848.12 [00:18<00:06, 3132.20it/s]
74%|██████████████████████████████████████████████████████████████████████████████▊ | 57355/77848.12 [00:18<00:06, 2959.01it/s]
74%|███████████████████████████████████████████████████████████████████████████████▎ | 57668/77848.12 [00:18<00:06, 2998.17it/s]
74%|███████████████████████████████████████████████████████████████████████████████▋ | 57979/77848.12 [00:18<00:06, 3021.17it/s]
75%|████████████████████████████████████████████████████████████████████████████████ | 58294/77848.12 [00:19<00:06, 3050.24it/s]
75%|████████████████████████████████████████████████████████████████████████████████▌ | 58604/77848.12 [00:19<00:06, 3057.05it/s]
76%|████████████████████████████████████████████████████████████████████████████████▉ | 58925/77848.12 [00:19<00:06, 3094.19it/s]
76%|█████████████████████████████████████████████████████████████████████████████████▍ | 59256/77848.12 [00:19<00:05, 3149.97it/s]
77%|█████████████████████████████████████████████████████████████████████████████████▉ | 59572/77848.12 [00:19<00:05, 3053.03it/s]
77%|██████████████████████████████████████████████████████████████████████████████████▎ | 59879/77848.12 [00:19<00:05, 3050.68it/s]
77%|██████████████████████████████████████████████████████████████████████████████████▋ | 60185/77848.12 [00:19<00:05, 2959.75it/s]
78%|███████████████████████████████████████████████████████████████████████████████████▏ | 60486/77848.12 [00:19<00:05, 2962.98it/s]
78%|███████████████████████████████████████████████████████████████████████████████████▌ | 60783/77848.12 [00:19<00:05, 2956.69it/s]
79%|████████████████████████████████████████████████████████████████████████████████████ | 61140/77848.12 [00:19<00:05, 3128.65it/s]
79%|████████████████████████████████████████████████████████████████████████████████████▍ | 61454/77848.12 [00:20<00:05, 3123.39it/s]
79%|████████████████████████████████████████████████████████████████████████████████████▉ | 61767/77848.12 [00:20<00:05, 3118.03it/s]
80%|█████████████████████████████████████████████████████████████████████████████████████▎ | 62080/77848.12 [00:20<00:05, 2939.11it/s]
80%|█████████████████████████████████████████████████████████████████████████████████████▋ | 62377/77848.12 [00:20<00:05, 2782.18it/s]
80%|██████████████████████████████████████████████████████████████████████████████████████▏ | 62665/77848.12 [00:20<00:05, 2801.74it/s]
81%|██████████████████████████████████████████████████████████████████████████████████████▌ | 62948/77848.12 [00:20<00:05, 2801.28it/s]
81%|██████████████████████████████████████████████████████████████████████████████████████▉ | 63270/77848.12 [00:20<00:05, 2914.12it/s]
82%|███████████████████████████████████████████████████████████████████████████████████████▎ | 63563/77848.12 [00:20<00:04, 2911.72it/s]
82%|███████████████████████████████████████████████████████████████████████████████████████▊ | 63883/77848.12 [00:20<00:04, 2988.05it/s]
82%|████████████████████████████████████████████████████████████████████████████████████████▏ | 64183/77848.12 [00:21<00:04, 2983.07it/s]
83%|████████████████████████████████████████████████████████████████████████████████████████▊ | 64604/77848.12 [00:21<00:03, 3338.02it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████▍ | 65042/77848.12 [00:21<00:03, 3636.45it/s]
84%|█████████████████████████████████████████████████████████████████████████████████████████▉ | 65407/77848.12 [00:21<00:03, 3630.99it/s]
84%|██████████████████████████████████████████████████████████████████████████████████████████▍ | 65771/77848.12 [00:21<00:03, 3518.90it/s]
85%|██████████████████████████████████████████████████████████████████████████████████████████▉ | 66124/77848.12 [00:21<00:03, 3433.40it/s]
85%|███████████████████████████████████████████████████████████████████████████████████████████▍ | 66497/77848.12 [00:21<00:03, 3509.75it/s]
86%|███████████████████████████████████████████████████████████████████████████████████████████▉ | 66849/77848.12 [00:21<00:03, 3291.04it/s]
86%|████████████████████████████████████████████████████████████████████████████████████████████▎ | 67182/77848.12 [00:21<00:03, 3294.84it/s]
87%|████████████████████████████████████████████████████████████████████████████████████████████▊ | 67541/77848.12 [00:21<00:03, 3369.16it/s]
87%|█████████████████████████████████████████████████████████████████████████████████████████████▎ | 67880/77848.12 [00:22<00:02, 3366.86it/s]
88%|█████████████████████████████████████████████████████████████████████████████████████████████▊ | 68218/77848.12 [00:22<00:02, 3362.47it/s]
88%|██████████████████████████████████████████████████████████████████████████████████████████████▎ | 68626/77848.12 [00:22<00:02, 3563.13it/s]
89%|██████████████████████████████████████████████████████████████████████████████████████████████▊ | 68984/77848.12 [00:22<00:02, 3454.97it/s]
89%|███████████████████████████████████████████████████████████████████████████████████████████████▎ | 69331/77848.12 [00:22<00:02, 3267.30it/s]
89%|███████████████████████████████████████████████████████████████████████████████████████████████▋ | 69661/77848.12 [00:22<00:02, 3197.12it/s]
90%|████████████████████████████████████████████████████████████████████████████████████████████████▏ | 69983/77848.12 [00:22<00:02, 3109.64it/s]
90%|████████████████████████████████████████████████████████████████████████████████████████████████▌ | 70296/77848.12 [00:22<00:02, 3008.70it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████ | 70598/77848.12 [00:22<00:02, 2923.21it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████▍ | 70892/77848.12 [00:23<00:02, 2922.75it/s]
91%|█████████████████████████████████████████████████████████████████████████████████████████████████▊ | 71185/77848.12 [00:23<00:02, 2837.28it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████▏ | 71470/77848.12 [00:23<00:02, 2770.74it/s]
92%|██████████████████████████████████████████████████████████████████████████████████████████████████▋ | 71820/77848.12 [00:23<00:02, 2970.26it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████▏ | 72119/77848.12 [00:23<00:01, 2950.60it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████▌ | 72415/77848.12 [00:23<00:01, 2947.07it/s]
93%|███████████████████████████████████████████████████████████████████████████████████████████████████▉ | 72715/77848.12 [00:23<00:01, 2957.04it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 73029/77848.12 [00:23<00:01, 3003.66it/s]
94%|████████████████████████████████████████████████████████████████████████████████████████████████████▊ | 73381/77848.12 [00:23<00:01, 3149.88it/s]
95%|█████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 73856/77848.12 [00:24<00:01, 3612.08it/s]
95%|██████████████████████████████████████████████████████████████████████████████████████████████████████ | 74218/77848.12 [00:24<00:01, 3238.30it/s]
96%|██████████████████████████████████████████████████████████████████████████████████████████████████████▍ | 74550/77848.12 [00:24<00:01, 3167.43it/s]
96%|██████████████████████████████████████████████████████████████████████████████████████████████████████▉ | 74872/77848.12 [00:24<00:00, 3087.17it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████▎ | 75185/77848.12 [00:24<00:00, 2851.88it/s]
97%|███████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 75476/77848.12 [00:24<00:00, 2861.18it/s]
97%|████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 75802/77848.12 [00:24<00:00, 2964.15it/s]
98%|████████████████████████████████████████████████████████████████████████████████████████████████████████▌ | 76118/77848.12 [00:24<00:00, 3014.26it/s]
98%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▏ | 76503/77848.12 [00:24<00:00, 3246.92it/s]
99%|█████████████████████████████████████████████████████████████████████████████████████████████████████████▋ | 76922/77848.12 [00:24<00:00, 3510.76it/s]
99%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▏| 77276/77848.12 [00:25<00:00, 3391.35it/s]
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████▊| 77673/77848.12 [00:25<00:00, 3550.15it/s]
78031it [00:25, 3354.10it/s]
78370it [00:25, 3093.64it/s]
78685it [00:25, 3020.06it/s]
78991it [00:25, 2865.33it/s]
79325it [00:25, 2984.91it/s]
79658it [00:25, 3074.01it/s]
79984it [00:25, 3120.46it/s]
80299it [00:26, 3124.89it/s]
80614it [00:26, 3127.64it/s]
80928it [00:26, 3124.12it/s]
81242it [00:26, 2716.95it/s]
81524it [00:26, 2725.61it/s]
81860it [00:26, 2848.87it/s]
82175it [00:26, 2925.55it/s]
82515it [00:26, 3053.91it/s]
82825it [00:26, 3042.08it/s]
83145it [00:27, 3080.90it/s]
83465it [00:27, 3108.33it/s]
83809it [00:27, 3197.14it/s]
84146it [00:27, 3240.21it/s]
84471it [00:27, 3064.41it/s]
84780it [00:27, 2873.59it/s]
85071it [00:27, 2875.82it/s]
85362it [00:27, 2799.10it/s]
85644it [00:27, 2721.23it/s]
85935it [00:28, 2768.19it/s]
86220it [00:28, 2784.89it/s]
86545it [00:28, 2912.18it/s]
86864it [00:28, 2986.45it/s]
87164it [00:28, 2983.06it/s]
87463it [00:28, 2979.24it/s]
87762it [00:28, 2891.27it/s]
88109it [00:28, 3053.22it/s]
88557it [00:28, 3463.34it/s]
88905it [00:28, 3265.84it/s]
89235it [00:29, 3266.04it/s]
89564it [00:29, 3264.73it/s]
89892it [00:29, 2999.72it/s]
90197it [00:29, 2930.95it/s]
90494it [00:29, 2928.95it/s]
90790it [00:29, 2774.99it/s]
91116it [00:29, 2903.72it/s]
91410it [00:29, 2907.37it/s]
91703it [00:29, 2907.81it/s]
91996it [00:30, 2908.03it/s]
92288it [00:30, 2741.80it/s]
92614it [00:30, 2882.62it/s]
92909it [00:30, 2894.88it/s]
93201it [00:30, 2894.29it/s]
93492it [00:30, 2749.35it/s]
93815it [00:30, 2877.62it/s]
94105it [00:30, 2779.79it/s]
94385it [00:30, 2642.53it/s]
94652it [00:31, 2557.89it/s]
94910it [00:31, 2488.62it/s]
95171it [00:31, 2517.11it/s]
95424it [00:31, 2459.46it/s]
95671it [00:31, 2360.39it/s]
95927it [00:31, 2370.99it/s]
96165it [00:31, 2354.45it/s]
96440it [00:31, 2460.78it/s]
96687it [00:31, 2457.74it/s]
96934it [00:31, 2385.35it/s]
96989it [00:32, 3026.70it/s]
CMC/ACT Stopping Criterion#
Continuous map criterion (CMC) [Girard2014] and Anatomically-constrained tractography (ACT) [Smith2012] both uses PVEs information from anatomical images to determine when the tractography stops. Both stopping criterion use a trilinear interpolation at the tracking position. CMC stopping criterion uses a probability derived from the PVE maps to determine if the streamline reaches a ‘valid’ or ‘invalid’ region. ACT uses a fixed threshold on the PVE maps. Both stopping criterion can be used in conjunction with PFT. In this example, we used CMC.
voxel_size = np.average(voxel_size[1:4])
step_size = 0.2
cmc_criterion = CmcStoppingCriterion.from_pve(pve_wm_data,
pve_gm_data,
pve_csf_data,
step_size=step_size,
average_voxel_size=voxel_size)
Particle Filtering Tractography#
pft_back_tracking_dist is the distance in mm to backtrack when the tractography incorrectly stops in the WM or CSF. pft_front_tracking_dist is the distance in mm to track after the stopping event using PFT.
The particle_count parameter is the number of samples used in the particle filtering algorithm.
min_wm_pve_before_stopping controls when the tracking can stop in the GM. The tractography must reaches a position where WM_pve >= min_wm_pve_before_stopping before stopping in the GM. As long as the condition is not reached and WM_pve > 0, the tractography will continue. It is particularlyusefull to set this parameter > 0.5 when seeding tractography at the WM-GM interface or in the sub-cortical GM. It allows streamlines to exit the seeding voxels until they reach the deep white matter where WM_pve > min_wm_pve_before_stopping.
pft_streamline_gen = ParticleFilteringTracking(dg,
cmc_criterion,
seeds,
affine,
max_cross=1,
step_size=step_size,
maxlen=1000,
pft_back_tracking_dist=2,
pft_front_tracking_dist=1,
particle_count=15,
return_all=False,
min_wm_pve_before_stopping=1)
streamlines = Streamlines(pft_streamline_gen)
sft = StatefulTractogram(streamlines, hardi_img, Space.RASMM)
save_trk(sft, "tractogram_pft.trk")
if has_fury:
scene = window.Scene()
scene.add(actor.line(streamlines, colormap.line_colors(streamlines)))
window.record(scene, out_path='tractogram_pft.png',
size=(800, 800))
if interactive:
window.show(scene)
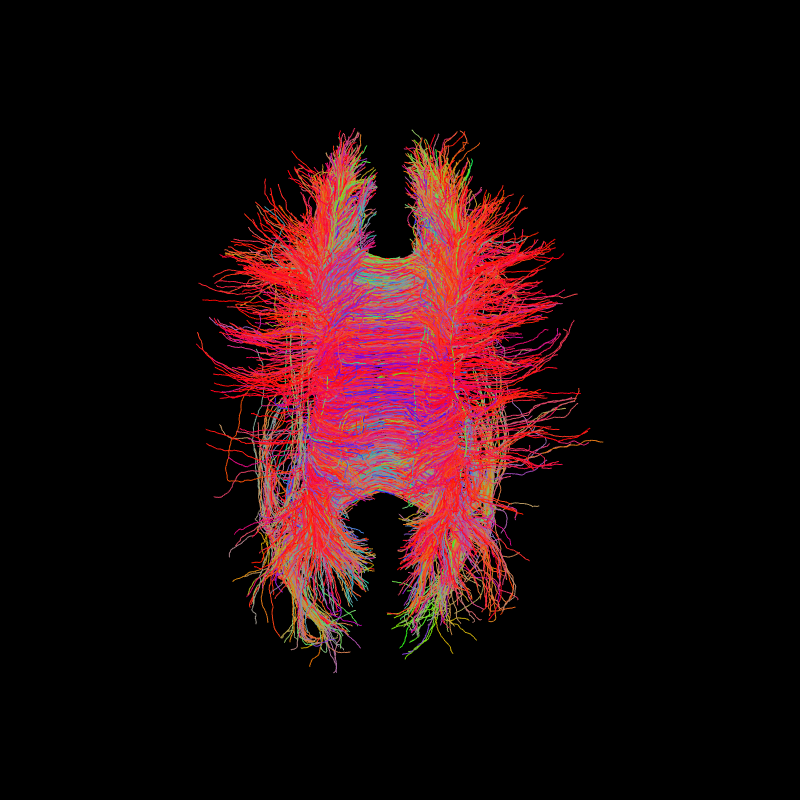
Corpus Callosum using particle filtering tractography
# Local Probabilistic Tractography
prob_streamline_generator = LocalTracking(dg,
cmc_criterion,
seeds,
affine,
max_cross=1,
step_size=step_size,
maxlen=1000,
return_all=False)
streamlines = Streamlines(prob_streamline_generator)
sft = StatefulTractogram(streamlines, hardi_img, Space.RASMM)
save_trk(sft, "tractogram_probabilistic_cmc.trk")
if has_fury:
scene = window.Scene()
scene.add(actor.line(streamlines, colormap.line_colors(streamlines)))
window.record(scene, out_path='tractogram_probabilistic_cmc.png',
size=(800, 800))
if interactive:
window.show(scene)
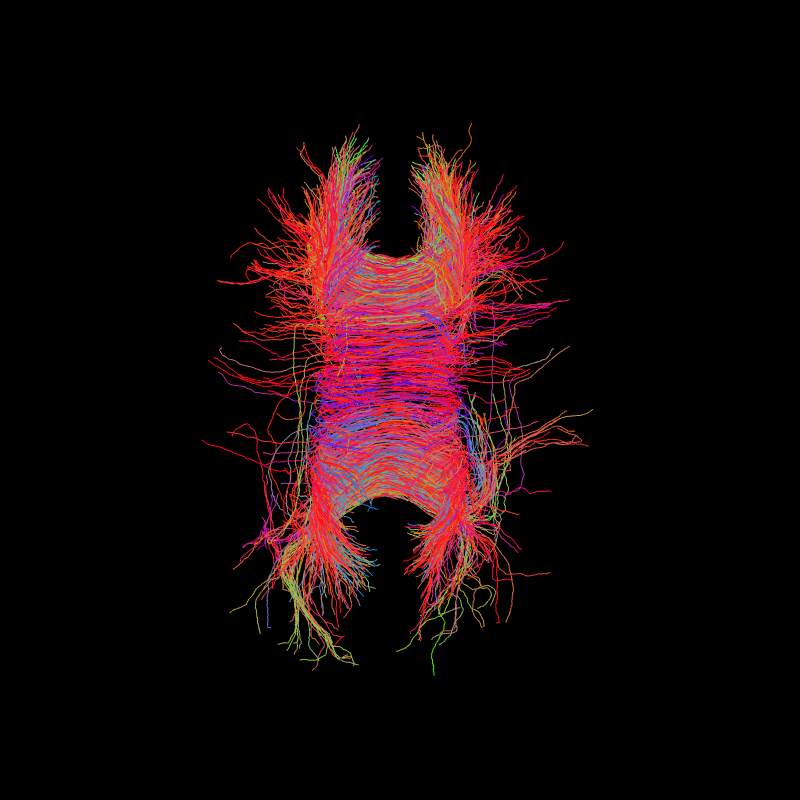
Corpus Callosum using probabilistic tractography
References#
Girard, G., Whittingstall, K., Deriche, R., & Descoteaux, M. Towards quantitative connectivity analysis: reducing tractography biases. NeuroImage, 98, 266-278, 2014.
Smith, R. E., Tournier, J.-D., Calamante, F., & Connelly, A. Anatomically-constrained tractography: Improved diffusion MRI streamlines tractography through effective use of anatomical information. NeuroImage, 63(3), 1924-1938, 2012.
Total running time of the script: (2 minutes 51.616 seconds)